hamt-sharding

JavaScript implementation of hamt for use in sharding
Lead Maintainer
Alex Potsides
Table of Contents
Install
> npm install hamt-sharding
Usage
Example
const hamt = require('hamt-sharding')
const crypto = require('crypto-promise')
const hashFn = async (value) => {
return crypto
.createHash('sha256')
.update(value)
.digest()
}
const bucket = hamt({
hashFn: hashFn
})
await bucket.put('key', 'value')
const output = await bucket.get('key')
API
const hamt = require('hamt-sharding')
bucket.put(key, value)
const hamt = require('hamt-sharding')
const bucket = hamt({...})
await bucket.put('key', 'value')
bucket.get(key)
const hamt = require('hamt-sharding')
const bucket = hamt({...})
await bucket.put('key', 'value')
console.info(await bucket.get('key'))
bucket.del(key)
const hamt = require('hamt-sharding')
const bucket = hamt({...})
await bucket.put('key', 'value')
await bucket.del('key', 'value')
console.info(await bucket.get('key'))
bucket.leafCount()
const hamt = require('hamt-sharding')
const bucket = hamt({...})
console.info(bucket.leafCount())
await bucket.put('key', 'value')
console.info(bucket.leafCount())
bucket.childrenCount()
const hamt = require('hamt-sharding')
const bucket = hamt({...})
console.info(bucket.childrenCount())
await bucket.put('key', 'value')
console.info(bucket.childrenCount())
bucket.onlyChild()
bucket.eachLeafSeries()
const hamt = require('hamt-sharding')
const bucket = hamt({...})
await bucket.put('key', 'value')
for await (const child of bucket.eachLeafSeries()) {
console.info(child.value)
}
bucket.serialize(map, reduce)
bucket.asyncTransform(asyncMap, asyncReduce)
bucket.toJSON()
bucket.prettyPrint()
bucket.tableSize()
hamt.isBucket(other)
const hamt = require('hamt-sharding')
const bucket = hamt({...})
console.info(hamt.isBucket(bucket))
console.info(hamt.isBucket(true))
Contribute
Feel free to join in. All welcome. Open an issue!
This repository falls under the IPFS Code of Conduct.
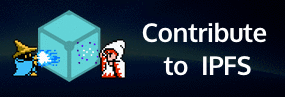
License
MIT