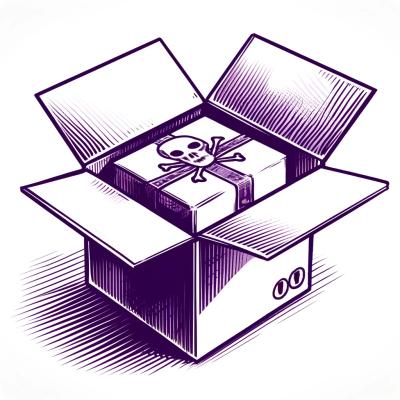
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
gw-docxtemplater-modules
Advanced tools
This module exposes a tag to include html. The HTML is converted to Native Open XML by the module. This allows you to add a formatted field entered by the user, or add more complex data by writing simple HTML (Open XML is much more complex to work with then HTML).
The HTML module currently supports:
<br>
tags<p>
tags<h1-6>
tags, <h1>
translates to Title
, <h2>
translates to Header1
, <h3>
translates to Header2
, because there is no concept of title in the body of HTML<p>
tags<b>
tags<i>
tags<u>
tags<ul>
tags<ol>
tags<li>
tags<span>
tags<small>
tags<s>
tags<strong>
tags<em>
tags<code>
tags<table>
, <tr>
, <td>
, <tbody>
, <thead>
, <tfoot>
, <th>
tags<a href="URL">Linktext</a>
tagsstyle="color: #bbbbbb"
propertystyle="font-size: 30px"
property<input type="checkbox">
and <input type="checkbox" checked>
style="background-color: blue"
property (for black, blue, cyan, darkBlue, darkGray, darkRed, green, lightGray, magenta, red, white, yellow). This limitation is of microsoft word itself.style="padding-left: 30px"
<sub>
and <sup>
<img>
only if including the imageModule too, by using base64 srcImportant : This module only supports docx, not pptx, for multiple reasons
You will need docxtemplater v3: npm install docxtemplater
Install this module with npm install --save "$url"
Your docx should contain the text: {~html}
. You can find a working sample at ./sample.js
{~html}
is used for inline HTML{~~html}
is used for block HTMLTo be clear :
{~inline}
tag is used when you want to replace part of a paragraph. For example you can write :My product is {~blueText} and costs ...
The tag is inline, there is some other text in the paragraph. In this case, you can only use inline HTML elements (<span>
, <b>
, <i>
, <u>
)
{~~block}
tag is used when you want to replace a whole paragraph, and you want to insert multiple elements{~~block}
The tag is block, there is no other text in the paragraph. In this case, you can only use block HTML elements (<p>
, <ul>
, <table>
, <ol>
, <h1>
)
Also, in tr
elements, we can have lists, ..., so you have to surround your tags with <p>
.
This code will throw an error (Tag 'em' is of type inline, it is not supported as the root of a block-scoped tag) :
<td style="width:33.333333333333336%;">
foobar<em>italics</em>
</td>
And this code will work :
<td style="width:33.333333333333336%;">
<p>foobar<em>italics</em></p>
</td>
It is possible to set options to the htmlModule.
Option descriptions :
<span>
For example, this will remap the styles so that h1 maps to Heading1 (instead of the default Title)
function styleTransformer (tags, docStyles) {
tags.h1 = docStyles.Heading1;
tags.h2 = docStyles.Heading2;
tags.h3 = docStyles.Heading3;
tags.h4 = docStyles.Heading4;
tags.h5 = docStyles.Heading5;
return tags;
};
const module = new HTMLModule({
styleTransformer: styleTransformer,
});
For example to ignore all unknown tags:
const module = new HTMLModule({
ignoreUnknownTags: true,
});
To change the size of "padding-left":
const module = new HTMLModule({
sizeConverters: {
paddingLeft: 20,
},
});
This will make paddingLeft a little bit larger on word than the default (which is 15).
This module handles only docx documents, not pptx.
The reason for that is that HTML maps well to docx because they use the same linear flow, eg elements are placed one after the other in the document. However PPTX documents have multiple slides, which are stored in different files, and each element is positioned absolutely in the slide. For example, in PPTX if you have a table element and a paragraph in the same slide, they need to be placed in two "different" blocks.
Lets take a simple example :
Paragraph and table in PPTX
Paragraph and table in DOCX
Here is how these documents map to XML :
<p:spPr>
<a:xfrm>
<a:off x="2376000" y="936000"/>
<a:ext cx="3384000" cy="346320"/>
</a:xfrm>
<a:t>My paragraph</a:t>
</p:spPr>
<p:graphicFrame>
<p:xfrm>
<a:off x="3579480" y="2726640"/>
<a:ext cx="5075280" cy="1439280"/>
</p:xfrm>
<a:tbl>
...
</a:tbl>
</p:graphicFrame>
<w:p>
<w:r>
<w:t>My paragraph</w:t>
</w:r>
</w:p>
<w:tbl>
...
</w:tbl>
As you can see, in docx, the elements come one after the other, without any "placement" information, in pptx however, these are placed absolutely with the <a:xfrm>
and <p:xfrm>
tags.
In standard HTML, you would write this example with :
<p>My paragraph</p>
<table>
...
</table>
which maps very well to docx, but not to pptx.
<img>
To be able to replace the <img>
tag, you have to also have access to the Image Module.
Then, you can do :
const HTMLModule = require("docxtemplater-html-module");
const ImageModule = require("docxtemplater-image-module");
const htmlModule = new HTMLModule({
img: {
Module: ImageModule,
// By default getSize returns the width and height attributes if both are present, or 200x200px as a default value.
getSize: function(data) {
// The html element, for example, if the data is :
// '<img height="20" src="...">'
// you will have data.element.attribs.width = '20'
console.log(data.element);
// The arraybuffer of your image
// (you could use https://github.com/image-size/image-size to calculate the size)
console.log(data.src);
// You return an array in pixel (here we have width 50px and height 100px)
return [50, 100];
}
}
}):
The <img>
tag only supports base64 src, so for example, you can do :
<p>Hello</p>
<img src="data:image/gif;base64,R0lGODlhDAAMAIQZAAAAAAEBAQICAgMDAwQEBCIiIiMjIyQkJCUlJSYmJigoKC0tLaSkpKampqenp6ioqKurq66urrCwsPb29vr6+vv7+/z8/P39/f7+/v///////////////////////////yH+EUNyZWF0ZWQgd2l0aCBHSU1QACwAAAAADAAMAAAFSiCWjRmViVVmZRWWjiurXrBI2uMkFcdjrRfaJQIQCAKQTDBmKAYACAwuswAABoBEDMUgYAkOpWiFeRgUDZIKNnqpLaJLXM2KjaYhADs=" alt="">
Note that HTTP URLs in src will not work, since docxtemplater doesn't know how to load those files.
You can use the following getSize function if you would like to use the same width and height as the image source.
import sizeOf from "image-size";
function getSize (img) {
const buffer = new Buffer(img.src);
return sizeOf(buffer);
}
You can build the es6 into js by running npm run compile
You can test the module with npm test
FAQs
Unknown package
The npm package gw-docxtemplater-modules receives a total of 0 weekly downloads. As such, gw-docxtemplater-modules popularity was classified as not popular.
We found that gw-docxtemplater-modules demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.