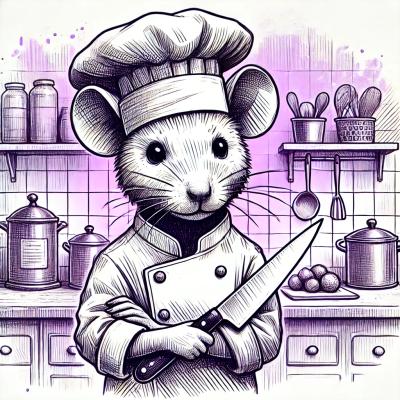
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
hapi-crud-promise
Advanced tools
Easily create CRUD routes on a hapi server by providing a path and promises for each of the actions
Reduce repetitive route setup for basic CRUD apps.
Provide one route and a 5 handlers:
/api/things/{thingId}
And get 5 routes added to your server:
GET /api/things
POST /api/things
GET /api/things/{thingId}
PUT /api/things/{thingId}
DELETE /api/things/{thingId}
const Hapi = require('hapi');
const Joi = require('joi');
const hapiCrudPromise = require('../index');
const server = new Hapi.Server();
server.connection({ host: '127.0.0.1' });
hapiCrudPromise(server, {
path: '/api/things/{thingId}',
config: {
validate: {
query: { // validation only applied to GET (all)
limit: Joi.number().optional()
}
params: { // validation only applied to GET (one), DELETE, and UPDATE routes
thingId: Joi.string().required()
},
payload: Joi.object({ // validation only applied to POST and PUT route
thing: Joi.object({
name: Joi.string().required()
}).required()
})
}
},
crudRead(req) {
return knex('things')
.first()
.where({ id: req.params.thingId });
},
crudReadAll(req) {
return knex('things').limit(req.query.limit);
},
crudUpdate(req) {
return knex('things')
.update(req.payload.thing)
.where({ id: req.params.thingId })
.limit(1)
.returning('*')
.spread((thing) => ({ thing: thing }));
},
crudCreate(req) {
return knex('things')
.insert(req.payload.thing)
.returning('*')
.spread((thing) => ({ thing: thing }));
},
crudDelete(req) {
return knex('things')
.delete()
.where({ id: req.params.thingId })
.limit(1);
}
});
If you have a long path in your route with multiple parameters the last one is special, it identifies the resource you are CRUD-ing and will only be included on validations for GET (one), DELETE, and UPDATE routes
const Hapi = require('hapi');
const Joi = require('joi');
const hapiCrudPromise = require('../index');
const server = new Hapi.Server();
server.connection({ host: '127.0.0.1' });
hapiCrudPromise(server, {
path: '/api/users/{userId}/things/{thingId}',
config: {
validate: {
query: { // validation only applied to GET (all)
limit: Joi.number().optional()
}
params: {
userId: Joi.string().required(), // This and other param validations applied to all routes
thingId: Joi.string().required() // Except this one! only applied to GET (one), DELETE, and UPDATE routes
},
payload: Joi.object({ // validation only applied to POST and PUT route
thing: Joi.object({
name: Joi.string().required()
}).required()
})
}
},
crudRead(req) {
...
},
crudReadAll(req) {
...
},
crudUpdate(req) {
...
},
crudCreate(req) {
...
},
crudDelete(req) {
...
}
});
Contributors wanted. If you are looking for a way to help out browse the Help Wanted issues and find one that looks good to you. If you have an idea to make hapi-crud-promise better submit a pull request.
Checklist for submitting a pull request:
npm run test
- Unit tests must passnpm run test-cov
- Code coverage cannot go downnpm run lint
- New code must have no linter errorsYeah, but with Promises! And active. And the Github repo is still live.
CRUD routes are repetitive. Write less code and go outside.
FAQs
Easily create CRUD routes on a hapi server by providing a path and promises for each of the actions
The npm package hapi-crud-promise receives a total of 2 weekly downloads. As such, hapi-crud-promise popularity was classified as not popular.
We found that hapi-crud-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.