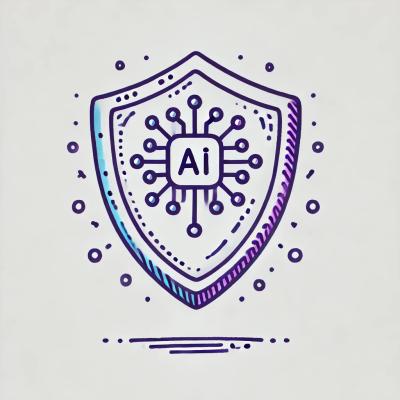
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
libnpmpublish
Advanced tools
The libnpmpublish package is a library for programmatically publishing npm packages. It provides a set of functions to handle the publishing process, including authentication, package validation, and publishing to the npm registry.
Authentication
This feature allows you to authenticate with the npm registry using your credentials. The code sample demonstrates how to use the `getAuth` function from the `libnpm` package to obtain authentication details.
const libnpmpublish = require('libnpmpublish');
const { getAuth } = require('libnpm');
async function authenticate() {
const auth = await getAuth({
scope: '@my-scope',
registry: 'https://registry.npmjs.org/'
});
return auth;
}
authenticate().then(auth => console.log(auth));
Package Validation
This feature allows you to validate your package before publishing it. The code sample demonstrates how to read a `package.json` file and validate its contents using the `validate` function from `libnpmpublish`.
const libnpmpublish = require('libnpmpublish');
const fs = require('fs');
async function validatePackage() {
const pkg = JSON.parse(fs.readFileSync('package.json', 'utf8'));
const validation = await libnpmpublish.validate(pkg);
console.log(validation);
}
validatePackage();
Publishing
This feature allows you to publish your npm package to the registry. The code sample demonstrates how to read a `package.json` file and a tarball file, and then publish the package using the `publish` function from `libnpmpublish`.
const libnpmpublish = require('libnpmpublish');
const fs = require('fs');
async function publishPackage() {
const pkg = JSON.parse(fs.readFileSync('package.json', 'utf8'));
const tarballData = fs.readFileSync('package.tgz');
const auth = { token: 'your-npm-token' };
await libnpmpublish.publish(pkg, tarballData, auth);
console.log('Package published successfully');
}
publishPackage();
The npm-registry-client package provides a client for interacting with the npm registry. It offers similar functionalities such as authentication, package validation, and publishing. However, it is more low-level compared to libnpmpublish and requires more manual handling of the publishing process.
The np package is a command-line tool for publishing npm packages. It automates the entire publishing process, including version bumping, git tagging, and publishing to the npm registry. Unlike libnpmpublish, which is a library for programmatic use, np is designed for use from the command line.
The publish-please package is a tool that helps ensure best practices when publishing npm packages. It provides pre-publish checks and hooks to validate the package before publishing. While it offers some similar functionalities to libnpmpublish, it is more focused on enforcing best practices and is used as a pre-publish tool rather than a publishing library.
libnpmpublish
is a Node.js
library for programmatically publishing and unpublishing npm packages. Give
it a manifest as an object and a tarball as a Buffer, and it'll put them on
the registry for you.
const { publish, unpublish } = require('libnpmpublish')
$ npm install libnpmpublish
opts
for libnpmpublish
commandslibnpmpublish
uses
npm-registry-fetch
. Most options
are passed through directly to that library, so please refer to its own
opts
documentation for
options that can be passed in.
A couple of options of note:
opts.defaultTag
- registers the published package with the given tag,
defaults to latest
.
opts.access
- tells the registry whether this package should be
published as public
or restricted
. Only applies to scoped
packages. Defaults to public
.
opts.token
- can be passed in and will be used as the authentication
token for the registry. For other ways to pass in auth details, see the
n-r-f docs.
opts.provenance
- when running in a supported CI environment, will trigger
the generation of a signed provenance statement to be published alongside
the package. Mutually exclusive with the provenanceFile
option.
opts.provenanceFile
- specifies the path to an externally-generated
provenance statement to be published alongside the package. Mutually
exclusive with the provenance
option. The specified file should be a
Sigstore Bundle
containing a DSSE-packaged
provenance statement.
> libpub.publish(manifest, tarData, [opts]) -> Promise
Sends the package represented by the manifest
and tarData
to the
configured registry.
manifest
should be the parsed package.json
for the package being
published (which can also be the manifest pulled from a packument, a git
repo, tarball, etc.)
tarData
is a Buffer
of the tarball being published.
If opts.npmVersion
is passed in, it will be used as the _npmVersion
field in the outgoing packument. You may put your own user-agent string in
there to identify your publishes.
If opts.algorithms
is passed in, it should be an array of hashing
algorithms to generate integrity
hashes for. The default is ['sha512']
,
which means you end up with dist.integrity = 'sha512-deadbeefbadc0ffee'
.
Any algorithm supported by your current node version is allowed -- npm
clients that do not support those algorithms will simply ignore the
unsupported hashes.
// note that pacote.manifest() and pacote.tarball() can also take
// any spec that npm can install. a folder shown here, since that's
// far and away the most common use case.
const path = '/a/path/to/your/source/code'
const pacote = require('pacote') // see: http://npm.im/pacote
const manifest = await pacote.manifest(path)
const tarData = await pacote.tarball(path)
await libpub.publish(manifest, tarData, {
npmVersion: 'my-pub-script@1.0.2',
token: 'my-auth-token-here'
}, opts)
// Package has been published to the npm registry.
> libpub.unpublish(spec, [opts]) -> Promise
Unpublishes spec
from the appropriate registry. The registry in question may
have its own limitations on unpublishing.
spec
should be either a string, or a valid
npm-package-arg
parsed spec object. For
legacy compatibility reasons, only tag
and version
specs will work as
expected. range
specs will fail silently in most cases.
await libpub.unpublish('lodash', { token: 'i-am-the-worst'})
//
// `lodash` has now been unpublished, along with all its versions
11.0.0 (2024-12-16)
5319e48
#7973 remove unnecessary sprintf-js files in node_modules (#7973)d369c77
#7976 socks-proxy-agent@8.0.5
3b2951a
#7976 https-proxy-agent@7.0.6
a598b7b
#7976 agent-base@7.1.3
52bcaf6
#7976 debug@4.4.0
aabf345
#7976 p-map@7.0.3
28e8761
#7976 npm-package-arg@12.0.1
ecd7190
#7976 dev dependency updates (@wraithgar)a07f4e0
#7976 @npmcli/template-oss@4.23.6
(@wraithgar)687ab12
#7970 remove pre-release mode from npm 11 and workspaces (#7970) (@wraithgar)@npmcli/arborist@9.0.0
@npmcli/config@10.0.0
libnpmaccess@10.0.0
libnpmdiff@8.0.0
libnpmexec@10.0.0
libnpmfund@7.0.0
libnpmorg@8.0.0
libnpmpack@9.0.0
libnpmpublish@11.0.0
libnpmsearch@9.0.0
libnpmteam@8.0.0
libnpmversion@8.0.0
FAQs
Programmatic API for the bits behind npm publish and unpublish
The npm package libnpmpublish receives a total of 651,050 weekly downloads. As such, libnpmpublish popularity was classified as popular.
We found that libnpmpublish demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.