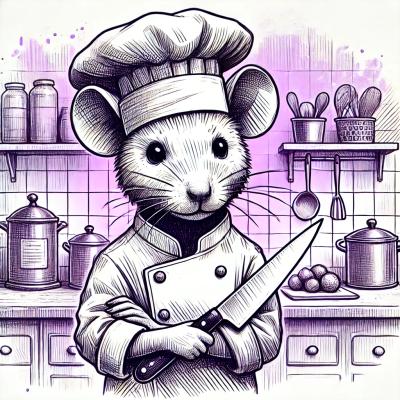
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Full Version | Homepage
Lists is a library of JavaScript higher-order functions for working with arrays and strings. The library was inspired by Haskell's Data.List module by the powerful people over at The University of Glasgow. You can view the original source here. You could then scroll through each function closely and see a purposefully similar correlation between the Haskell implementation and this implementation.
Pass functions to functions to functions to solve complex problems. Most of the functions featured in Lists produce new arrays, to reinforce the paradigm of stateless programming.
Disclaimer: Almost all of these functions are naive recursive definitions. The idea behind this library is to maximize problem-solving expressivity. This library provides an alternative toolbox by which to solve problems. This library should be sufficient for most projects but remember to monitor your heap memory space in the case of excessive function calls.
##Install
##Components
#Contents
Legend - arr | [] : Array, obj | {} : Object, str : String, num : Number, f : Function, x : variable;
A{ 1. } B{ tail } C{ arr|str -> [x] }
map : [x] -> f -> [x]
Basic Functions
append
: arr|str -> arr|str|num -> [x]|strhead
: arr|str -> xlast
: arr|str -> xinit
: arr|str -> [x]tail
: arr|str -> [x]nil
: arr|str -> booleanList Transformations
map
: [x] -> f -> [x]rev
: [x] -> [x]intersperse
: x -> [x] -> arr|strintercalate
: [x] -> [[x]] -> [x]transpose
: [[x]] -> [[x]]subsequences
: [x] -> [[x]]permutations
: [x]|str -> [[x]]|[str]Reducing Lists (folds)
foldl
: x -> [x]|str -> f -> xfoldl1
: [x]|str -> f -> xfoldr
: x -> [x]|str -> f -> xfoldr1
: [x]|str -> f -> xconcatMap
: [x]|str -> f -> [x]|strand
: [boolean] -> booleanor
: [boolean] -> booleanany
: [x]|str -> f -> booleanall
: [x]|str -> f -> booleansum
: [num] -> numproduct
: [num] -> nummaximum
: [num] -> numminimum
: [num] -> nummaxList
: [[x]] -> [x]minList
: [[x]] -> [x]Building Lists
scanl
: x -> [x]|str -> f -> [x]scanr
: x -> [x]|str -> f -> [x]mapAccumL
: x -> [x]|str -> f -> [x, [x]]mapAccumR
: x -> [x]|str -> f -> [x, [x]]iterate
: x -> num -> f -> [x]replicate
: x -> num -> [x]cycle
: [x]|str -> num -> [x]|strunfold
: f -> f -> f -> x -> [x]|strSublists
take
: num -> [x]|str -> [x]drop
: num -> [x]|str -> [x]splitAt
: num -> [x]|str -> [[x],[x]]takeWhile
: f -> [x]|str -> [x]dropWhile
: f -> [x]|str -> [x]span
: arr|str -> f -> [[x], [x]|str]break
: arr|str -> f -> [[x], [x]|str]stripPrefix
: [num]|str -> [num]|str -> [num]|[str]Searching Lists
Indexing Lists
Zipping and Unzipping Lists
Special Lists
Generalized Functions
Extra Functional Utilities
Signature Definition: Give arg 1 an Array or a String; Give arg 2 an Array or a String or a Number; Get an Array of variables or String.
Example Usage:
lists.append([1],[2]); /* [1,2] */
lists.append([1],2); /* [1,2] */
lists.append('a','b'); /* 'ab' */
Signature Definition: Give arg 1 an Array or a String; Get a variable.
Example Usage:
lists.head([1,2]); /* 1 */
lists.head([[1,2],[3,4]]); /* [1,2] */
lists.head('ab'); /* 'a' */
Signature Definition: Give arg 1 an Array or a String; Get a variable.
Example Usage:
lists.last([1,2]); /* 2 */
lists.last([[1,2],[3,4]]); /* [3,4] */
lists.last('ab'); /* 'b' */
Signature Definition: Give arg 1 an Array or a String; Get an Array of variables.
Example Usage:
lists.init([1,2,3]); /* [1,2] */
lists.init([[1,2],[3,4],[5,6]]); /* [[1,2],[3,4]] */
lists.init('abc'); /* ['a','b'] */
Signature Definition: Give arg 1 an Array or a String; Get an Array of variables.
Example Usage:
lists.tail([1,2,3]); /* [2,3] */
lists.tail([[1,2],[3,4],[5,6]]); /* [[3,4],[5,6]] */
lists.tail('abc'); /* ['b','c'] */
Signature Definition: Give arg 1 an Array or a String; Get a boolean.
Example Usage:
lists.nil(null); /* true */
lists.nil([]); /* true */
lists.nil(''); /* true */
lists.nil('a'); /* false */
lists.nil([1]); /* false */
Signature Definition: Give arg 1 an Array or a String; Give arg 2 a Function; Get an Array of variables.
Example Usage:
lists.map([1,2,3], function(x) { return x*2 }); /* [2,4,6] */
lists.map('abc', function(str) { return str.toUpperCase() }); /* ["A","B","C"] */
lists.map([{'S': 1}, {'u': 2}, {'p': 3}], function(obj) {
return lists.flatten(lists.keys(obj))
}); /* ["S", "u", "p"] */
Signature Definition: Give arg 1 an Array or a String; Get an Array of variables.
Example Usage:
lists.rev([1,2,3]); /* [3,2,1] */
lists.rev('abc'); /* ['c','b','a'] */
lists.rev([[2],[3]]) /* [[3],[2]] */
Signature Definition: Give arg 1 a variable; Give arg 2 an Array of variables; Get an Array of variables.
Example Usage:
lists.intersperse(1,[5,5,5]); /* [5,1,5,1,5] */
lists.intersperse([1,2],[[6],[6]]); /* [[6],[1,2],[6]] */
lists.intersperse('b','ac'); /* 'abc' */
lists.intersperse({b:2},[{a:1},{b:3}]); /* [{a:1},{b:2},{c:3}] */
Signature Definition: Give arg 1 an Array of variables; Give arg 2 an Array of Arrays of variables; Get an Array of variables.
Example Usage:
lists.intercalate([1],[[5],[5],[5]]); /* = lists.flatten(lists.intersperse([1],[[5],[5],[5]])) // [5,1,5,1,5] */
lists.intercalate(["abc"],[["efg"],["qrs"]]) /* ["efg", "abc", "qrs"] */
lists.intercalate([{a:1}],[[{b:1}],[{c:2}]]); /* [{b:1},{a:1},{c:2}] */
Signature Definition: Give arg 1 an Array of Arrays of variables; Get an Array of Arrays of variables.
Example Usage:
lists.transpose([[1,2,4],[3,4,4]]) /* [[1,3],[2,4],[4,4]] */
lists.transpose([["a","b","c"],["a","b","c"]]) /* [["a","a"],["b","b"],["c","c"]] */
Signature Definition: Give arg 1 an Array of variables; Get an Array of Arrays of variables.
Example Usage:
lists.subsequences('ab') /* [[],['a'],['b'],['ab']] */
lists.subsequences([1,2,3]) /* [[],[1],[2],[1,2],[3],[1,3],[2,3],[1,2,3]] */
Signature Definition: Give arg 1 an Array of variables or a String; Get an Array of Arrays of variables or an Array of Strings respectively.
Example Usage:
lists.permutations('abc') /* ["abc","acb","bac","bca","cab","cba"] */
lists.permutations([1,2,3]) /* [[1,2,3],[1,3,2],[2,1,3],[2,3,1],[3,1,2],[3,2,1]] */
Signature Definition: Give arg 1 a starting variable (usually a left identity of the binary operator); Give arg 2 an Array of variables or a String; Give arg 3 a function (binary operator); Get a variable.
Example Usage:
reverse = lists.foldl('','abc',function(x,y){ return y.concat(x); }); /* "cba" */
sum = lists.foldl(0,[1,2,3],function(x,y){ return x+y; }); /* 6 */
lists.foldl([], [[1,2],[3,4]], function(x,y) {return x.concat(y) }) /* [1,2,3,4] */
Signature Definition: Give arg 1 an Array of variables or a String; Give arg 2 a function (binary operator); Get a variable.
Example Usage:
lists.foldl1('abc',function(x,y){ return x.concat(y).toUpperCase() }) /* "ABC" */
lists.foldl1([1,2,3],function(x,y){ return x+y }) /* 6 */
Signature Definition: Give arg 1 a starting variable (usually a right identity of the binary operator); Give arg 2 an Array of variables or a String; Give arg 3 a function (binary operator); Get a variable.
Example Usage:
lists.foldr(0,[1,2,3,4],function(x,y){ return x-y; }) /* -2 */
lists.foldr([],[[1,2],[3,4],[5,6]],function(x,y){
return lists.rev(x).concat(y);
}); /* [4,3,1,2,5,6] */
Signature Definition: Give arg 1 an Array of variables or a String; Give arg 2 a function (binary operator); Get a variable.
Example Usage:
lists.foldr1([1,2,3],function(x,y){ return x - y }) /* 3 */
lists.foldr1('aabbcc',function(x,y){ return x=='a'? x=y : x.concat(y)}) /* "bbcc" */
Signature Definition: Give arg 1 an Array of Arrays of variables or a String; Get an Array of variables or a String
Example Usage:
lists.flatten(['abc']); /* 'abc' */
lists.flatten([[1,2],[3,4]]) /* [1,2,3,4] */
Signature Definition: Give arg 1 an Array of variables or a String; Give arg 2 a function that produces an Array of variables or String; Get an Array of variables or a String
Example Usage:
lists.concatMap(['bang','bang'], function(x){ return x+'!'}) /* "bang!bang!" */
lists.concatMap([1,2,3],function(x){ return [[x*2,x/2]] }) /* [[2,0.5],[4,1],[6,1.5]] */
lists.concatMap([{a:1},{b:2}], function(x){
x.prop = 'hi';
return [x]
}); /* [{a:1,prop:"hi"},{b:2,prop:"hi"}]*/
Signature Definition: Give arg 1 an Array of booleans. Get a boolean.
Example Usage:
lists.and([5>1,5>2,5>3]) /* true */
lists.and([5>1,false,5>3]) /* false */
Signature Definition: Give arg 1 an Array of booleans. Get a boolean.
Example Usage:
lists.or([5<1,5<2,5>3]) /* true */
lists.or([5<1,5<2,5<3]) /* false */
Signature Definition: Give arg 1 an Array of variables or String; Give arg 2 a predicate function to be applied to each element of arg 1; Get a boolean.
Example Usage:
lists.any([1,2,3],function(x) { return x < .5}) /* false */
lists.any('abc',function(x) { return x == 'b'}) /* true */
Signature Definition: Give arg 1 an Array of variables or String; Give arg 2 a predicate function to be applied to each element of arg 1; Get a boolean.
Example Usage:
lists.all('abc', function(x){ return x==x.toUpperCase() }) /* false */
lists.all([2,4], function(x) { return x > 3 }) /* false */
lists.all([2,4], function(x) { return x%2==0 }) /* true */
Signature Definition: Give arg 1 an Array of Numbers; Get a number.
Example Usage:
lists.sum([2,4,6]) /* 12 */
lists.sum([.2,.4,.6]) /* 1.2000000000000002 */
Signature Definition: Give arg 1 an Array of Numbers; Get a number.
Example Usage:
lists.product([2,4,6]) /* 48 */
lists.product([.2,.4,.6]) /* 0.04800000000000001 */
Signature Definition: Give arg 1 an Array of Numbers; Get a number.
Example Usage:
lists.maximum([2,4,6]) /* 6 */
lists.maximum([.2,.4,.6]) /* .6 */
Signature Definition: Give arg 1 an Array of Numbers; Get a number.
Example Usage:
lists.minimum([2,4,6]) /* 2 */
lists.minimum([.2,.4,.6]) /* .2 */
Signature Definition: Give arg 1 an Array of Arrays of variables; Get an Array of variables.
Example Usage:
lists.maxList([[1],[2,3]]) /* [2,3] */
lists.maxList([[1,2],[3]]) /* [1,2] */
Signature Definition: Give arg 1 an Array of Arrays of variables; Get an Array of variables.
Example Usage:
lists.minList([[],[1]]) /* [] */
lists.minList([[1,2],[3]]) /* [3] */
Signature Definition: Give arg 1 a starting variable; Give arg 2 an Array of variables or a String; Give arg 3 a function (binary operator); Get an Array of variables.
Example Usage:
lists.scanl('.','abc',function(x,y){return x + y}) /* [".",".a",".ab",".abc"] */
lists.scanl(0,[1,2,3],function(x,y){return x + y}) /* [0,1,3,6] */
Signature Definition: Give arg 1 a starting variable; Give arg 2 an Array of variables or a String; Give arg 3 a function (binary operator); Get an Array of variables.
Example Usage:
lists.scanr('.','abc',function(x,y){return x + y}) /* ["abc.","bc.","c.","."] */
lists.scanr(0,[1,2,3],function(x,y){return x + y}) /* [6,5,3,0] */
Signature Definition: Give arg 1 a starting variable (accumulator); Give arg 2 an Array of variables or a String; Give arg 3 a function; Get an Array of variable followed by Array of variables.
Example Usage:
lists.mapAccumL(5, [2,4,8], function(x,y){ return [x+y,x*y]}) /* [19, [10,28,88]]*/
lists.mapAccumL(5, [2,4,8], function(x,y){ return [y,y]}) /* [8, [2,4,8]] */
lists.mapAccumL(5, [5,5,5], function(x,y){ return [x,x]}) /* [5, [5,5,5]] */
Signature Definition: Give arg 1 a starting variable (accumulator); Give arg 2 an Array of variables or a String; Give arg 3 a function; Get an Array of variable followed by Array of variables.
Example Usage:
lists.mapAccumR(5, [2,4,8], function(x,y){ return [x+y,x*y]}) /* [19, [34,52,40]]*/
lists.mapAccumR(5, [2,4,8], function(x,y){ return [y,y]}) /* [2, [2,4,8]] */
lists.mapAccumR(5, [5,5,5], function(x,y){ return [x,x]}) /* [5, [5,5,5]] */
Signature Definition: Give arg 1 a variable; Give arg 2 a number; Give arg 3 a function; Get an Array of variables.
Example Usage:
lists.iterate('a',3,function(ch){return ch+'b'}) /* ["a","ab","abb"] */
lists.iterate([1,2],3,function(xs){return lists.intersperse(6,xs)}) /* [[1,2],[1,6,2],[1,6,6,6,2]] */
lists.iterate(2,4,function(x){ return x*x }) /* [2,4,16,256] */
Signature Definition: Give arg 1 a variable; Give arg 2 a number; Get an Array of variables.
Example Usage:
lists.replicate(5,5) /* [5,5,5,5,5] */
lists.replicate([1,2],2) /* [[1,2],[1,2]] */
lists.replicate({a:1},2) /* [{a:1},{a:2}] */
Signature Definition: Give arg 1 an Array of variables; Give arg 2 a number; Get an Array of variables.
Example Usage:
lists.cycle('abc',3) /* "abcabcabc" */
lists.cycle([1,2],2) /* [1,2,1,2] */
Signature Definition: Give arg 1 an function (predicate); Give arg 2 a function; Give arg 3 a function; Give arg 4 a variable (seed).
Example Usage:
function chop8(xs){
return l.unfold(l.nil,l.part(l.take,8,_),l.part(l.drop,8,_),xs)
}
chop8([1,2,3,4,5,6,7,8,9]) /* [[1,2,3,4,5,6,7,8],[9]] */
function unfoldMap(xs,f) {
return lists.unfold(
lists.nil,
lists.part(lists.pipe(f,lists.head),_),
lists.part(lists.tail,_),
xs)
}
unfoldMap([1,2],function(x){ return x * 2 }) /* [2,4] */
Signature Definition: Give arg 1 a number; Give arg 2 an Array of variables or String; Get an Array of variables.
Example Usage:
lists.take(2,[1,2,3]) /* [1,2] */
lists.take(2,'abc') /* ["a","b"] */
Signature Definition: Give arg 1 a number; Give arg 2 an Array of variables or String; Get an Array of variables.
Example Usage:
lists.drop(2,[1,2,3]) /* [3] */
lists.drop(2,'abc') /* ["c"] */
Signature Definition: Give arg 1 a number; Give arg 2 an Array of variables or String; Get an Array of two Arrays of variables.
Example Usage:
lists.splitAt(2,'abc') /* [['a','b'],['c']] */
lists.splitAt(2,[1,2,3]) /* [[1,2],[3]] */
Signature Definition: Give arg 1 a function; Give arg 2 an Array of variables or String; Get an Array of variables.
Example Usage:
lists.takeWhile([1,2,3,1], function(x){ return x < 3 }) /* [1,2] */
lists.takeWhile([1], function(x){ return x < 2 }) /* [1] */
lists.takeWhile('aabc', function(x){ return x =='a' }) /* ["a","a"] */
Signature Definition: Give arg 1 a function; Give arg 2 an Array of variables or String; Get an Array of variables.
Example Usage:
lists.dropWhile([1,2,3,4], function(x){ return x < 3 }) /* [3,4] */
lists.dropWhile([1], function(x){ return x < 2 }) /* [2] */
lists.dropWhile('aabc', function(x){ return x =='a' }) /* ["b","c"] */
Signature Definition: Give arg 1 an Array of variables or String; Give arg 2 a function; Get an Array of Array of variables and Array of variables or String (psuedo-tuple).
Example Usage:
lists.span([1,2,3,4], function(x){return x < 3}) /* [[1,2],[3,4]] */
lists.span("abcde", function(x){return x == "a"}) /* [["a"],["b","c","d","e"]] */
lists.span([{a:2},{a:2},{b:2}], function(x){return x.a==2}) /* [[{a:2},{a:2}],[{b:2}]] */
Signature Definition: Give arg 1 an Array of variables or String; Give arg 2 a function; Get an Array of Array of variables and Array of variables or String (psuedo-tuple).
Example Usage:
lists.break([1,2,3,4], function(x){return x < 3}) /* [[],[1,2,3,4]] */
lists.break("abcde", function(x){return x == "a"}) /* [[],"abcde"] */
lists.break([{a:2},{a:2},{b:2}], function(x){return x.a==2}) /* [[],[{a:2},{a:2},{b:2}]] */
Signature Definition: Give arg 1 an Array of Numbers or String; Give arg 2 an Array of Numbers or String; Get an Array of Numbers or an Array of Strings.
Example Usage:
lists.stripPrefix("abc","abcabce") /* ["a","b","c","e"] */
lists.stripPrefix([1,2],[1,2,3,4]) /* [3,4] */
FAQs
A library of higher-order functions modeled after Haskell's Data.List module
The npm package lists receives a total of 11 weekly downloads. As such, lists popularity was classified as not popular.
We found that lists demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.