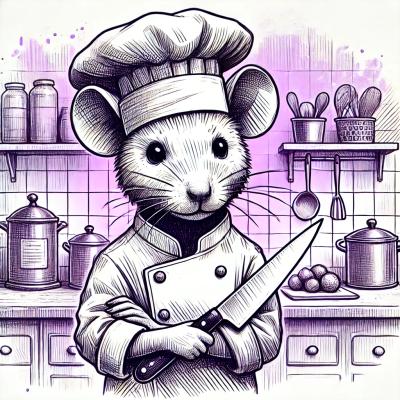
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Create composable extensible styled text in both consoles and browsers.
Install it with NPM or add it to your package.json
:
$ npm install magicpen
Then:
var magicpen = require('magicpen');
var pen = magicpen('ansi');
pen.red('Hello').sp().green('world!');
console.log(pen.toString());
Include magicpen.js
.
<script src="magicpen.js"></script>
this will expose the magicpen
function under the following namespace:
var magicpen = weknowhow.magicpen;
var pen = magicpen('html');
pen.red('Hello').sp().green('world!');
document.getElementById('output').innerHTML = pen.toString();
Include the library with RequireJS the following way:
require.config({
paths: {
magicpen: 'path/to/magicpen'
}
});
define(['magicpen'], function (magicpen) {
var pen = magicpen('html');
pen.red('Hello').sp().green('world!');
document.getElementById('output').innerHTML = pen.toString();
});
You create a new magicpen
instance by calling the magicpen
function. The function takes one parameter, the output mode. By
default the text
mode is console output without colors. You can
also choose the ansi
mode or the html
mode. The ansi
mode will
format the output for the console with colors and basic styling. The
html
mode will format the output in html with colors and basic
styling.
Let's try to create our first magicpen
in ansi
mode:
var pen = magicpen('ansi');
pen.red('Hello').sp().green('world!');
console.log(pen.toString());
The above snippet create a new magicpen
in ansi
mode and writes
Hello in red, space and world! in green and prints the formatted
output to the console. This will produce the following output:

Let's try to create the same output but format it as html:
var pen = magicpen('html');
pen.red('Hello').sp().green('world!');
document.getElementById('output').innerHTML = pen.toString();
You will get the following output it the browser:

Append the given content to the output with the styles specified in the style strings.
Text properties: bold
, dim
, italic
, underline
, inverse
, hidden
, strikeThrough
.
Foreground colors: black
, red
, green
, yellow
, blue
, magenta
, cyan
, white
, gray
.
Background colors: bgBlack
, bgRed
, bgGreen
, bgYellow
, bgBlue
, bgMagenta
, bgCyan
, bgWhite
.
var pen = magicpen('ansi');
pen.text('Hello', 'red')
.text(' ')
.text('colorful', 'yellow, bold')
.text(' ')
.text('world', 'green', 'underline')
.text('!', 'bgYellow, blue');
console.log(pen.toString());

Notice that special characters might get escaped by this method. The example below shows how special html characters is escaped by the html mode.
var pen = magicpen('html');
pen.text('<strong>Hello world!</strong>');
expect(pen.toString(), 'to equal',
'<code>\n' +
' <div><strong>Hello world!</strong></div>\n' +
'</code>');
Starts a new line.
Example:
pen.text('Hello').nl()
.indentLines()
.indent().text('beautiful').nl()
.outdentLines()
.text('world');
expect(pen.toString(), 'to equal',
'Hello\n' +
' beautiful\n' +
'world');
Increments the indentation level.
Decrements the indentation level.
Appends the indentation to the output.
Appends the content of the given pen to the end of this pen.
Notice, that the given pen must be in the same mode as this pen.
Example:
var pen = magicpen();
var otherPen = pen.clone().text('world!');
pen.text('Hello').sp().append(otherPen);
expect(pen.toString(), 'to equal', 'Hello world!');
Appends the content of the given pen to the end of this pen in an inline block.
Notice, that the given pen must be in the same mode as this pen.
Example:
var pen = magicpen();
var otherPen = pen.clone()
.text(' // This is a').nl()
.text(' // multiline block');
pen.text('Text before block').block(otherPen);
expect(pen.toString(), 'to equal',
'Text before block // This is a\n' +
' // multiline block');
Prepends each line of this pen with the content of the given pen.
Notice, that the given pen must be in the same mode as this pen.
Example:
var pen = magicpen();
var otherPen = pen.clone().text('> ');
pen.text('Line').nl()
.text('after line').nl()
.text('after line');
expect(pen.toString(), 'to equal',
'> Line\n' +
'> after line\n' +
'> after line');
Returns a clone of the current pen with an empty output buffer. This operation is very cheap, so don't hesitate to use it when it makes sense.
Defines a new style for the magicpen. The usage is best explained by an example:
var pen = magicpen('ansi');
pen.addStyle('rainbow', function (text, rainbowColors) {
rainbowColors = rainbowColors ||
['gray', 'red', 'green', 'yellow', 'blue', 'magenta', 'cyan'];
for (var i = 0; i < text.length; i += 1) {
var color = rainbowColors[i % rainbowColors.length];
this.text(text[i], color);
}
});
pen.rainbow('The unicorns are flying low today').nl();
.rainbow('The unicorns are flying low today', ['green', 'red', 'cyan']);
console.log(pen.toString());
As you can see in the example above, a custom style can produce any kind of output using an instance of a magicpen.
Alias for text(duplicate(' ', count))
.
Alias for text(content, 'bold')
.
Alias for text(content, 'dim')
.
Alias for text(content, 'italic')
.
Alias for text(content, 'underline')
.
Alias for text(content, 'inverse')
.
Alias for text(content, 'hidden')
.
Alias for text(content, 'strikeThrough')
.
Alias for text(content, 'black')
.
Alias for text(content, 'red')
.
Alias for text(content, 'green')
.
Alias for text(content, 'yellow')
.
Alias for text(content, 'blue')
.
Alias for text(content, 'magenta')
.
Alias for text(content, 'cyan')
.
Alias for text(content, 'white')
.
Alias for text(content, 'gray')
.
Alias for text(content, 'bgBlack')
.
Alias for text(content, 'bgRed')
.
Alias for text(content, 'bgGreen')
.
Alias for text(content, 'bgYellow')
.
Alias for text(content, 'bgBlue')
.
Alias for text(content, 'bgMagenta')
.
Alias for text(content, 'bgCyan')
.
Alias for text(content, 'bgWhite')
.
FAQs
Styled output in both consoles and browsers
The npm package magicpen receives a total of 11,988 weekly downloads. As such, magicpen popularity was classified as popular.
We found that magicpen demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.