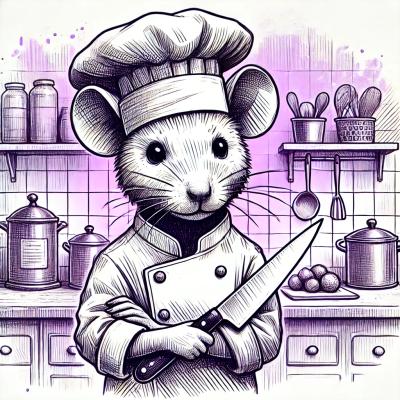
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
gridstack
Advanced tools
gridstack.js for dashboard layout and creation, with many wrappers (React, Angular, Ember, knockout...)
Gridstack is a JavaScript library that helps you create dynamic, responsive, and draggable grid layouts. It is particularly useful for building dashboards, widgets, and other interactive web applications where users can customize the layout by dragging and resizing elements.
Draggable Widgets
This feature allows you to add draggable widgets to the grid. The code sample initializes a grid and adds a widget at position (0, 0) with a width and height of 2 units.
const grid = GridStack.init();
grid.addWidget('<div><div class="grid-stack-item-content">Widget 1</div></div>', {x: 0, y: 0, width: 2, height: 2});
Resizable Widgets
This feature allows you to add resizable widgets to the grid. The code sample initializes a grid and adds a resizable widget at position (1, 1) with a width and height of 2 units.
const grid = GridStack.init();
grid.addWidget('<div><div class="grid-stack-item-content">Widget 2</div></div>', {x: 1, y: 1, width: 2, height: 2, resizable: true});
Static Grid
This feature allows you to create a static grid where widgets cannot be dragged or resized. The code sample initializes a static grid and adds a widget at position (2, 2) with a width and height of 2 units.
const grid = GridStack.init({staticGrid: true});
grid.addWidget('<div><div class="grid-stack-item-content">Static Widget</div></div>', {x: 2, y: 2, width: 2, height: 2});
Saving and Loading Layouts
This feature allows you to save the current layout of the grid and load it later. The code sample demonstrates how to save the layout of a grid and then load it into another grid instance.
const grid = GridStack.init();
const layout = grid.save();
// Later, you can load the layout
const grid2 = GridStack.init();
grid2.load(layout);
Muuri is a JavaScript library for creating responsive, sortable, filterable, and draggable grid layouts. It offers more advanced sorting and filtering options compared to Gridstack but may require more configuration for basic grid functionalities.
Packery is a JavaScript library for creating draggable and resizable grid layouts. It focuses on packing elements in the most efficient way possible, which can be useful for creating masonry-style layouts. However, it lacks some of the built-in features for saving and loading layouts that Gridstack offers.
Interact.js is a JavaScript library for drag-and-drop, resizing, and multi-touch gestures. While it is more versatile and can be used for a variety of interactive elements, it requires more custom code to achieve the same grid functionalities that Gridstack provides out of the box.
Mobile-friendly Javascript library for dashboard layout and creation. Making a drag-and-drop, multi-column dashboard has never been easier. Allows you to build draggable, responsive bootstrap v3-friendly layouts. It also has multiple bindings and works great with React, Angular, Knockout.js, Ember and others, and comes with a Typescript definition out of the box.
Inspired by no-longer maintained gridster.js, built with love.
Please visit http://gridstackjs.com and these demos.
Join us on Slack: https://gridstackjs.troolee.com
Table of Contents generated with DocToc
Please visit http://gridstackjs.com and these demos.
$ yarn install gridstack
<link rel="stylesheet" href="gridstack.css" />
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="gridstack.all.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/gridstack@0.6.4/dist/gridstack.min.css" />
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/gridstack@0.6.4/dist/gridstack.all.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/gridstack@0.6.4/dist/gridstack.css" />
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/gridstack@0.6.4/dist/gridstack.js"></script>
<script src="https://cdn.jsdelivr.net/npm/gridstack@0.6.4/dist/jquery-ui.js"></script>
<script src="https://cdn.jsdelivr.net/npm/gridstack@0.6.4/dist/gridstack.jQueryUI.js"></script>
<div class="grid-stack">
<div class="grid-stack-item">
<div class="grid-stack-item-content">Item 1</div>
</div>
<div class="grid-stack-item" data-gs-width="2">
<div class="grid-stack-item-content">Item 2 wider</div>
</div>
</div>
<script type="text/javascript">
$(function () {
$('.grid-stack').gridstack();
});
</script>
see jsfiddle sample as running example too.
Array.prototype.find
, and Number.isNaN()
for IE and older browsers.
gridstack-poly.js
for that
(part of gridstack.all.js
) or you can look at other pollyfills
(core.js and mozilla.org).Using gridstack.js with jQuery UI
jquery-ui.js
(and min.js) which is part of gridstack.all.js
. If you wish to bring your own lib, include the individual gridstack parts instead of all.jsDocumentation can be found here.
You can easily extend or patch gridstack with code like this:
$(function () {
// extend gridstack with our own custom method
window.GridStackUI.prototype.printCount = function() {
console.log('grid has ' + this.grid.nodes.length + ' items');
};
$('.grid-stack').gridstack();
// you can now call on any grid this...
$('.grid-stack').data('gridstack').printCount();
});
Please use jQuery UI Touch Punch to make jQuery UI Draggable/Resizable working on touch-based devices.
<script src="core-js/client/shim.min.js"></script>
<script src="jquery.min.js"></script>
<script src="jquery-ui.min.js"></script>
<script src="jquery.ui.touch-punch.min.js"></script>
<script src="gridstack.js"></script>
Also alwaysShowResizeHandle
option may be useful:
$(function () {
var options = {
alwaysShowResizeHandle: /Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(navigator.userAgent)
};
$('.grid-stack').gridstack(options);
});
If you're still experiencing issues on touch devices please check #444
search for 'gridstack' under NPM for latest, more to come...
GridStack makes it very easy if you need [1-12] columns out of the box (default is 12), but you always need 2 things if you need to customize this:
column
grid option when creating a grid to your number N$('.grid-stack').gridstack( {column: N} );
gridstack-extra.css
if N < 12 (else custom CSS - see next). Without these, things will not render/work correctly.<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/gridstack@0.6.4/dist/gridstack-extra.css"/>
<div class="grid-stack grid-stack-N">...</div>
Note: we added grid-stack-N
class and gridstack-extra.css
which defines CSS for grids with custom [1-12] columns. Anything more and you'll need to generate the SASS/CSS yourself (see next).
See example: 2 grids demo with 6 columns
If you need > 12 columns or want to generate the CSS manually you will need to generate CSS rules for .grid-stack-item[data-gs-width="X"]
and .grid-stack-item[data-gs-x="X"]
.
For instance for 3-column grid you need to rewrite CSS to be:
.grid-stack-item[data-gs-width="3"] { width: 100% }
.grid-stack-item[data-gs-width="2"] { width: 66.66666667% }
.grid-stack-item[data-gs-width="1"] { width: 33.33333333% }
.grid-stack-item[data-gs-x="2"] { left: 66.66666667% }
.grid-stack-item[data-gs-x="1"] { left: 33.33333333% }
For 4-column grid it should be:
.grid-stack-item[data-gs-width="4"] { width: 100% }
.grid-stack-item[data-gs-width="3"] { width: 75% }
.grid-stack-item[data-gs-width="2"] { width: 50% }
.grid-stack-item[data-gs-width="1"] { width: 25% }
.grid-stack-item[data-gs-x="3"] { left: 75% }
.grid-stack-item[data-gs-x="2"] { left: 50% }
.grid-stack-item[data-gs-x="1"] { left: 25% }
and so on.
Better yet, here is a SASS code snippet which can make life much easier (Thanks to @ascendantofrain, #81 and @StefanM98, #868) and you can use sites like sassmeister.com to generate the CSS for you instead:
.grid-stack > .grid-stack-item {
$gridstack-columns: 12;
min-width: (100% / $gridstack-columns);
@for $i from 1 through $gridstack-columns {
&[data-gs-width='#{$i}'] { width: (100% / $gridstack-columns) * $i; }
&[data-gs-x='#{$i}'] { left: (100% / $gridstack-columns) * $i; }
&[data-gs-min-width='#{$i}'] { min-width: (100% / $gridstack-columns) * $i; }
&[data-gs-max-width='#{$i}'] { max-width: (100% / $gridstack-columns) * $i; }
}
}
you can also look at the SASS src/gridstack-extra.scss and modify to add more columns
and also have the .grid-stack-N
prefix to support letting the user change columns dynamically.
You can override default resizable
/draggable
options. For instance to enable other then bottom right resizing handle
you can init gridstack like:
$('.grid-stack').gridstack({
resizable: {
handles: 'e, se, s, sw, w'
}
});
Note: It's not recommended to enable nw
, n
, ne
resizing handles. Their behaviour may be unexpected.
As of v0.3.0, gridstack introduces a new plugin system. The drag'n'drop functionality has been modified to take advantage of this system. Because of this, and to avoid dependency on core code from jQuery UI, the plugin functionality was moved to a separate file.
To ensure gridstack continues to work, either include the additional gridstack.jQueryUI.js
file into your HTML or use gridstack.all.js
:
<script src="gridstack.js"></script>
<script src="gridstack.jQueryUI.js"></script>
or
<script src="gridstack.all.js"></script>
We're working on implementing support for other drag'n'drop libraries through the new plugin system.
View our change log here.
gridstack.js is currently maintained by Dylan Weiss and Alain Dumesny, originally created by Pavel Reznikov. We appreciate all contributors for help.
FAQs
TypeScript/JS lib for dashboard layout and creation, responsive, mobile support, no external dependencies, with many wrappers (React, Angular, Vue, Ember, knockout...)
The npm package gridstack receives a total of 176,757 weekly downloads. As such, gridstack popularity was classified as popular.
We found that gridstack demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.