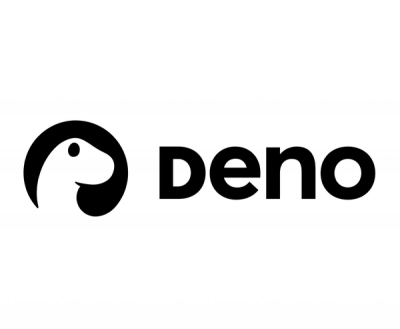
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
lit-element
Advanced tools
The lit-element package is a simple base class for creating fast, lightweight web components with the Lit library. It provides a declarative template system that ties your markup to your component's properties and state, along with reactive updates and a component lifecycle.
Declarative Templates
LitElement uses `html` tagged template literals to define templates that are bound to the component's properties. When properties change, the template is efficiently re-rendered.
import { LitElement, html } from 'lit-element';
class MyElement extends LitElement {
static get properties() {
return {
message: { type: String }
};
}
constructor() {
super();
this.message = 'Hello, World!';
}
render() {
return html`<p>${this.message}</p>`;
}
}
customElements.define('my-element', MyElement);
Reactive Properties
Properties can be made reactive using the `@property` decorator. When a reactive property changes, LitElement automatically updates the component's template.
import { LitElement, html, property } from 'lit-element';
class MyElement extends LitElement {
@property({ type: String }) greeting = 'Hello';
render() {
return html`<h1>${this.greeting}, World!</h1>`;
}
}
customElements.define('my-element', MyElement);
Lifecycle Methods
LitElement provides lifecycle methods such as `firstUpdated`, `updated`, and `disconnectedCallback` for managing the component's lifecycle events.
import { LitElement } from 'lit-element';
class MyElement extends LitElement {
firstUpdated(changedProperties) {
console.log('Component first updated!');
}
updated(changedProperties) {
console.log('Component updated with changed properties:', changedProperties);
}
disconnectedCallback() {
console.log('Component removed from the DOM!');
}
}
customElements.define('my-element', MyElement);
React is a popular library for building user interfaces. It uses a virtual DOM for efficient updates, which is different from LitElement's direct DOM manipulation. React components are typically more verbose and use JSX for templating.
Vue is a progressive framework for building UIs. Like LitElement, it offers a reactive and composable data model. Vue uses a virtual DOM similar to React and has a more opinionated structure, including a focus on single-file components.
Svelte is a compiler that generates efficient JavaScript code for creating web components. Unlike LitElement, which updates the DOM in response to property changes, Svelte compiles components to update the DOM directly, which can result in better performance for some applications.
Stencil is a compiler that generates web components with a focus on performance and compatibility. It provides a set of features similar to LitElement but includes a virtual DOM and TypeScript support out of the box.
This is a major version pre-release of LitElement 3.0. See issue #1077 for the full list of changes planned/considered for this release.
This pre-release is not yet feature complete or API stable. Please note the breaking changes, known issues, and limitations below, and use at your risk until the stable release is available. Issues are welcome for unexpected changes not noted below or in the changelog.
While LitElement
3.0 is intended to be a mostly backward-compatible change for the
majority of 2.x users, please be aware of the following notable breaking
changes:
LitElement
pre-release uses the lit-html
pre-release as well.
Please see the lit-html
pre-release README and
changelog for information on
any breaking changes to lit-html
features in your components.lit-element
module.
Instead, import any decorators you use from lit-element/decorators/*
.requestUpdate()
no longer returns a Promise. Instead await the
updateComplete
Promise.See the full changelog for more details on these and other minor breaking changes.
lit-html
are still in progress.
Please refer to the pre-release README for a list of
known lit-html
issues.A simple base class for creating fast, lightweight web components with lit-html.
Full documentation is available at lit-element.polymer-project.org.
LitElement uses lit-html to render into the
element's Shadow DOM
and adds API to help manage element properties and attributes. LitElement reacts to changes in properties
and renders declaratively using lit-html
. See the lit-html guide
for additional information on how to create templates for lit-element.
import {LitElement, html, css} from 'lit-element';
import {customElement, property} from 'lit-element/decorators.js';
// This decorator defines the element.
@customElement('my-element')
export class MyElement extends LitElement {
// This decorator creates a property accessor that triggers rendering and
// an observed attribute.
@property()
mood = 'great';
static styles = css`
span {
color: green;
}
`;
// Render element DOM by returning a `lit-html` template.
render() {
return html`Web Components are <span>${this.mood}</span>!`;
}
}
<my-element mood="awesome"></my-element>
Note, this example uses decorators to create properties. Decorators are a proposed standard currently available in TypeScript or Babel. LitElement also supports a vanilla JavaScript method of declaring reactive properties.
Runs in browsers with JavaScript Modules: Stackblitz, JSFiddle, JSBin, CodePen.
You can also copy this HTML file into a local file and run it in any browser that supports JavaScript Modules.
From inside your project folder, run:
$ npm install lit-element
To install the web components polyfills needed for older browsers:
$ npm i -D @webcomponents/webcomponentsjs
lit-element includes a development mode which adds additional checks that are reported in the console.
To enable development mode, add the development
exports condition to your node
resolve configuration.
NOTE: Requires rollup#540
{
nodeResolve: {
exportConditions: ['development'];
}
}
NOTE: Requires rollup#540
{
plugins: [
nodeResolve({
exportConditions: ['development'],
}),
];
}
NOTE: Requires Webpack v5
{
resolve: {
conditionNames: ['development'];
}
}
The last 2 versions of all modern browsers are supported, including Chrome, Safari, Opera, Firefox, Edge. In addition, Internet Explorer 11 is also supported.
Edge and Internet Explorer 11 require the web components polyfills.
Please see CONTRIBUTING.md.
FAQs
A simple base class for creating fast, lightweight web components
The npm package lit-element receives a total of 1,552,317 weekly downloads. As such, lit-element popularity was classified as popular.
We found that lit-element demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 13 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.