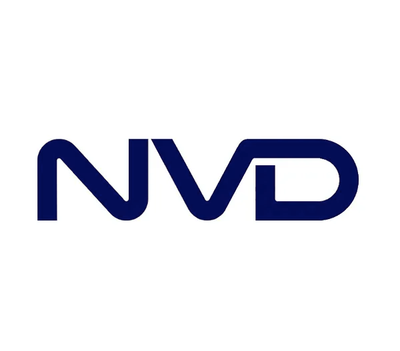
Security News
NVD Backlog Tops 20,000 CVEs Awaiting Analysis as NIST Prepares System Updates
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
mailcomposer
Advanced tools
The mailcomposer npm package is a tool for creating email messages with various features such as attachments, HTML content, and custom headers. It is useful for generating email content that can be sent using an SMTP client or other email transport methods.
Basic Email Composition
This feature allows you to compose a basic email with a sender, receiver, subject, and plain text content.
const MailComposer = require('mailcomposer');
const mail = new MailComposer({
from: 'sender@example.com',
to: 'receiver@example.com',
subject: 'Hello',
text: 'Hello world!'
});
mail.build((err, message) => {
if (err) {
console.error('Error:', err);
} else {
console.log('Email message:', message.toString());
}
});
HTML Email Composition
This feature allows you to compose an email with HTML content, which can include rich text formatting and images.
const MailComposer = require('mailcomposer');
const mail = new MailComposer({
from: 'sender@example.com',
to: 'receiver@example.com',
subject: 'Hello',
html: '<h1>Hello world!</h1>'
});
mail.build((err, message) => {
if (err) {
console.error('Error:', err);
} else {
console.log('Email message:', message.toString());
}
});
Attachments
This feature allows you to add attachments to your email, such as files or images.
const MailComposer = require('mailcomposer');
const mail = new MailComposer({
from: 'sender@example.com',
to: 'receiver@example.com',
subject: 'Hello',
text: 'Hello world!',
attachments: [
{
filename: 'text.txt',
content: 'Hello world!'
}
]
});
mail.build((err, message) => {
if (err) {
console.error('Error:', err);
} else {
console.log('Email message:', message.toString());
}
});
Custom Headers
This feature allows you to add custom headers to your email, which can be useful for various purposes such as tracking or custom processing.
const MailComposer = require('mailcomposer');
const mail = new MailComposer({
from: 'sender@example.com',
to: 'receiver@example.com',
subject: 'Hello',
text: 'Hello world!',
headers: {
'X-Custom-Header': 'Custom header value'
}
});
mail.build((err, message) => {
if (err) {
console.error('Error:', err);
} else {
console.log('Email message:', message.toString());
}
});
Nodemailer is a module for Node.js applications to allow easy email sending. It supports various transport methods, including SMTP, and provides a rich set of features for composing and sending emails. Compared to mailcomposer, Nodemailer is more comprehensive as it includes both email composition and transport functionalities.
EmailJS is a library for sending emails from JavaScript applications. It supports SMTP and provides features for composing and sending emails. While it offers similar functionalities to mailcomposer, it also includes built-in transport methods, making it a more complete solution for email handling.
Sendmail is a simple way to send emails from your Node.js application without needing a dedicated SMTP server. It is lightweight and easy to use, but it lacks some of the advanced composition features provided by mailcomposer.
mailcomposer is a Node.JS module for generating e-mail messages that can be streamed to SMTP or file.
This is a standalone module that only generates raw e-mail source, you need to write your own or use an existing transport mechanism (SMTP client, Amazon SES, SendGrid etc). mailcomposer frees you from the tedious task of generating rfc822 compatible messages.
mailcomposer supports:
Install through NPM
npm install mailcomposer
var MailComposer = require("mailcomposer").MailComposer;
MailComposer
instancevar mailcomposer = new MailComposer([options]);
Where options
is an optional options object with the following possible properties:
"quoted-printable"
)"utf-8"
)Bcc:
field in the message headers. Useful for sendmail command.The following example generates a simple e-mail message with plaintext and html body.
var MailComposer = require("mailcomposer").MailComposer;
mailcomposer = new MailComposer(),
fs = require("fs");
// add additional header field
mailcomposer.addHeader("x-mailer", "Nodemailer 1.0");
// setup message data
mailcomposer.setMessageOption({
from: "andris@tr.ee",
to: "andris@node.ee",
body: "Hello world!",
html: "<b>Hello world!</b>"
});
mailcomposer.streamMessage();
// pipe the output to a file
mailcomposer.pipe(fs.createWriteStream("test.eml"));
The output for such a script (the contents for "test.eml") would look like:
MIME-Version: 1.0
X-Mailer: Nodemailer 1.0
From: andris@tr.ee
To: andris@node.ee
Content-Type: multipart/alternative;
boundary="----mailcomposer-?=_1-1328088797399"
------mailcomposer-?=_1-1328088797399
Content-Type: text/plain; charset=utf-8
Content-Transfer-Encoding: quoted-printable
Hello world!
------mailcomposer-?=_1-1328088797399
Content-Type: text/html; charset=utf-8
Content-Transfer-Encoding: quoted-printable
<b>Hello world!</b>
------mailcomposer-?=_1-1328088797399--
Headers can be added with mailcomposer.addHeader(key, value)
var mailcomposer = new MailComposer();
mailcomposer.addHeader("x-mailer", "Nodemailer 1.0");
If you add an header value with the same key several times, all of the values will be used in the generated header. For example:
mailcomposer.addHeader("x-mailer", "Nodemailer 1.0");
mailcomposer.addHeader("x-mailer", "Nodemailer 2.0");
Will be generated into
...
X-Mailer: Nodemailer 1.0
X-Mailer: Nodemailer 2.0
...
The contents of the field value is not edited in any way (except for the folding), so if you want to use unicode symbols you need to escape these to mime words by yourself. Exception being object values - in this case the object is automatically JSONized and mime encoded.
// using objects as header values is allowed (will be converted to JSON)
var apiOptions = {};
apiOptions.category = "newuser";
apiOptions.tags = ["user", "web"];
mailcomposer.addHeader("X-SMTPAPI", apiOptions)
You can set message sender, receiver, subject line, message body etc. with
mailcomposer.setMessageOption(options)
where options is an object with the
data to be set. This function overwrites any previously set values with the
same key
The following example creates a simple e-mail with sender being andris@tr.ee
,
receiver andris@node.ee
and plaintext part of the message as Hello world!
:
mailcomposer.setMessageOption({
from: "andris@tr.ee",
to: "andris@node.ee",
body: "Hello world!"
});
Possible options that can be used are (all fields accept unicode):
sender
) - the sender of the message. If several addresses are given, only the first one will be usedTo:
fieldCc:
fieldBcc:
fieldreply_to
) - e-mail address for the Reply-To:
fieldtext
) - the plaintext part of the messageThis method can be called several times
mailcomposer.setMessageOption({from: "andris@tr.ee"});
mailcomposer.setMessageOption({to: "andris@node.ee"});
mailcomposer.setMessageOption({body: "Hello world!"});
Trying to set the same key several times will yield in overwrite
mailcomposer.setMessageOption({body: "Hello world!"});
mailcomposer.setMessageOption({body: "Hello world?"});
// body contents will be "Hello world?"
All e-mail address fields take structured e-mail lists (comma separated) as the input. Unicode is allowed for all the parts (receiver name, e-mail username and domain) of the address. If the domain part contains unicode symbols, it is automatically converted into punycode, user part will be converted into UTF-8 mime word.
E-mail addresses can be a plain e-mail addresses
username@example.com
or with a formatted name
'Ноде Майлер' <username@example.com>
Or in case of comma separated lists, the formatting can be mixed
username@example.com, 'Ноде Майлер' <username@example.com>, "Name, User" <username@example.com>
SMTP envelope is usually auto generated from from
, to
, cc
and bcc
fields but
if for some reason you want to specify it yourself, you can do it with envelope
property.
envelope
is an object with the following params: from
, to
, cc
and bcc
just like
with regular mail options. You can also use the regular address format.
mailOptions = {
...,
from: "mailer@node.ee",
to: "daemon@node.ee",
envelope: {
from: "Daemon <deamon@node.ee>",
to: "mailer@node.ee, Mailer <mailer2@node.ee>"
}
}
Attachments can be added with mailcomposer.addAttachment(attachment)
where
attachment
is an object with attachment (meta)data with the following possible
properties:
filename
) - filename to be reported as the name of the attached file, use of unicode is allowedfileName
propertyOne of contents
, filePath
or streamSource
must be specified, if none is
present, the attachment will be discarded. Other fields are optional.
Attachments can be added as many as you want.
Using embedded images in HTML
Attachments can be used as embedded images in the HTML body. To use this
feature, you need to set additional property of the attachment - cid
(unique identifier of the file) which is a reference to the attachment file.
The same cid
value must be used as the image URL in HTML (using cid:
as
the URL protocol, see example below).
NB! the cid value should be as unique as possible!
var cid_value = Date.now() + '.image.jpg';
var html = 'Embedded image: <img src="cid:' + cid_value + '" />';
var attachment = {
fileName: "image.png",
filePath: "/static/images/image.png",
cid: cid_value
};
Automatic embedding images
If you want to convert images in the HTML to embedded images automatically, you can
set mailcomposer option forceEmbeddedImages
to true. In this case all images in
the HTML that are either using an absolute URL (http://...) or absolute file path
(/path/to/file) are replaced with embedded attachments.
For example when using this code
var mailcomposer = new MailComposer({forceEmbeddedImages: true});
mailcomposer.setMessageOption({
html: 'Embedded image: <img src="http://example.com/image.png">'
});
The image linked is fetched and added automatically as an attachment and the url
in the HTML is replaced automatically with a proper cid:
string.
mailcomposer supports DKIM signing with very simple setup. Use this with caution
though since the generated message needs to be buffered entirely before it can be
signed - in this case the streaming capability offered by mailcomposer is illusionary,
there will only be one 'data'
event with the entire message. Not a big deal with
small messages but might consume a lot of RAM when using larger attachments.
Set up the DKIM signing with useDKIM
method:
mailcomposer.useDKIM(dkimOptions)
Where dkimOptions
includes necessary options for signing
zzz
is the selectorNB! Currently if several header fields with the same name exists, only the last one (the one in the bottom) is signed.
Example:
mailcomposer.setMessageOption({from: "andris@tr.ee"});
mailcomposer.setMessageOption({to: "andris@node.ee"});
mailcomposer.setMessageOption({body: "Hello world!"});
mailcomposer.useDKIM({
domainName: "node.ee",
keySelector: "dkim",
privateKey: fs.readFileSync("private_key.pem")
});
When the message data is setup, streaming can be started. After this it is not possible to add headers, attachments or change body contents.
mailcomposer.streamMessage();
This generates 'data'
events for the message headers and body and final 'end'
event.
As MailComposer
objects are Stream instances, these can be piped
// save the output to a file
mailcomposer.streamMessage();
mailcomposer.pipe(fs.createWriteStream("out.txt"));
If you do not want to use the streaming possibilities, you can compile the entire
message into a string in one go with buildMessage
.
mailcomposer.buildMessage(function(err, messageSource){
console.log(err || messageSource);
});
The function is actually just a wrapper around streamMessage
and emitted events.
Envelope can be generated with an getEnvelope()
which returns an object
that includes a from
address (string) and a list of to
addresses (array of
strings) suitable for forwarding to a SMTP server as MAIL FROM:
and RCPT TO:
.
console.log(mailcomposer.getEnvelope());
// {from:"sender@example.com", to:["receiver@example.com"]}
NB! both from
and to
properties might be missing from the envelope object
if corresponding addresses were not detected from the e-mail.
Tests are run with nodeunit
Run
npm test
MIT
FAQs
Compose E-Mail messages
The npm package mailcomposer receives a total of 45,210 weekly downloads. As such, mailcomposer popularity was classified as popular.
We found that mailcomposer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.