Glitched Writer

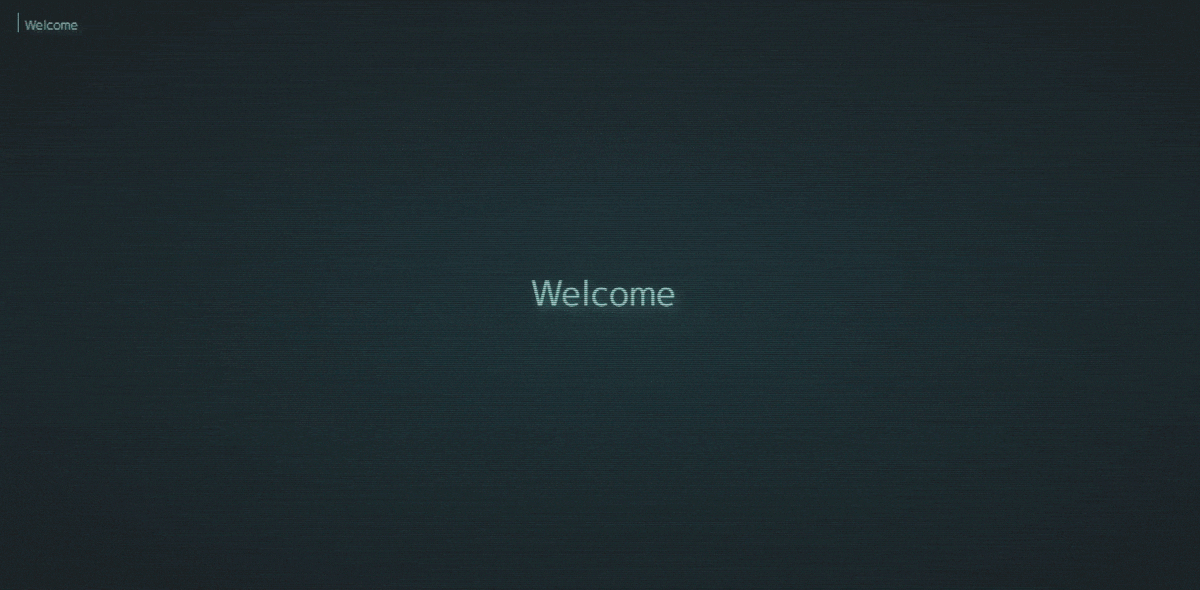
What is Glitched Writer:
A lightweight, glitched, text writing module. Highly customizable settings. Decoding, decrypting, scrambling, and simply spelling out text.
Features:
-
Manages text animation of HTML Element. Write, pause, play, add, remove and write some more!
-
Highly customizable behavior. Set of options let you animate the text the way is suits your design.
-
Callback functions firing on finish and every step.
-
Custom Event gw-finished will be dispatched on the HTML Element.
-
For styling purposes writer attatches gw-writing class to the HTML Element and data-gw-string attribute with current string.
-
Handles html tags & html entities (e.g. <br/>, <a href="#">link</a>, ;, &).
-
Can letterize string it into many span elements inside the parent element.
-
Written fully in Typescript.
Table Of Contents
- Installation
- Usage
- Presets
- Options
Installation
Download and install with npm.
npm i glitched-writer
import GlitchedWriter from 'glitched-writer'
Or use Skypack to import without need to install the package.
import GlitchedWriter from 'https://cdn.skypack.dev/glitched-writer'
CDN
You can also attach script tag with src pointing to CDN, like JsDelivr.
<script src="https://cdn.jsdelivr.net/npm/glitched-writer/lib/index.min.js"></script>
In result, the GlitchedWriter object will be available in your code, this object contains all named exports, listed here.
const writer = GlitchedWriter.create(Element, options, stepCB, finishCB)
Usage:
Creating Class Instance
Creating writer class instance:
const Writer = new GlitchedWriter(
htmlElement,
options,
onStepCallback,
onFinishCallback,
)
const Writer = new GlitchedWriter(htmlElement, {
interval: [10, 70],
oneAtATime: true,
letterize: true,
})
const Writer = new GlitchedWriter(htmlElement, {}, (string, writerData) => {
console.log(`Current string: ${string}`)
console.log('All the class data:', writerData)
})
Writing
Writing stuff and waiting with async / await.
import { wait } from 'glitched-writer'
const res = await Writer.write('Welcome')
console.log(`Finished writing: ${res.string}`)
console.log('All the writer data:', res)
await wait(1200)
await Writer.write('...to Glitch City!')
Pausing & Playing
Writer.write('Some very cool header.').then(({ status, message }) => {
console.log(`${status}: ${message}`)
})
setTimeout(() => {
Writer.pause()
}, 1000)
setTimeout(async () => {
await Writer.play()
console.log(Writer.string)
}, 2000)
One-Time-Use
For quick one-time writing.
import { glitchWrite } from 'glitched-writer'
glitchWrite('Write this and DISAPER!', htmlElement, options, ...)
On Text Input
Don't be afraid to call write method on top of each oder.
New will stop the ongoing.
inputEl.addEventListener('input', () => {
Writer.write(inputEl.value)
})
Listening For Events
textHtmlElement.addEventListener('gw-finished', e =>
console.log('finished writing:', e.detail.string),
)
Add & Remove
.add(string) & .remove(number) are methods usefull for quick, slight changes to the displayed text.
Writer.add('!!!')
Writer.remove(9)
Writing HTML
(! Potentially dangerous !) Let's you write text with html tags in it.
const Writer = new GlitchedWriter(htmlElement, { html: true })
Writer.write('<b>Be sure to click <a href="...">this!</a></b>')
Letterize
Splits written text into series of span elements. Then writing letters seperately into these child-elements. Now can be used fully with HTML option!
const Writer = new GlitchedWriter(htmlElement, { letterize: true })
Writer.write('Hello there!')
Endless animation
New option "endless" let's you run the text animation until you disable that function.
This opens the door for some additional effects, like: Show on hover (e.g. on secret fields) or refreshing text to give it user attention.
Here is a live example.
Writer.options.endless = true
Writer.write('PASSWORD')
passEl.addEventListener('mouseover', () => {
Writer.options.endless = false
})
Writer.options.endless = true
Writer.write('LOOK AT ME')
await wait(1500)
Writer.options.endless = false
Changing options post initialization
Options can be changed in 3 ways after initial Writer instance creation.
Writer.options.endless = true
Writer.extendOptions({
endless: true,
maxGhosts: 10,
})
Writer.setOptions({
endless: true,
maxGhosts: 10,
})
Available imports
List of all things that can be imported from glitched-writer module.
import GlitchedWriter, {
ConstructorOptions,
Callback,
WriterDataResponse,
glitchWrite,
presets,
glyphs,
wait,
create,
} from 'glitched-writer'
Presets
To use one of the available presets, You can simply write it's name when creating writer, in the place of options.
Available presets as for now:
-
default - Loaded automatically, featured on the GIF up top.
-
nier - Imitating the way text was appearing in the NieR: Automata's UI.
-
typewriter - One letter at a time, only slightly glitched.
-
terminal - Imitating being typed by a machine or a computer.
-
zalgo - Inspired by the "zalgo" or "cursed text", Ghost characters mostly includes the unicode combining characters, which makes the text glitch vertically.
-
neo - Recreated: Justin Windle's "Text Scramble Effect"
-
encrypted - Simple Text Scramble effect, suits well displaying secret data, like passwords or card numbers.
new GlitchedWriter(htmlElement, 'terminal')
Importing preset objects
You can import the option object of mentioned presets and tweak them, as well as some glyph sets.
import { presets, glyphs } from 'glitched-writer'
new GlitchedWriter(htmlElement, {
...presets.typewriter,
letterize: true,
})
Customizing behavior
Types and defaults:
{
steps?: [number, number] | number,
interval?: [number, number] | number,
initialDelay?: [number, number] | number,
changeChance?: number,
ghostChance?: number,
maxGhosts?: number,
glyphs?: string | string[] | Set<string>,
glyphsFromString?: boolean,
startFrom?: 'matching' | 'previous' | 'erase',
oneAtATime?: boolean | number,
html?: boolean,
letterize?: boolean,
fillSpace?: boolean,
endless?: boolean
}
Options Description
Range values will result in random values for each step for every letter.
Ghost are "glitched letters" that gets rendered randomly in the time of writing, but aren't part of final string.
-
steps - Number of minimum steps it takes one letter to reach it's goal one. Set to 0 if you want them to change to right letter in one step. (int)
-
interval - Interval between each step, for every letter. (int: ms)
-
initialDelay - first delay each letter must wait before it starts working (int: ms)
-
changeChance - Percentage chance for letter to change to glitched one (from glyphs) (p: 0-1)
-
ghostChance - Percentage chance for ghost letter to appear (p: 0-1)
-
maxGhosts - Maximal number of ghosts to occur
- int - (eg. 15) -> this will be the limit.
- float - (eg. 0.25) -> Limit = maxGhosts * goalString.length
-
glyphs - A set of characters that can appear as ghosts or letters can change into them
-
glyphsFromString - If you want to add letters from written string to the glyph charset
-
startFrom - Decides on witch algorithm to use.
- 'matching' - Will scan starting and goal string for matching characters and will try to build character map from that.
- 'previous' - Wont do any matching, just converts starting string into character map.
- 'erase' - First Erases entire string and then writes from blank space.
-
oneAtATime - Without this option enabled, letters in your string will animate all at once. Enabling this option, by setting it to true or any intiger larger than 0, will cause the string to be written from left to right (startFrom: 'erase', will make it go form right to left - when erasing). Number value, signifies number of letters being typed at one time.
-
html - Potentially dangerous option. If true, written string will be injected as html, not text content. It provides advanced text formating with html tags and more. But be sure to NOT enable it on user-provided content.
-
letterize - Instead of injecting written text to "textContent" or "innerHTML", it appends every letter of that text as a child span element. Then changing textContent of that span to current letter. It gives a lot of styling possibilities, as you can style ghosts, letters, and whole chars seperately, depending on current writer and char state.
-
fillSpace - With this enabled if letter gets erased ny replacing with space - to keep the same "width" of previous string, and to make letters "disappear in space". If disabled, every letter will "stick" to the rest. To make it more clear (hopefully), here is an example "frame" of writing: "Something farely long" -> "Short String".
- false - "XOSh8rt S3rinFv"
- true - " X OSh8rt S3rinF v "
-
endless - It will make the animation endless. But why? Well, you can disable this option while the animation is running (writer.options.endless = false) and finish the animation when you want.