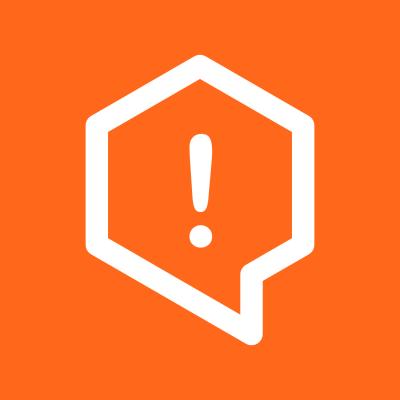
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
A javascript library to validate, format and convert IBAN (International Bank Account Number) and BBAN (Basic Bank Account Number)
The 'iban' npm package provides utilities for validating, formatting, and generating International Bank Account Numbers (IBANs). It is useful for financial applications that need to handle IBANs in a standardized way.
Validation
This feature allows you to validate whether a given IBAN is correct. The code sample checks if the provided IBAN is valid and returns a boolean value.
const iban = require('iban');
const isValid = iban.isValid('DE89370400440532013000');
console.log(isValid); // true
Formatting
This feature formats an IBAN into a more readable form by adding spaces. The code sample demonstrates how to format an IBAN string.
const iban = require('iban');
const formattedIban = iban.printFormat('DE89370400440532013000');
console.log(formattedIban); // 'DE89 3704 0044 0532 0130 00'
Generation
This feature generates an IBAN from a given Basic Bank Account Number (BBAN) and country code. The code sample shows how to generate an IBAN from a BBAN.
const iban = require('iban');
const generatedIban = iban.fromBBAN('DE', '370400440532013000');
console.log(generatedIban); // 'DE89370400440532013000'
The 'ibantools' package provides similar functionalities for validating and formatting IBANs. It also includes additional features like generating IBANs from BBANs and country codes. Compared to 'iban', 'ibantools' offers a more comprehensive set of tools for handling IBANs.
IBAN and BBAN validation, formatting and conversion in Javascript. Check the demo on demo page to try it.
IBAN.js follows the ISO 13616 IBAN Registry technical specification.
IBAN.js is compatible with both commonjs and AMD module definition. It can be used as a node.js module and in the browser. It also has a bower manifest, a Typescript definition and a Meteor wrapper.
var IBAN = require('iban');
IBAN.isValid('hello world'); // false
IBAN.isValid('BE68539007547034'); // true
Using a module loader (AMD or commonjs) or directly through the global IBAN
object:
<script src="iban.js"></script>
<script>
// the API is now accessible from the window.IBAN global object
IBAN.isValid('hello world'); // false
IBAN.isValid('BE68539007547034'); // true
</script>
The library is also available from the typescript language. To do this, download the definition and add a reference to this:
/// <reference path="iban.d.ts" />
IBAN.isValid('hello world');
IBAN.isValid('BE68539007547034');
A wrapper package for the Meteor framework is available here.
* isValid(iban)
* toBBAN(iban, separator)
* fromBBAN(countryCode, bban)
* isValidBBAN(countryCode, bban)
* printFormat(iban, separator)
* electronicFormat(iban)
FAQs
A javascript library to validate, format and convert IBAN (International Bank Account Number) and BBAN (Basic Bank Account Number)
The npm package iban receives a total of 116,913 weekly downloads. As such, iban popularity was classified as popular.
We found that iban demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.