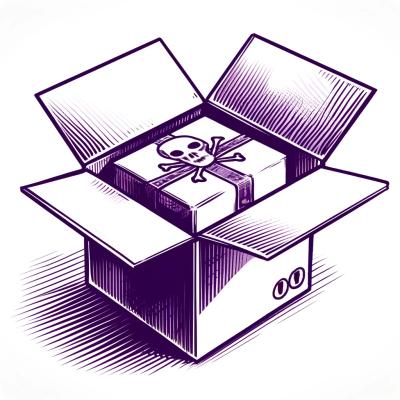
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
graphql-react
Advanced tools
A lightweight GraphQL client for React; the first Relay and Apollo alternative with server side rendering.
gql
..mjs
.module
entry for tree shaking bundlers.id
fields.__typename
insertion.Install with npm:
npm install graphql-react
Create and provide a GraphQL client:
import { GraphQL, Provider } from 'graphql-react'
const graphql = new GraphQL()
const Page = () => (
<Provider value={graphql}>Use Consumer or Query components…</Provider>
)
See the example Next.js app and GraphQL API.
Consider polyfilling:
A lightweight GraphQL client that caches requests.
Parameters
options
Object Options. (optional, default {}
)
options.cache
Object Cache to import; usually from a server side render. (optional, default {}
)Examples
import { GraphQL } from 'graphql-react'
const graphql = new GraphQL()
GraphQL request cache map, keyed by fetch options hashes.
Type: Object<string, RequestCache>
Examples
Export cache as JSON.
const exportedCache = JSON.stringify(graphql.cache)
Queries a GraphQL server.
Parameters
options
Object Options.
options.operation
Operation GraphQL operation object.options.fetchOptionsOverride
FetchOptionsOverride? Overrides default GraphQL request fetch options.options.resetOnLoad
boolean Should the GraphQL cache reset when the query loads. (optional, default false
)Returns ActiveQuery Loading query details.
Resets the GraphQL cache. Useful when a user logs out.
Parameters
exceptFetchOptionsHash
string? A fetch options hash to exempt a request from cache deletion. Useful for resetting cache after a mutation, preserving the mutation cache.Examples
graphql.reset()
A React component provides a GraphQL instance in context for nested Consumer components to use.
Parameters
Examples
import { GraphQL, Provider } from 'graphql-react'
const graphql = new GraphQL()
const Page = () => (
<Provider value={graphql}>Use Consumer or Query components…</Provider>
)
Returns ReactElement React virtual DOM element.
A React component that gets the GraphQL instance from context.
Parameters
children
ConsumerRender Render function that receives a GraphQL instance.Examples
A button component that resets the GraphQL cache.
import { Consumer } from 'graphql-react'
const ResetCacheButton = () => (
<Consumer>
{graphql => <button onClick={graphql.reset}>Reset cache</button>}
</Consumer>
)
Returns ReactElement React virtual DOM element.
Renders a GraphQL consumer.
Type: Function
Parameters
Examples
A button that resets the GraphQL cache.
graphql => <button onClick={graphql.reset}>Reset cache</button>
Returns ReactElement React virtual DOM element.
A React component to manage a GraphQL query or mutation.
Parameters
props
Object Component props.
props.variables
Object? GraphQL query variables.props.query
string GraphQL query.props.fetchOptionsOverride
FetchOptionsOverride? Overrides default GraphQL request fetch options.props.loadOnMount
boolean Should the query load when the component mounts. (optional, default false
)props.loadOnReset
boolean Should the query load when the GraphQL cache is reset. (optional, default false
)props.resetOnLoad
boolean Should the GraphQL cache reset when the query loads. (optional, default false
)props.children
QueryRender Renders the query status.Examples
A query to display a user profile.
import { Query } from 'graphql-react'
const Profile = ({ userId }) => (
<Query
loadOnMount
loadOnReset
fetchOptionsOverride={options => {
options.url = 'https://api.example.com/graphql'
}}
variables={{ userId }}
query={`
query user($userId: ID!) {
user(userId: $id) {
name
}
}
`}
>
{({ load, loading, httpError, parseError, graphQLErrors, data }) => (
<article>
<button onClick={load}>Reload</button>
{loading && <span>Loading…</span>}
{(httpError || parseError || graphQLErrors) && <strong>Error!</strong>}
{data && <h1>{data.user.name}</h1>}
</article>
)}
</Query>
)
A mutation to clap an article.
import { Query } from 'graphql-react'
const ClapArticleButton = ({ articleId }) => (
<Query
resetOnLoad
fetchOptionsOverride={options => {
options.url = 'https://api.example.com/graphql'
}}
variables={{ articleId }}
query={`
mutation clapArticle($articleId: ID!) {
clapArticle(articleId: $id) {
clapCount
}
}
`}
>
{({ load, loading, httpError, parseError, graphQLErrors, data }) => (
<aside>
<button onClick={load} disabled={loading}>
Clap
</button>
{(httpError || parseError || graphQLErrors) && <strong>Error!</strong>}
{data && <p>Clapped {data.clapArticle.clapCount} times.</p>}
</aside>
)}
</Query>
)
Returns ReactElement React virtual DOM element.
Renders the status of a query or mutation.
Type: Function
Parameters
load
Function Loads the query on demand, updating cache.loading
boolean Is the query loading.httpError
HTTPError? Fetch HTTP error.parseError
string? Parse error message.graphQLErrors
Object? GraphQL response errors.data
Object? GraphQL response data.Examples
;({ load, loading, httpError, parseError, graphQLErrors, data }) => (
<aside>
<button onClick={load}>Reload</button>
{loading && <span>Loading…</span>}
{(httpError || parseError || graphQLErrors) && <strong>Error!</strong>}
{data && <h1>{data.user.name}</h1>}
</aside>
)
Returns ReactElement React virtual DOM element.
Recursively preloads Query components that have the loadOnMount
prop in a React element tree. Useful for server side rendering (SSR) or to preload components for a better user experience when they mount.
Parameters
element
ReactElement A React virtual DOM element.Examples
An async SSR function that returns a HTML string and cache JSON for client hydration.
import { GraphQL, preload, Provider } from 'graphql-react'
import { renderToString } from 'react-dom/server'
import { App } from './components'
const graphql = new GraphQL()
const page = (
<Provider value={graphql}>
<App />
</Provider>
)
export async function ssr() {
await preload(page)
return {
cache: JSON.stringify(graphql.cache),
html: renderToString(page)
}
}
Returns Promise Resolves once loading is done and cache is ready to be exported from the GraphQL instance. Cache can be imported when constructing new GraphQL instances.
Loading query details.
Type: Object
Properties
fetchOptionsHash
string fetch options hash.cache
RequestCache? Results from the last identical request.request
RequestCachePromise A promise that resolves fresh request cache.Fetch options for a GraphQL request. See polyfillable fetch options.
Type: Object
Properties
url
string A GraphQL API URL.body
(string | FormData) HTTP request body.headers
Object HTTP request headers.credentials
string? Authentication credentials mode.Overrides default GraphQL request fetch options. Modify the provided options object without a return.
Type: Function
Parameters
fetchOptions
FetchOptions Default GraphQL request fetch options.operation
Operation? A GraphQL operation object.Examples
options => {
options.url = 'https://api.example.com/graphql'
options.credentials = 'include'
}
Fetch HTTP error.
Type: Object
Properties
A GraphQL operation object. Additional properties may be used; all are sent to the GraphQL server.
Type: Object
Properties
JSON serializable result of a GraphQL request (including all errors and data) suitable for caching.
Type: Object
Properties
httpError
HTTPError? Fetch HTTP error.parseError
string? Parse error message.graphQLErrors
Object? GraphQL response errors.data
Object? GraphQL response data.A promise for a loading query that resolves the request cache.
Type: Promise<RequestCache>
FAQs
A GraphQL client for React using modern context and hooks APIs that’s lightweight (< 4 kB) but powerful; the first Relay and Apollo alternative with server side rendering.
The npm package graphql-react receives a total of 1,669 weekly downloads. As such, graphql-react popularity was classified as popular.
We found that graphql-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.