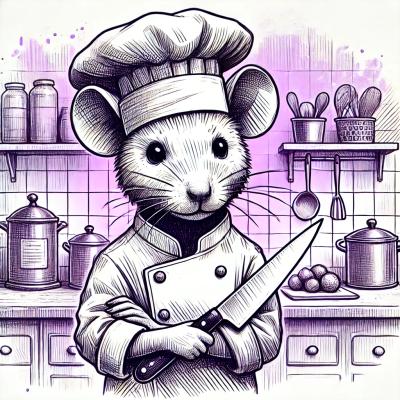
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
graphql-yoga
Advanced tools
[](https://travis-ci.org/graphcool/graphql-yoga) [](https://badge.fury.io/js/graphql-yoga) [;
const typeDefs = `
type Query {
hello: String!
}
`;
const resolvers = {
Query: {
hello: () => 'Hello, world!',
},
};
const server = createServer({ typeDefs, resolvers });
server.start(() => console.log('Server is running on http://localhost:4000'));
Subscriptions
This code demonstrates how to set up a GraphQL server with subscriptions. It uses the 'graphql-subscriptions' package to handle real-time updates. A new message is published every second.
const { createServer } = require('graphql-yoga');
const { PubSub } = require('graphql-subscriptions');
const pubsub = new PubSub();
const typeDefs = `
type Query {
hello: String!
}
type Subscription {
newMessage: String!
}
`;
const resolvers = {
Query: {
hello: () => 'Hello, world!',
},
Subscription: {
newMessage: {
subscribe: () => pubsub.asyncIterator(['NEW_MESSAGE']),
},
},
};
const server = createServer({ typeDefs, resolvers });
server.start(() => console.log('Server is running on http://localhost:4000'));
setInterval(() => {
pubsub.publish('NEW_MESSAGE', { newMessage: 'Hello, world!' });
}, 1000);
File Uploads
This code sets up a GraphQL server that supports file uploads. It defines a custom scalar 'Upload' and a mutation 'singleUpload' that handles the file upload process.
const { createServer } = require('graphql-yoga');
const typeDefs = `
scalar Upload
type Query {
hello: String!
}
type Mutation {
singleUpload(file: Upload!): String!
}
`;
const resolvers = {
Query: {
hello: () => 'Hello, world!',
},
Mutation: {
singleUpload: async (parent, { file }) => {
const { createReadStream, filename } = await file;
createReadStream().pipe(fs.createWriteStream(path.join(__dirname, filename)));
return filename;
},
},
};
const server = createServer({ typeDefs, resolvers });
server.start(() => console.log('Server is running on http://localhost:4000'));
Apollo Server is a community-driven, open-source GraphQL server that works with any GraphQL schema. It provides a robust set of features, including caching, subscriptions, and more. Compared to graphql-yoga, Apollo Server offers more advanced features and integrations but may require more configuration.
express-graphql is a minimalistic GraphQL HTTP server middleware for Express. It is easy to set up and use, making it a good choice for simple applications. However, it lacks some of the advanced features provided by graphql-yoga, such as built-in subscriptions and file uploads.
graphql-koa is a GraphQL server middleware for Koa. It provides a simple way to integrate GraphQL into a Koa application. While it is similar to express-graphql in terms of simplicity, it does not offer the same level of built-in features as graphql-yoga.
Fully-featured GraphQL Server with focus on easy setup, performance & great developer experience
graphql-yoga
is based on the following libraries & tools:
express
/apollo-server
: Performant, extensible web server frameworkapollo-upload-server
: File uploads via queries or mutationsgraphql-subscriptions
/subscriptions-transport-ws
: GraphQL subscriptions servergraphql.js
/graphql-tools
: GraphQL engine & schema helpersgraphql-playground
: Interactive GraphQL IDEyarn add graphql-yoga
import { GraphQLServer } from 'graphql-yoga'
// ... or using `require()`
// const { GraphQLServer } = require('graphql-yoga')
const typeDefs = `
type Query {
hello(name: String): String!
}
`
const resolvers = {
Query: {
hello: (_, { name }) => `Hello ${name || 'World'}`,
},
}
const server = new GraphQLServer({ typeDefs, resolvers })
server.start(() => console.log('Server is running on localhost:4000'))
To get started with
graphql-yoga
, follow the instructions in the READMEs of the examples.
new GraphQLServer()
Type Signature:
constructor(props: Props): GraphQLServer
import { GraphQLServer } from 'graphql-yoga'
const server = new GraphQLServer({
typeDefs,
resolvers,
options: {
port: 4000,
},
})
Here is a list of all argument fields:
typeDefs
A string containing GraphQL type definitions in SDL (required if schema
is not provided)
resolvers
An object containing resolvers for the fields specified in typeDefs
(required if schema
is not provided). Uses makeExecutableSchema
from graphql-tools
.
schema
An instance of GraphQLSchema
(required if typeDefs
and resolvers
are not provided)
context
An object or function containing custom data being passed through your resolver chain
The function has the following signature: ({ request?: Request, connection?: SubscriptionOptions }) => any
request
is the HTTP request object which is provided for normal HTTP requests (queries/mutations)connection
is the connection object provided for new subscriptionsNote that there is always either the
request
or theconnection
argument provided.
options
The options
object has the following fields:
cors
: An object containing configuration options for cors. Provide false
to disable. (default: undefined
).
disableSubscriptions
: A boolean indicating where subscriptions should be en- or disabled for your server (default: false
).
port
: An integer determining the port your server will be listening on (default: 4000
); note that you can also specify the port by setting the PORT
environment variable.
endpoint
: A string that defines the HTTP endpoint of your server (default: '/'
).
subscriptionsEndpoint
: A string that defines the subscriptions (websocket) endpoint for your server (default: '/'
).
playgroundEndpoint
: A string that defines the endpoint where you can invoke the Playground (default: '/'
).
disablePlayground
: A boolean indicating whether the Playground should be enabled (default: false
).
uploads
: An object containing configuration options for apollo-upload-server.
tracing
: A boolean or object ({ mode: 'enabled' | 'disabled' | 'http-header' }
) to enable or disable tracing responses (default: { mode: 'http-header' }
).
When using tracing
with { mode: 'http-header' }
you can enable tracing results on a per-request basis by setting the X-Apollo-Tracing: 1
in your client (automatically set by GraphQL Playground).
GraphQLServer.start()
Type Signature:
start(callback?: (() => void)): Promise<void>
// this starts the HTTP and WebSocket server
server.start()
// you can also provide a callback (e.g. for logging)
server.start(() => console.log('Server is running on localhost:4000'))
GraphQLServer.express
Type Signature:
express: express.Application
You can easily access (and modify) the underlying Express application using the exposed express
property. Here is a simple example:
server.express.use(myMiddleware())
PubSub
PubSub
can be used to implement subscriptions and has a simple publish-subscribe API:
import { PubSub } from 'graphql-yoga'
// simply instantiate a new PubSub object ...
const pubsub = new PubSub()
const SOMETHING_CHANGED_TOPIC = 'something_changed';
const resolvers = {
Subscription: {
somethingChanged: {
// ... implement the `subscribe` method in your subscription resolvers ...
subscribe: () => pubsub.asyncIterator(SOMETHING_CHANGED_TOPIC),
},
},
}
// ... and from somewhere publish events
setInterval(
() => pubsub.publish(SOMETHING_CHANGED_TOPIC, { somethingChanged: { id: "123" }}),
2000
)
For more information see the original documentation in graphql-subscriptions
.
graphql-yoga
by default exposes an HTTP and WebSocket endpoint on localhost:4000/
. (Can be adjusted in the constructor options.)
Once your graphql-yoga
server is running, you can use GraphQL Playground out of the box. (Read here for more information.)
There are three examples demonstrating how to quickly get started with graphql-yoga
:
hello
query.create-react-app
demonstrating how to query data from graphql-yoga
with Apollo Client 2.0.now
To deploy your graphql-yoga
server with now
, follow these instructions:
graphql-yoga
servernow
in your terminalup
(Coming soon 🔜 )graphql-yoga
compare to apollo-server
and other tools?As mentioned above, graphql-yoga
is built on top of a variety of other packages, such as graphql.js
, express
and apollo-server
. Each of these provide a certain piece of functionality required for building a GraphQL server.
Using these packages individually incurs overhead in the setup process and requires you to write a lot of boilerplate. graphql-yoga
abstracts away the initial complexity and required boilerplate and let's you get started quickly with a set of sensible defaults for your server configuration.
graphql-yoga
is like create-react-app
for building GraphQL servers.
express
and graphql.js
?graphql-yoga
is all about convenience and a great "Getting Started"-experience by abstracting away the complexity that comes when you're building your own GraphQL from scratch. It's a pragmatic approach to bootstrap a GraphQL server, much like create-react-app
removes friction when first starting out with React.
Whenever the defaults of graphql-yoga
are too tight of a corset for you, you can simply eject from it and use the tooling it's build upon - there's no lock-in or any other kind of magic going on preventing you from this.
express
setup?The core value of graphql-yoga
is that you don't have to write the boilerplate required to configure your express.js application. However, once you need to add more customized behaviour to your server, the default configuration provided by graphql-yoga
might not suit your use case any more. For example, it might be the case that you want to add more custom middleware to your server, like for logging or error reporting.
For these cases, GraphQLServer
exposes the express.Application
directly via its express
property:
server.express.use(myMiddleware())
Join our Slack community if you run into issues or have questions. We love talking to you!
FAQs
Unknown package
The npm package graphql-yoga receives a total of 330,741 weekly downloads. As such, graphql-yoga popularity was classified as popular.
We found that graphql-yoga demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.