Golden Path
Golden Path is a functional (immutable) query tiny parser that can give you the chance to do your object/array queries and updates on the fly.
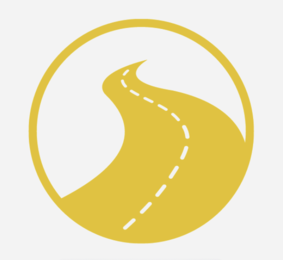
This module has the following functions:
Note: Mutating objects might break the get
so the objects that the Golden Path deals with should be updated by the update
function only. This happens because we use cache for optimizations stuff. It's recommended to call clearPathResolverCache
in order to clear it at some point (This is more relevant when using SSR).
Examples!
import { get, update, v } from 'golden-path';
const object = { name: 'islam' };
get('name', object);
const object = { peoples: [{ id: 1 }] };
get(`peoples.0.id`, object);
const object = { peoples: [{ id: 1 }, { id: 1 }] };
get(`peoples[id=1]`, object);
const object = { peoples: [{ name: 'John' }, { name: 'Alex' }] };
get(`peoples[name="Alex"]`, object);
const nameFromServer = '[]&4%45.';
const object = { peoples: [{ name: nameFromServer }, { name: 'x#DCGEDS' }] };
get(`peoples[name="${v(nameFromServer)}"]`, object);
const object = { peoples: [{ id: 1 }, { id: 1 }] };
get(`peoples*[id=1]`, object);
const object = { peoples: [{ id: 1, age: 20 }, { id: 1, age: 30 }] };
get(`peoples*[id=1][age>=20]`, object);
const object = { peoples: [{ id: 1, age: 20, kind: 'human' }, { id: 1, age: 30, kind: 'robot' }] };
get(`peoples*[id=1][age>=20].kind`, object);
const object = { peoples: [{ id: 1, age: 20 }, { id: 1, age: 30 }] };
update(`peoples*[id=1][age>=20]`, (x) => ({...x, updated: true }), object);
const englishUpdaterPipeline = _.flow([
get(`[lang="en"]`),
update('isEnglish', true)
]);
englishUpdaterPipeline([{ lang: 'en' }, { lang: 'ar' }]);
Install
npm i golden-path
Supports:
- First match queries and updates.
- Greedy (All matches) queries and updates.
- Queries in all levels.
- Multiple queries on the same array items.
- Sanitization for server dynamic values (in order to not break the parser by any symbols).
- Comparator operators: = > < >= <= !=