i18next-hmr

I18Next HMR 🔥webpack plugin that allows to reload translation resources on client & server
Requirements
- Node.js v8 or above
- Webpack 4.x
Installation
$ npm install --save-dev i18next-hmr
Usage
Add the plugin to your webpack config (or nextjs).
const { I18NextHMRPlugin } = require('i18next-hmr/plugin');
module.exports = {
...
plugins: [
new I18NextHMRPlugin({
localesDir: path.resolve(__dirname, 'static/locales'),
})
]
};
const i18next = require('i18next');
i18next.init(options, callback);
if (process.env.NODE_ENV === 'development') {
const { applyClientHMR } = require('i18next-hmr');
applyClientHMR(i18next);
}
const express = require('express');
const i18n = require('./i18n');
if (process.env.NODE_ENV === 'development') {
const { applyServerHMR } = require('i18next-hmr');
applyServerHMR(i18n);
}
const port = process.env.PORT || 3000;
(async () => {
const server = express();
server.get('*', (req, res) => handle(req, res));
await server.listen(port);
console.log(`> Ready on http://localhost:${port}`);
})();
Start the app with NODE_ENV=development
Server side
The lib will trigger i18n.reloadResources([lang], [ns])
on the server side with lang
& namespace
extracted from the translation filename that was changed.
Client side
The lib will invoke webpacks hmr to update client side, that will re-fetch (with cache killer) the updated translation json, and trigger i18n.changelanguage(lang)
to trigger listeners (in React app it will update the UI).
Example
A working nextjs
with next-i18next
example can be found in the examples
folder.
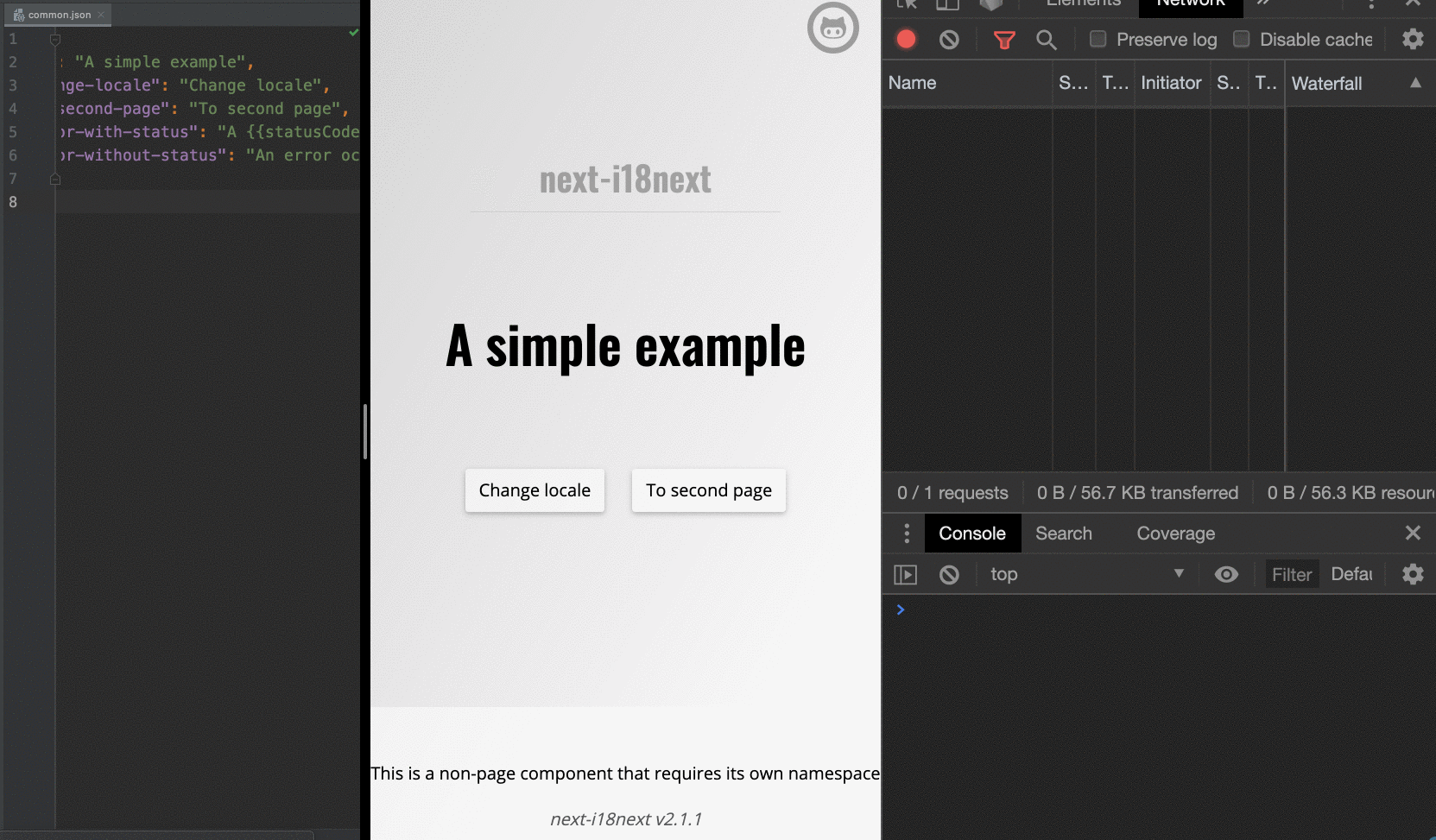