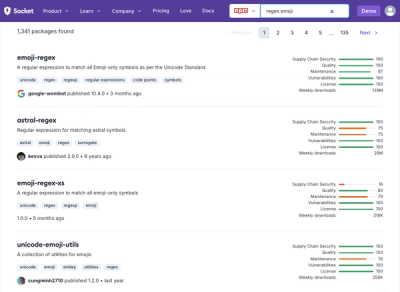
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
A simple, lightweight & framework agnostic JSON:API client for Kitsu.io and other APIs
Check out the Migration Guide for breaking changes and new features in 4.x
yarn add kitsu
npm install kitsu
Package | Package Size | Node | Chrome | Firefox | Safari | Edge | IE |
---|---|---|---|---|---|---|---|
Default | 17.9 kb | 6+ | 49+ | 57+ | 10.1+ | 15+ | |
Legacy | 19.9 kb | 6+ | 4+ | 3+ | 3.1+ | 12+ | 8+ |
Node | 14.5 kb | 6+ |
A GET response by a JSON:API server returns:
{
data: {
id: '1'
type: 'articles'
attributes: {
title: 'JSON API paints my bikeshed'
}
relationships: {
author: {
data: {
id: '42'
type: 'people'
}
}
}
}
included: [
{
id: '42'
type: 'people'
attributes: {
name: 'John'
}
}
]
}
A GET request with kitsu
returns:
{
data: {
id: '1'
type: 'articles'
title: 'JSON API paints my bikeshed'
author: {
id: '42'
type: 'people'
name: 'John'
}
}
}
import Kitsu from 'kitsu' // ES Modules and Babel
const Kitsu = require('kitsu') // CommonJS and Browserify
const Kitsu = require('kitsu/lib/legacy') // Legacy IE8+ support
const Kitsu = require('kitsu/lib/node') // Lighter node-only package
const api = new Kitsu() // For kitsu.io developers
const api = new Kitsu({ // For other JSON:API servers
baseURL: 'https://api.example/2' // e.g https://api.example/2
})
const { data } = await api.get('anime') // Using with async/await
api.get('anime') // Using with Promises
.then(({ data }) => { ... })
.catch(err => throw err)
// Fetching resources with get() or fetch()
api.get('anime', { params }, { headers }) // Collection of resources
api.get('anime/1') // Single resource
api.get('anime/1/episodes') // Single resource's relationship
// Creating resources with post() or create()
api.create('post', {
content: 'some content'
})
// Updating resources with patch() or update()
api.update('post', {
id: '1',
content: 'new content'
})
// Deleting resources
api.remove('post', 1)
If you're working with Kitsu.io's API, their API docs lists all available resources with their attributes and relationships
Parameters
options
Object Options
options.baseURL
string Set the API endpoint (default https://kitsu.io/api/edge
)options.headers
Object Additional headers to send with requestsoptions.camelCaseTypes
boolean If true, the type
value will be camelCased, e.g library-entries
and library_entries
become libraryEntries
(default true
)options.resourceCase
string kebab
, snake
or none
. If kebab
, /libraryEntries
will become /library-entries
. If snake
, /libraryEntries
will become /library_entries
, If none
, /libraryEntries
will be unchanged (default kebab
)options.pluralize
boolean If true
, /user
will become /users
in the URL request and type
will be pluralized in post, patch and delete requests - user
-> users
(default true
)options.timeout
number Set the request timeout in milliseconds (default 30000
)Examples
// If using Kitsu.io's API
const api = new Kitsu()
// If using another API server
const api = new Kitsu({
baseURL: 'https://api.example.org/2'
})
// Set a `user-agent` and an `authorization` token
const api = new Kitsu({
headers: {
'User-Agent': 'MyApp/1.0.0 (github.com/username/repo)',
Authorization: 'Bearer 1234567890'
}
})
Fetch resources
Aliases: fetch
Parameters
model
string Model to fetch data fromparams
Object JSON-API request queries (optional, default {}
)
params.page
Object jsonapi.org/format/#fetching-pagination
params.fields
Object Return a sparse fieldset with only the included attributes/relationships jsonapi.org/format/#fetching-sparse-fieldsetsparams.filter
Object Filter dataset by attribute values jsonapi.org/format/#fetching-filteringparams.sort
string Sort dataset by one or more comma separated attributes (prepend -
for descending order) jsonapi.org/format/#fetching-sortingparams.include
string Include relationship data jsonapi.org/format/#fetching-includesheaders
Object Additional headers to send with request (optional, default {}
)Examples
// Get a specific user's name & birthday
api.get('users', {
fields: {
users: 'name,birthday'
},
filter: {
name: 'wopian'
}
})
// Get a collection of anime resources and their categories
api.get('anime', {
include: 'categories'
})
// Get a single resource and its relationships by ID (method one)
api.get('anime', {
include: 'categories',
filter: { id: '2' }
})
// Get a single resource and its relationships by ID (method two)
api.get('anime/2', {
include: 'categories'
})
// Get a resource's relationship data only
api.get('anime/2/categories')
Returns Object JSON-parsed response
Get the current headers or add additional headers
Examples
// Receive all the headers
api.headers
// Receive a specific header
api.headers['User-Agent']
// Add or update a header
api.headers['Authorization'] = 'Bearer 1234567890'
Returns Object All the current headers
Check if the client is authenticated (oAuth2/Authorization header)
Examples
if (api.isAuth) console.log('Authenticated')
else console.log('Not authenticated')
Returns boolean
Update a resource
Aliases: update
Parameters
model
string Model to update data inbody
Object Data to send in the requestheaders
Object Additional headers to send with request (optional, default {}
)Examples
// Update a user's post (Note: For Kitsu.io, posts cannot be edited 30 minutes after creation)
api.update('posts', {
id: '12345678',
content: 'Goodbye World'
})
Returns Object JSON-parsed response
Create a new resource
Aliases: create
Parameters
model
string Model to create a resource underbody
Object Data to send in the requestheaders
Object Additional headers to send with request (optional, default {}
)Examples
// Post to a user's own profile
api.create('posts', {
content: 'Hello World',
targetUser: {
id: '42603',
type: 'users'
},
user: {
id: '42603',
type: 'users'
}
})
Returns Object JSON-parsed response
Remove a resource
Parameters
model
string Model to remove data fromid
(string | number) Resource ID to removeheaders
Object Additional headers to send with request (optional, default {}
)Examples
// Delete a user's post
api.remove('posts', 123)
Returns Object JSON-parsed response
Get the authenticated user's data
Note: Requires the JSON:API server to support filter[self]=true
Parameters
params
Object JSON-API request queries (optional, default {}
)
headers
Object Additional headers to send with request (optional, default {}
)Examples
// Receive all attributes
api.self()
// Receive a sparse fieldset
api.self({
fields: 'name,birthday'
})
Returns Object JSON-parsed response
See CONTRIBUTING
See CHANGELOG
All code released under MIT
FAQs
A simple, lightweight & framework agnostic JSON:API client using Axios
The npm package kitsu receives a total of 3,542 weekly downloads. As such, kitsu popularity was classified as popular.
We found that kitsu demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.