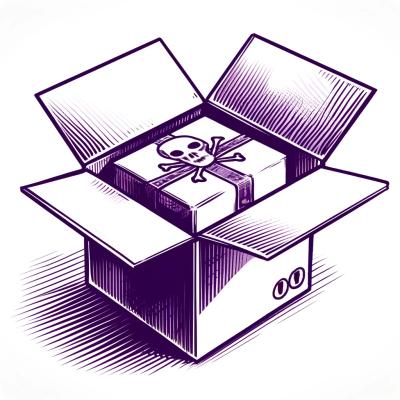
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
graphql-react
Advanced tools
A lightweight GraphQL client for React; the first Relay and Apollo alternative with server side rendering.
gql
..mjs
.module
entry for tree shaking bundlers.id
fields.__typename
insertion.To install graphql-react
from npm run:
npm install graphql-react
Create and provide a single GraphQL
client to hold the cache for all the queries in your app:
import { GraphQL, Provider } from 'graphql-react'
const graphql = new GraphQL()
export const App = ({ children }) => (
<Provider value={graphql}>{children}</Provider>
)
GraphQL
accepts a single cache
option for hydration after SSR; see Example.
Setup is simple because Query
components determine their own fetch options (such as the GraphQL endpoint URI). Multiple GraphQL APIs can be used in an app 🤯
Use the Query
component for queries and mutations throughout your app:
import { Query } from 'graphql-react'
export const PokemonViewer = ({ name }) => (
<Query
loadOnMount
loadOnReset
fetchOptionsOverride={options => {
options.url = 'https://graphql-pokemon.now.sh'
}}
variables={{ name }}
query={
/* GraphQL */ `
query pokemon($name: String!) {
pokemon(name: $name) {
number
image
}
}
`
}
>
{({ loading, data }) =>
data ? (
<figure>
<img src={data.image} alt={name} />
<figcaption>
Pokémon #{data.number}: {name}
</figcaption>
</figure>
) : loading ? (
<p>Loading…</p>
) : (
<p>Error!</p>
)
}
</Query>
)
To make queries and mutations without a component, use the GraphQL
instance method query
.
See the example GraphQL API and Next.js web app, deployed at graphql-react.now.sh.
Consider polyfilling:
A lightweight GraphQL client that caches requests.
Parameter | Type | Description |
---|---|---|
options | Object? = {} | Options. |
options.cache | Object? = {} | Cache to import; usually from a server side render. |
Constructing a new GraphQL client.
import { GraphQL } from 'graphql-react'
const graphql = new GraphQL()
Queries a GraphQL server.
Parameter | Type | Description |
---|---|---|
options | Object | Options. |
options.operation | Operation | GraphQL operation object. |
options.fetchOptionsOverride | FetchOptionsOverride? | Overrides default GraphQL request fetch options. |
options.resetOnLoad | boolean? = false | Should the GraphQL cache reset when the query loads. |
Returns: ActiveQuery — Loading query details.
Resets the GraphQL cache. Useful when a user logs out.
Parameter | Type | Description |
---|---|---|
exceptFetchOptionsHash | string? | A fetch options hash to exempt a request from cache deletion. Useful for resetting cache after a mutation, preserving the mutation cache. |
Resetting the GraphQL cache.
graphql.reset()
GraphQL request cache map, keyed by fetch options hashes.
Export cache as JSON.
const exportedCache = JSON.stringify(graphql.cache)
A React component that gets the GraphQL
instance from context.
Parameter | Type | Description |
---|---|---|
children | ConsumerRender | Render function that receives a GraphQL instance. |
Returns: ReactElement — React virtual DOM element.
A button component that resets the GraphQL cache.
import { Consumer } from 'graphql-react'
const ResetCacheButton = () => (
<Consumer>
{graphql => <button onClick={graphql.reset}>Reset cache</button>}
</Consumer>
)
Recursively preloads Query
components that have the loadOnMount
prop in a React element tree. Useful for server side rendering (SSR) or to preload components for a better user experience when they mount.
Parameter | Type | Description |
---|---|---|
element | ReactElement | A React virtual DOM element. |
Returns: Promise — Resolves once loading is done and cache is ready to be exported from the GraphQL
instance. Cache can be imported when constructing new GraphQL
instances.
An async SSR function that returns a HTML string and cache JSON for client hydration.
import { GraphQL, preload, Provider } from 'graphql-react'
import { renderToString } from 'react-dom/server'
import { App } from './components'
const graphql = new GraphQL()
const page = (
<Provider value={graphql}>
<App />
</Provider>
)
export async function ssr() {
await preload(page)
return {
cache: JSON.stringify(graphql.cache),
html: renderToString(page)
}
}
A React component that provides a GraphQL
instance in context for nested Consumer
components to use.
Parameter | Type | Description |
---|---|---|
value | GraphQL | A GraphQL instance. |
children | ReactNode | A React node. |
Returns: ReactElement — React virtual DOM element.
Using the Provider
component for a page.
import { GraphQL, Provider } from 'graphql-react'
const graphql = new GraphQL()
const Page = () => (
<Provider value={graphql}>Use Consumer or Query components…</Provider>
)
A React component to manage a GraphQL query or mutation.
Parameter | Type | Description |
---|---|---|
props | Object | Component props. |
props.variables | Object? | GraphQL query variables. |
props.query | string | GraphQL query. |
props.fetchOptionsOverride | FetchOptionsOverride? | Overrides default GraphQL request fetch options. |
props.loadOnMount | boolean? = false | Should the query load when the component mounts. |
props.loadOnReset | boolean? = false | Should the query load when the GraphQL cache is reset. |
props.resetOnLoad | boolean? = false | Should the GraphQL cache reset when the query loads. |
props.children | QueryRender | Renders the query status. |
Returns: ReactElement — React virtual DOM element.
A query to display a user profile.
import { Query } from 'graphql-react'
const Profile = ({ userId }) => (
<Query
loadOnMount
loadOnReset
fetchOptionsOverride={options => {
options.url = 'https://api.example.com/graphql'
}}
variables={{ userId }}
query={`
query user($userId: ID!) {
user(userId: $userId) {
name
}
}
`}
>
{({
load,
loading,
fetchError,
httpError,
parseError,
graphQLErrors,
data
}) => (
<article>
<button onClick={load}>Reload</button>
{loading && <span>Loading…</span>}
{(fetchError || httpError || parseError || graphQLErrors) && (
<strong>Error!</strong>
)}
{data && <h1>{data.user.name}</h1>}
</article>
)}
</Query>
)
A mutation to clap an article.
import { Query } from 'graphql-react'
const ClapArticleButton = ({ articleId }) => (
<Query
resetOnLoad
fetchOptionsOverride={options => {
options.url = 'https://api.example.com/graphql'
}}
variables={{ articleId }}
query={`
mutation clapArticle($articleId: ID!) {
clapArticle(articleId: $articleId) {
clapCount
}
}
`}
>
{({
load,
loading,
fetchError,
httpError,
parseError,
graphQLErrors,
data
}) => (
<aside>
<button onClick={load} disabled={loading}>
Clap
</button>
{(fetchError || httpError || parseError || graphQLErrors) && (
<strong>Error!</strong>
)}
{data && <p>Clapped {data.clapArticle.clapCount} times.</p>}
</aside>
)}
</Query>
)
Loading query details.
Type: Object
Property | Type | Description |
---|---|---|
fetchOptionsHash | string | fetch options hash. |
cache | RequestCache? | Results from the last identical request. |
request | Promise<RequestCache> | A promise that resolves fresh request cache. |
Renders a GraphQL
consumer.
Type: function
Parameter | Type | Description |
---|---|---|
graphql | GraphQL | GraphQL instance. |
Returns: ReactElement — React virtual DOM element.
A button that resets the GraphQL cache.
graphql => <button onClick={graphql.reset}>Reset cache</button>
Polyfillable fetch options for a GraphQL request.
Type: Object
Property | Type | Description |
---|---|---|
url | string | A GraphQL API URL. |
body | string | FormData | HTTP request body. |
headers | Object | HTTP request headers. |
credentials | string? | Authentication credentials mode. |
Overrides default GraphQL request fetch options. Modify the provided options object without a return.
Type: function
Parameter | Type | Description |
---|---|---|
fetchOptions | FetchOptions | Default GraphQL request fetch options. |
operation | Operation? | A GraphQL operation object. |
Setting fetch options for an example API.
options => {
options.url = 'https://api.example.com/graphql'
options.credentials = 'include'
}
Fetch HTTP error.
Type: Object
Property | Type | Description |
---|---|---|
status | number | HTTP status code. |
statusText | string | HTTP status text. |
A GraphQL operation object. Additional properties may be used; all are sent to the GraphQL server.
Type: Object
Property | Type | Description |
---|---|---|
query | string | GraphQL queries or mutations. |
variables | Object | Variables used by the query. |
Renders the status of a query or mutation.
Type: function
Parameter | Type | Description |
---|---|---|
load | function | Loads the query on demand, updating cache. |
loading | boolean | Is the query loading. |
fetchError | string? | Fetch error message. |
httpError | HttpError? | Fetch response HTTP error. |
parseError | string? | Parse error message. |
graphQLErrors | Object? | GraphQL response errors. |
data | Object? | GraphQL response data. |
Returns: ReactElement — React virtual DOM element.
Rendering a user profile query.
;({
load,
loading,
fetchError,
httpError,
parseError,
graphQLErrors,
data
}) => (
<aside>
<button onClick={load}>Reload</button>
{loading && <span>Loading…</span>}
{(fetchError || httpError || parseError || graphQLErrors) && (
<strong>Error!</strong>
)}
{data && <h1>{data.user.name}</h1>}
</aside>
)
JSON serializable result of a GraphQL request (including all errors and data) suitable for caching.
Type: Object
Property | Type | Description |
---|---|---|
fetchError | string? | Fetch error message. |
httpError | HttpError? | Fetch response HTTP error. |
parseError | string? | Parse error message. |
graphQLErrors | Object? | GraphQL response errors. |
data | Object? | GraphQL response data. |
2.0.1
GraphQLQuery
component from API documentation as it used internally and is not exported.jsdoc-md
version.FAQs
A GraphQL client for React using modern context and hooks APIs that’s lightweight (< 4 kB) but powerful; the first Relay and Apollo alternative with server side rendering.
The npm package graphql-react receives a total of 1,669 weekly downloads. As such, graphql-react popularity was classified as popular.
We found that graphql-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.