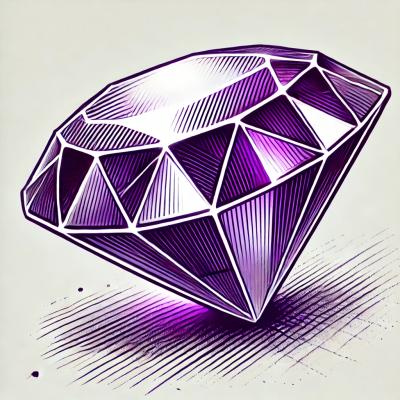
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
little-state-machine
Advanced tools
The little-state-machine npm package is a lightweight state management library for React applications. It is designed to be simple and easy to use, making it ideal for small to medium-sized projects where you need to manage state without the overhead of more complex solutions.
State Initialization
This feature allows you to initialize the state of your application. The `createStore` function is used to create a store with an initial state.
import { createStore } from 'little-state-machine';
const initialState = {
user: {
name: '',
age: 0
}
};
const store = createStore(initialState);
State Update
This feature allows you to update the state. The `useStateMachine` hook is used to access actions that can update the state. In this example, the `updateUser` action updates the user information in the state.
import { useStateMachine } from 'little-state-machine';
const updateUser = (state, payload) => ({
...state,
user: {
...state.user,
...payload
}
});
const Component = () => {
const { actions } = useStateMachine({ updateUser });
const handleUpdate = () => {
actions.updateUser({ name: 'John', age: 30 });
};
return <button onClick={handleUpdate}>Update User</button>;
};
State Access
This feature allows you to access the current state. The `useStateMachine` hook is used to access the state, which can then be used in your components.
import { useStateMachine } from 'little-state-machine';
const Component = () => {
const { state } = useStateMachine();
return (
<div>
<p>Name: {state.user.name}</p>
<p>Age: {state.user.age}</p>
</div>
);
};
Redux is a popular state management library for JavaScript applications. It is more complex and feature-rich compared to little-state-machine, making it suitable for larger applications with more complex state management needs.
MobX is a state management library that makes state management simple and scalable by transparently applying functional reactive programming. It is more flexible and powerful than little-state-machine, but also comes with a steeper learning curve.
Zustand is a small, fast, and scalable state management solution for React. It is similar to little-state-machine in terms of simplicity and ease of use, but offers more features and better performance.
sessionStorage
or localStorage
)$ npm install little-state-machine
StateMachineProvider
This is a Provider Component to wrapper around your entire app in order to create context.
createStore
createStore(store, options?: {
name: string; // rename the store
middleWares?: Function[]; // function to invoke each action
syncStores?: // sync with external store in your session/local storage
| Record<string, string[]>
| { externalStoreName: string; transform: Function } // name of the external store, and state to sync
| { externalStoreName: string; transform: Function }[];
}})
Function to initialize the global store, invoked at your app root (where <StateMachineProvider />
lives).
import yourDetail from './state/yourDetail';
function log(store) {
console.log(store);
}
createStore(
{
yourDetail, // it's an object of your state { firstName: '', lastName: '' }
},
{
middleWares: [log], // an array of middleWares, which gets run each actions
syncStores: {
// you can sync with external store and transform the data
externalStoreName: 'externalStoreName',
transform: ({ externalStoreData, currentStoreData }) => {
return { ...externalStoreData, ...currentStoreData };
},
},
// alternative you can just specify the store name and root state name { yourDetails: { firstName: '' } }
// syncStores : {
// externalStoreName: ['yourDetail'],
// }
// or you can pass in an array of transform function
// syncStores : [
// {
// externalStoreName: 'externalStoreName',
// transform: ({ externalStoreData, currentStoreData }) => {
// return { ...externalStoreData, ...currentStoreData };
// },
// }
// ]
},
);
useStateMachine
This hook function will return action/actions and state of the app.
import { updateUserNameAction, removeNameAction } from './actions/yourDetails';
const { action, state } = useStateMachine(updateUserNameAction);
const { actions, state } = useStateMachine({
removeNameAction,
updateUserNameAction,
});
// The following examples are for optional argument
const { actions, state } = useStateMachine(
{
removeNameAction,
updateUserNameAction,
},
{
removeNameAction: 'removeName',
updateUserNameAction: 'updateUserName',
},
);
const { action, state } = useStateMachine(updateUserNameAction, {
shouldReRenderApp: false, // This will prevent App from re-render and only update the store
});
📋 app.js
import React from 'react';
import yourDetail from './yourDetail';
import YourComponent from './yourComponent';
import { StateMachineProvider, createStore } from 'little-state-machine';
import { DevTool } from 'little-state-machine-devtools';
// The following code is for React Native usage
// import { AsyncStorage } from "react-native";
// setStorageType(AsyncStorage);
// create your store
createStore({
yourDetail,
});
export default () => {
return (
<StateMachineProvider>
<DevTool />
<YourComponent />
</StateMachineProvider>
);
};
📋 yourComponent.js
import React from 'react';
import { updateName } from './action.js';
import { useStateMachine } from 'little-state-machine';
export default function YourComponent() {
const {
action,
state: {
yourDetail: { name },
},
} = useStateMachine(updateName);
return <div onClick={() => action({ name: 'bill' })}>{name}</div>;
}
📋 yourDetail.js
export default {
name: 'test',
};
📋 action.js
export function updateName(state, payload) {
return {
...state,
yourDetail: {
...state.yourDetail,
...payload,
},
};
}
DevTool component to track your state change and action.
import { DevTool } from 'little-state-machine-devtools';
<StateMachineProvider>
<DevTool />
</StateMachineProvider>;
For legacy IE11 support, you can import little-state-machine IE11 version.
import { createStore } from 'little-state-machine/dist/little-state-machine.ie11'
Consider adding Object.entries()
polyfill if you're wondering to have support for old browsers.
You can weather consider adding snippet below into your code, ideally before your App.js file:
📋 utils.[js|ts]
if (!Object.entries) {
Object.entries = function( obj ){
var ownProps = Object.keys( obj ),
i = ownProps.length,
resArray = new Array(i); // preallocate the Array
while (i--)
resArray[i] = [ownProps[i], obj[ownProps[i]]];
return resArray;
};
}
Or you can add core-js polyfill into your project and add core-js/es/object/entries
in your polyfills.[js|ts]
file.
FAQs
State management made super simple
The npm package little-state-machine receives a total of 224,334 weekly downloads. As such, little-state-machine popularity was classified as popular.
We found that little-state-machine demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.