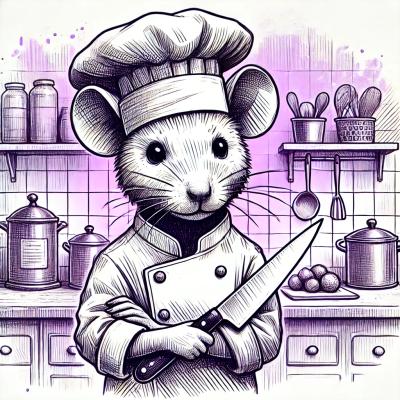
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Exposes a way to enforce an interface on classes.
npm install --save interface
To enforce an interface, first create a new Interface
and pass it the function names that you want to enforce.
const MyInterface = Interface.create('myMethodA', 'myMethodB')
or alternatively
const MyInterface = new Interface('myMethodA', 'myMethodB')
Next, just make your class extend from the interface. Make sure you call super()
within your class's constructor.
class MyClass extends MyInterface {
constructor () {
super()
}
myMethodA () {
// ...implementation goes here
}
}
Now, whenever you try to instantiate MyClass
, the interface will be enforced.
const instance = new MyClass()
// throws a new error with the message:
// 'The following function(s) need to be implemented for class MyClass: myMethodB'
Of course, the interface is enforced on all subclasses as well.
class MySubClass extends MyClass {
constructor () {
super()
}
myMethodA () {
// override 'myMethodA'
}
}
const instance = new MySubClass()
// still throws an error with the message:
// 'The following function(s) need to be implemented for class MyClass: myMethodB'
Interfaces can be enforced for classes defined the old way too.
const inherits = require('util').inherits
const MyInterface = new Interface('myMethodA', 'myMethodB', 'myMethodC')
function MyClass () {
MyInterface.call(this)
}
inherits(MyClass, MyInterface)
MyClass.prototype.myMethodA = function () {
// implementation
}
function MySubClass () {
MyClass.call(this)
}
// inherit prototype of parent class
inherits(MySubClass, MyClass)
MySubClass.prototype.myMethodB = function () {
// implementation
}
var instance = new MySubClass()
// throws an error with the message:
// 'The following function(s) need to be implemented for class MyClass: myMethodC'
You can also enforce that arbitrary objects match an interface by using
the isImplementedBy
method.
const MyInterface = new Interface('myMethod')
class MyClass {
myMethod () {
// some implementation
}
}
class MyOtherClass {
myOtherMethod () {
// some implementation
}
}
const instanceA = new MyClass()
const instanceB = new MyOtherClass()
MyInterface.isImplementedBy(instanceA) // returns true
MyInterface.isImplementedBy(instanceB) // returns false
FAQs
Enforce an interface on classes
The npm package interface receives a total of 3,677 weekly downloads. As such, interface popularity was classified as popular.
We found that interface demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.