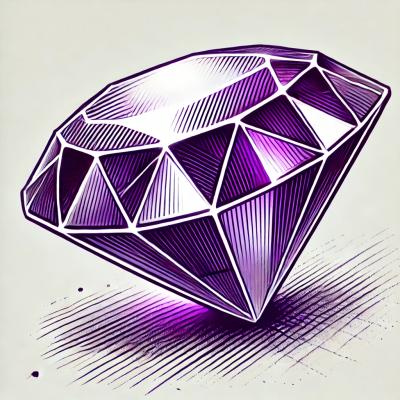
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
A tiny (366B) and fast utility to "deep clone" Objects, Arrays, Dates, RegExps, and more!
The klona npm package is a JavaScript utility for deep cloning objects. It allows developers to create a deep copy of an object, ensuring that changes to the new object do not affect the original object. This is particularly useful when working with complex data structures or when you need to ensure data immutability.
Deep cloning of objects
This feature allows you to create a deep clone of an object, which means that nested objects are also cloned, and changes to the cloned object do not affect the original object.
{"const klona = require('klona');\nconst original = { a: 1, b: { c: 2 } };\nconst copy = klona(original);\ncopy.b.c = 3;\nconsole.log(original.b.c); // 2\nconsole.log(copy.b.c); // 3"}
Deep cloning of arrays
Similar to object cloning, klona can also deep clone arrays, including nested arrays, ensuring that modifications to the cloned array do not affect the original array.
{"const klona = require('klona');\nconst original = [1, [2, 3], [4, 5]];\nconst copy = klona(original);\ncopy[1][0] = 'changed';\nconsole.log(original[1][0]); // 2\nconsole.log(copy[1][0]); // 'changed'"}
Cloning class instances
klona is capable of cloning instances of classes, allowing you to duplicate an instance and modify the copy without affecting the original instance.
{"const klona = require('klona');\nclass Example {\n constructor(value) {\n this.value = value;\n }\n}\nconst original = new Example(1);\nconst copy = klona(original);\ncopy.value = 2;\nconsole.log(original.value); // 1\nconsole.log(copy.value); // 2"}
Lodash is a popular utility library that includes a `cloneDeep` function for deep cloning objects. It is more feature-rich than klona but also larger in size, which might be a consideration for projects where bundle size is a concern.
deep-copy is another npm package that provides deep cloning functionality. It offers similar capabilities to klona but may have different performance characteristics or API nuances.
rfdc (Really Fast Deep Clone) is a package that focuses on performance for deep cloning objects and arrays. It claims to be faster than other deep cloning methods, which might make it a preferred choice for performance-critical applications.
Unlike a "shallow copy" (eg, Object.assign
), a "deep clone" recursively traverses a source input and copies its values — instead of references to its values — into a new instance of that input. The result is a structurally equivalent clone that operates independently of the original source and controls its own values.
Additionally, this module is delivered as:
dist/klona.mjs
dist/klona.js
dist/klona.min.js
Why "klona"? It's "clone" in Swedish.
What's with the sheep? Dolly.
$ npm install --save klona
import klona from 'klona';
const input = {
foo: 1,
bar: {
baz: 2,
bat: {
hello: 'world'
}
}
};
const output = klona(input);
// exact copy of original
assert.deepStrictEqual(input, output);
// applying deep updates...
output.bar.bat.hola = 'mundo';
output.bar.baz = 99;
// ...doesn't affect source!
console.log(
JSON.stringify(input, null, 2)
);
// {
// "foo": 1,
// "bar": {
// "baz": 2,
// "bat": {
// "hello": "world"
// }
// }
// }
Returns: typeof input
Returns a deep copy/clone of the input.
via Node.js v10.13.0
Validation:
✘ JSON.stringify (FAILED @ "initial copy")
✘ fast-clone (FAILED @ "initial copy")
✔ lodash
✔ clone-deep
✘ deep-copy (FAILED @ "initial copy")
✔ depcopy
✔ klona
Benchmark:
JSON.stringify x 37,803 ops/sec ±0.68% (89 runs sampled)
fast-clone x 24,210 ops/sec ±0.81% (91 runs sampled)
lodash x 40,563 ops/sec ±1.10% (94 runs sampled)
clone-deep x 85,020 ops/sec ±0.17% (95 runs sampled)
deep-copy x 116,139 ops/sec ±0.29% (96 runs sampled)
depcopy x 24,392 ops/sec ±0.71% (96 runs sampled)
klona x 274,496 ops/sec ±0.15% (99 runs sampled)
MIT © Luke Edwards
FAQs
A tiny (240B to 501B) and fast utility to "deep clone" Objects, Arrays, Dates, RegExps, and more!
The npm package klona receives a total of 0 weekly downloads. As such, klona popularity was classified as not popular.
We found that klona demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.