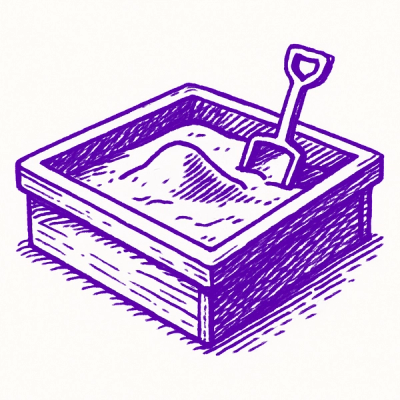
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
html-minifier
Advanced tools
The html-minifier npm package is a highly configurable, well-tested, JavaScript-based HTML minifier, with lint-like capabilities. It compresses HTML files by removing unnecessary whitespace, comments, and other unneeded characters without changing the functionality of the HTML. This can result in faster page load times and reduced bandwidth usage.
Minify HTML
This feature collapses whitespace and strips out unnecessary spaces and line breaks to minimize the HTML file size.
const htmlMinifier = require('html-minifier').minify;
let result = htmlMinifier('<p> Some HTML </p>', {collapseWhitespace: true});
console.log(result); // Output: '<p>Some HTML</p>'
Remove Comments
This feature removes HTML comments to reduce the file size.
const htmlMinifier = require('html-minifier').minify;
let result = htmlMinifier('<!-- This is a comment --> <div>Content</div>', {removeComments: true});
console.log(result); // Output: '<div>Content</div>'
Remove Optional Tags
This feature removes optional tags like <html>, <head>, <body>, and <colgroup> when they are not necessary.
const htmlMinifier = require('html-minifier').minify;
let result = htmlMinifier('<html><head><title>Title</title></head><body><p>Content</p></body></html>', {removeOptionalTags: true});
console.log(result); // Output: '<title>Title</title><p>Content</p>'
Minify CSS
This feature minifies inline CSS reducing its size by removing unnecessary characters and whitespace.
const htmlMinifier = require('html-minifier').minify;
let result = htmlMinifier('<style> .class { font-size: 18px; } </style>', {minifyCSS: true});
console.log(result); // Output: '<style>.class{font-size:18px}</style>'
Minify JavaScript
This feature minifies inline JavaScript code by removing unnecessary characters and whitespace.
const htmlMinifier = require('html-minifier').minify;
let result = htmlMinifier('<script> var name = "Your Name"; </script>', {minifyJS: true});
console.log(result); // Output: '<script>var name="Your Name";</script>'
This package is a gulp plugin that minifies HTML. It wraps around html-minifier and provides a similar set of features but is designed to be used within the gulp streaming build system.
Minimize is another HTML minifier with a focus on configurability and performance. It offers a different API and plugin system compared to html-minifier, which might be preferred in certain development environments.
Prettydiff is a code beautifier as well as a minifier. It supports HTML, CSS, and JavaScript. While it provides similar minification features, it also includes code comparison and beautification options, which html-minifier does not.
HTMLMinifier is a highly configurable, well-tested, JavaScript-based HTML minifier.
See corresponding blog post for all the gory details of how it works, description of each option, testing results and conclusions.
Test suite is available online.
Also see corresponding Ruby wrapper, and for Node.js, Grunt plugin, Gulp module, Koa middleware wrapper and Express middleware wrapper.
For lint-like capabilities take a look at HTMLLint.
How does HTMLMinifier compare to other solutions — HTML Minifier from Will Peavy (1st result in Google search for "html minifier") as well as htmlcompressor.com and minimize?
Site | Original size (KB) | HTMLMinifier | minimize | Will Peavy | htmlcompressor.com |
---|---|---|---|---|---|
46 | 42 | 46 | 48 | 46 | |
HTMLMinifier | 125 | 98 | 111 | 117 | 111 |
207 | 165 | 200 | 224 | 200 | |
Stack Overflow | 253 | 195 | 207 | 215 | 204 |
Bootstrap CSS | 271 | 260 | 269 | 228 | 269 |
BBC | 298 | 239 | 290 | 291 | 280 |
Amazon | 422 | 316 | 412 | 425 | n/a |
NBC | 553 | 530 | 552 | 553 | 534 |
Wikipedia | 565 | 461 | 548 | 569 | 548 |
New York Times | 678 | 606 | 675 | 670 | n/a |
Eloquent Javascript | 870 | 815 | 840 | 864 | n/a |
ES6 table | 5911 | 5051 | 5595 | n/a | n/a |
ES draft | 6126 | 5495 | 5664 | n/a | n/a |
Most of the options are disabled by default.
Option | Description | Default |
---|---|---|
caseSensitive | Treat attributes in case sensitive manner (useful for custom HTML tags) | false |
collapseBooleanAttributes | Omit attribute values from boolean attributes | false |
collapseInlineTagWhitespace | Don't leave any spaces between display:inline; elements when collapsing. Must be used in conjunction with collapseWhitespace=true | false |
collapseWhitespace | Collapse white space that contributes to text nodes in a document tree | false |
conservativeCollapse | Always collapse to 1 space (never remove it entirely). Must be used in conjunction with collapseWhitespace=true | false |
continueOnParseError | Handle parse errors instead of aborting. | false |
customAttrAssign | Arrays of regex'es that allow to support custom attribute assign expressions (e.g. '<div flex?="{{mode != cover}}"></div>' ) | [ ] |
customAttrCollapse | Regex that specifies custom attribute to strip newlines from (e.g. /ng-class/ ) | |
customAttrSurround | Arrays of regex'es that allow to support custom attribute surround expressions (e.g. <input {{#if value}}checked="checked"{{/if}}> ) | [ ] |
customEventAttributes | Arrays of regex'es that allow to support custom event attributes for minifyJS (e.g. ng-click ) | [ /^on[a-z]{3,}$/ ] |
decodeEntities | Use direct Unicode characters whenever possible | false |
html5 | Parse input according to HTML5 specifications | true |
ignoreCustomComments | Array of regex'es that allow to ignore certain comments, when matched | [ /^!/ ] |
ignoreCustomFragments | Array of regex'es that allow to ignore certain fragments, when matched (e.g. <?php ... ?> , {{ ... }} , etc.) | [ /<%[\s\S]*?%>/, /<\?[\s\S]*?\?>/ ] |
includeAutoGeneratedTags | Insert tags generated by HTML parser | true |
keepClosingSlash | Keep the trailing slash on singleton elements | false |
maxLineLength | Specify a maximum line length. Compressed output will be split by newlines at valid HTML split-points | |
minifyCSS | Minify CSS in style elements and style attributes (uses clean-css) | false (could be true , Object , Function(text, type) ) |
minifyJS | Minify JavaScript in script elements and event attributes (uses UglifyJS) | false (could be true , Object , Function(text, inline) ) |
minifyURLs | Minify URLs in various attributes (uses relateurl) | false (could be String , Object , Function(text) ) |
preserveLineBreaks | Always collapse to 1 line break (never remove it entirely) when whitespace between tags include a line break. Must be used in conjunction with collapseWhitespace=true | false |
preventAttributesEscaping | Prevents the escaping of the values of attributes | false |
processConditionalComments | Process contents of conditional comments through minifier | false |
processScripts | Array of strings corresponding to types of script elements to process through minifier (e.g. text/ng-template , text/x-handlebars-template , etc.) | [ ] |
quoteCharacter | Type of quote to use for attribute values (' or ") | |
removeAttributeQuotes | Remove quotes around attributes when possible | false |
removeComments | Strip HTML comments | false |
removeEmptyAttributes | Remove all attributes with whitespace-only values | false (could be true , Function(attrName, tag) ) |
removeEmptyElements | Remove all elements with empty contents | false |
removeOptionalTags | Remove optional tags | false |
removeRedundantAttributes | Remove attributes when value matches default. | false |
removeScriptTypeAttributes | Remove type="text/javascript" from script tags. Other type attribute values are left intact | false |
removeStyleLinkTypeAttributes | Remove type="text/css" from style and link tags. Other type attribute values are left intact | false |
removeTagWhitespace | Remove space between attributes whenever possible. Note that this will result in invalid HTML! | false |
sortAttributes | Sort attributes by frequency | false |
sortClassName | Sort style classes by frequency | false |
trimCustomFragments | Trim white space around ignoreCustomFragments . | false |
useShortDoctype | Replaces the doctype with the short (HTML5) doctype | false |
Minifier options like sortAttributes
and sortClassName
won't impact the plain-text size of the output. However, they form long repetitive chains of characters that should improve compression ratio of gzip used in HTTP compression.
If you have chunks of markup you would like preserved, you can wrap them <!-- htmlmin:ignore -->
.
SVG tags are automatically recognized, and when they are minified, both case-sensitivity and closing-slashes are preserved, regardless of the minification settings used for the rest of the file.
HTMLMinifier can't work with invalid or partial chunks of markup. This is because it parses markup into a tree structure, then modifies it (removing anything that was specified for removal, ignoring anything that was specified to be ignored, etc.), then it creates a markup out of that tree and returns it.
Input markup (e.g. <p id="">foo
)
↓
Internal representation of markup in a form of tree (e.g. { tag: "p", attr: "id", children: ["foo"] }
)
↓
Transformation of internal representation (e.g. removal of id
attribute)
↓
Output of resulting markup (e.g. <p>foo</p>
)
HTMLMinifier can't know that original markup was only half of the tree; it does its best to try to parse it as a full tree and it loses information about tree being malformed or partial in the beginning. As a result, it can't create a partial/malformed tree at the time of the output.
From NPM for use as a command line app:
npm install html-minifier -g
From NPM for programmatic use:
npm install html-minifier
From Git:
git clone git://github.com/kangax/html-minifier.git
cd html-minifier
npm link .
Note that almost all options are disabled by default. For command line usage please see html-minifier --help
for a list of available options. Experiment and find what works best for you and your project.
html-minifier --collapse-whitespace --remove-comments --remove-optional-tags --remove-redundant-attributes --remove-script-type-attributes --remove-tag-whitespace --use-short-doctype --minify-css true --minify-js true
var minify = require('html-minifier').minify;
var result = minify('<p title="blah" id="moo">foo</p>', {
removeAttributeQuotes: true
});
result; // '<p title=blah id=moo>foo</p>'
Benchmarks for minified HTML:
node benchmark.js
FAQs
Highly configurable, well-tested, JavaScript-based HTML minifier.
The npm package html-minifier receives a total of 2,682,277 weekly downloads. As such, html-minifier popularity was classified as popular.
We found that html-minifier demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.