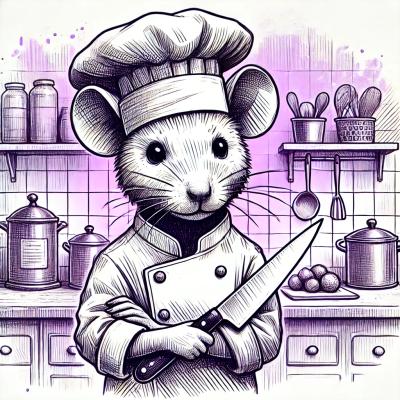
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Efficient Binary heap (priority queue, binary tree) data structure for JavaScript / TypeScript. Includes JavaScript methods, Python's heapq module methods, and Java's PriorityQueue methods.
heap-js is a JavaScript implementation of a binary heap data structure. It provides efficient methods for priority queue operations, such as inserting elements, finding the minimum or maximum element, and removing elements. This package is useful for algorithms that require priority queues, such as Dijkstra's algorithm for shortest paths, Huffman coding, and more.
MinHeap
This feature allows you to create a MinHeap, where the smallest element is always at the root. The code sample demonstrates how to insert elements into the MinHeap, peek at the smallest element, and remove elements in ascending order.
const { MinHeap } = require('heap-js');
const minHeap = new MinHeap();
minHeap.push(3);
minHeap.push(1);
minHeap.push(2);
console.log(minHeap.peek()); // Output: 1
console.log(minHeap.pop()); // Output: 1
console.log(minHeap.pop()); // Output: 2
MaxHeap
This feature allows you to create a MaxHeap, where the largest element is always at the root. The code sample demonstrates how to insert elements into the MaxHeap, peek at the largest element, and remove elements in descending order.
const { MaxHeap } = require('heap-js');
const maxHeap = new MaxHeap();
maxHeap.push(3);
maxHeap.push(1);
maxHeap.push(2);
console.log(maxHeap.peek()); // Output: 3
console.log(maxHeap.pop()); // Output: 3
console.log(maxHeap.pop()); // Output: 2
Custom Comparator
This feature allows you to create a heap with a custom comparator function. The code sample demonstrates how to create a MinHeap for objects with a 'priority' property, and how to insert and remove elements based on their priority.
const { MinHeap } = require('heap-js');
const customHeap = new MinHeap({
compare: (a, b) => a.priority - b.priority
});
customHeap.push({ value: 'task1', priority: 2 });
customHeap.push({ value: 'task2', priority: 1 });
console.log(customHeap.pop().value); // Output: 'task2'
The 'heap' package provides a binary heap implementation with similar functionalities to heap-js. It supports both MinHeap and MaxHeap operations and allows custom comparator functions. However, heap-js is often preferred for its more modern API and better performance in certain use cases.
The 'js-priority-queue' package offers a priority queue implementation using a binary heap. It supports custom comparator functions and provides methods for inserting and removing elements. Compared to heap-js, js-priority-queue is more focused on priority queue operations and may have a simpler API for those specific needs.
The 'priorityqueuejs' package provides a priority queue implementation with a binary heap. It supports custom comparator functions and offers methods for inserting and removing elements. While similar to heap-js, priorityqueuejs is designed to be lightweight and easy to use, making it a good choice for simpler applications.
Efficient Binary heap (priority queue, binary tree) data structure for JavaScript / TypeScript.
Includes JavaScript methods, Python's heapq module methods, and Java's PriorityQueue methods.
Easy to use, known interfaces, tested, and well documented JavaScript binary heap library.
Instances are integer min heap
by default.
It depends on your usage, but for some scenarios it is much faster:
heap vs array: push + pop/unshift 50
heap x 72,130 ops/sec ±0.50% (93 runs sampled)
array x 121 ops/sec ±78.09% (5 runs sampled)
heap vs array: push + peek 20
heap x 622,332 ops/sec ±27.93% (63 runs sampled)
array x 207 ops/sec ±78.18% (5 runs sampled)
heap vs array: push + top(1) of 100
heap x 124,835 ops/sec ±40.37% (61 runs sampled)
array x 123 ops/sec ±78.49% (5 runs sampled)
heap vs array: push + top(50) of 100
heap x 59,210 ops/sec ±17.66% (82 runs sampled)
array x 125 ops/sec ±78.79% (5 runs sampled)
Heap.nlargest
as heapq.Heap.nsmallest
as heapq.top
/ bottom
input to force an integer.The main breaking change is that now top(N)
does NOT sort the output. It should not be part of the spec for a priority queue, the output should be the top N elements. It will be partially ordered with the peek at index 0
by definition, that is all.
top(N)
is unordered, only the first element is guaranteed to be the top priority element.Iterator
interface and iterator()
method.// Basic usage example
import Heap from 'heap-js';
const minHeap = new Heap();
const maxHeap = new Heap(Heap.maxComparator);
minHeap.init([5, 18, 1]);
minHeap.push(2);
console.log(minHeap.peek()); //> 1
console.log(minHeap.pop()); //> 1
console.log(minHeap.peek()); //> 2
// Iterator
maxHeap.init([3, 4, 1, 12, 8]);
for (const value of maxHeap) {
console.log('Next top value is', value);
}
// Priority Queue usage example
const { Heap } = require('heap-js');
const tasks = db.collection.find().toArray();
const customPriorityComparator = (a, b) => a.priority - b.priority;
const priorityQueue = new Heap(customPriorityComparator);
priorityQueue.init(tasks);
// priorityQueue === priorityQueue.iterator()
for (const task of priorityQueue) {
// Do something
}
// Python-like static methods example
import { Heap } from 'heap-js';
const numbers = [2, 3, 7, 5];
Heap.heapify(numbers);
console.log(numbers); //> [ 2, 3, 5, 7 ]
Heap.heappush(numbers, 1);
console.log(numbers); //> [ 1, 2, 5, 7, 3 ]
yarn add heap-js # if you use yarn
npm install --save heap-js # if you use npm
new Heap([comparator]);
Basic comparators already included:
Heap.minComparator
Integer min heap (default)Heap.maxComparator
Integer max heaplength
of the heaplimit
amount of elements in the heappop()
the top elementpush(...elements)
one or more elements to the heappushpop(element)
faster than push
& pop
replace(element)
faster than pop
& push
top(number?)
most valuable elements from the heapbottom(number?)
least valuable elements from the heapPriorityQueue
interface:add(element)
to the heapaddAll([elment, element, ... ])
to the heap, faster than loop add
clear()
clone()
comparator()
contains(element, fn?)
element()
alias of peek()
isEmpty()
iterator()
returns this
because it is iterableoffer(element)
alias of add(element)
peek()
poll()
alias of pop()
remove(element?)
removeAll()
alias of clear()
size()
alias of length
toArray()
toString()
To do:
containsAll
equals
retainAll
heapq
interface:Heap.heapify(array, comparator?)
that converts an array to an array-heapHeap.heappop(heapArray, comparator?)
that takes the peek of the array-heapHeap.heappush(heapArray, item, comparator?)
that appends elements to the array-heapHeap.heappushpop(heapArray, item, comparator?)
faster than heappush
& heappop
Heap.heapreplace(heapArray, item, comparator?)
faster than heappop
& heappush
Heap.nlargest(n, iterable, comparator?)
that gets the n
most valuable elements of an iterableHeap.nsmallest(n, iterable, comparator?)
that gets the n
least valuable elements of an iterableExtras:
Heap.heaptop(n, heapArray, comparator?)
that returns the n
most valuable elements of the array-heapHeap.heapbottom(n, heapArray, comparator?)
that returns the n
least valuable elements of the array-heapTo do:
merge(...iterables, comparator?)
Extensive documentation included at ./dist/docs
. It'll be published to gh-pages
in a next release.
Development of Heap.js happens in the open on GitHub, and I am grateful to the community for contributing bugfixes and improvements.
yarn
npm run test
npm run benchmarks
Heap.js is BSD licensed.
FAQs
Efficient Binary heap (priority queue, binary tree) data structure for JavaScript / TypeScript. Includes JavaScript methods, Python's heapq module methods, and Java's PriorityQueue methods.
The npm package heap-js receives a total of 611,620 weekly downloads. As such, heap-js popularity was classified as popular.
We found that heap-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.