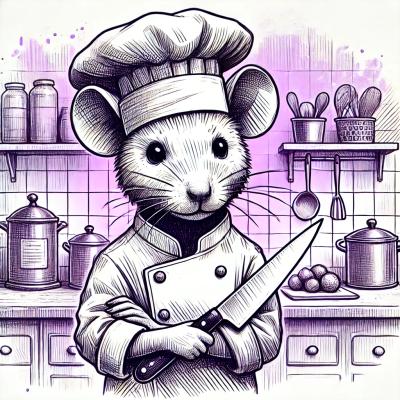
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The map-visit npm package is designed to map over the properties of an object and apply a function to each property's value. It is particularly useful for manipulating and transforming object properties in a flexible and customizable way.
Mapping over object properties
This feature allows you to iterate over each property of an object and apply a function to its value. In the provided code sample, the function converts each property value to uppercase and logs it along with its key.
const mapVisit = require('map-visit');
const obj = { a: 'foo', b: 'bar' };
mapVisit(obj, (value, key) => console.log(key, value.toUpperCase()));
Similar to map-visit, object-map allows mapping over an object's properties to transform their values. However, object-map returns a new object with the transformed properties, whereas map-visit applies a function for side effects without necessarily returning a result.
map-obj is another package that provides functionality to map keys and values of an object. Unlike map-visit, which is used for applying functions to object properties primarily for side effects, map-obj focuses on transforming the keys and values and returning a new object, which can be useful for more functional programming approaches.
Map
visit
over an array of objects.
Install with npm
$ npm i map-visit --save
var mapVisit = require('map-visit');
Let's say you want to add a set
method to your application that will:
data
objectdata
objectExample using extend
Here is one way to accomplish this using Lo-Dash's extend
:
var _ = require('lodash');
var obj = {
data: {},
set: function (key, value) {
if (Array.isArray(key)) {
_.extend.apply(_, [obj.data].concat(key));
} else if (typeof key === 'object') {
_.extend(obj.data, key);
} else {
obj.data[key] = value;
}
}
};
obj.set('a', 'a');
obj.set([{b: 'b'}, {c: 'c'}]);
obj.set({d: {e: 'f'}});
console.log(obj.data);
//=> {a: 'a', b: 'b', c: 'c', d: { e: 'f' }}
The above approach works fine for most use cases. But if you also want to emit an event each time a property is added to the data
object. A better approach would be to use visit
.
Example using visit
In this approach, when an array is passed to set
, mapVisit
calls set
on each object in the array. When an object is passed, visit
calls set
on each property in the object. As a result, the data
event will be emitted every time a property is added to data
.
var mapVisit = require('map-visit');
var visit = require('object-visit');
var obj = {
data: {},
set: function (key, value) {
if (Array.isArray(key)) {
mapVisit(obj, 'set', key);
} else if (typeof key === 'object') {
visit(obj, 'set', key);
} else {
// some event-emitter
console.log('emit', key, value);
obj.data[key] = value;
}
}
};
obj.set('a', 'a');
obj.set([{b: 'b'}, {c: 'c'}]);
obj.set({d: {e: 'f'}});
obj.set({g: 'h', i: 'j', k: 'l'});
console.log(obj.data);
//=> {a: 'a', b: 'b', c: 'c', d: { e: 'f' }, g: 'h', i: 'j', k: 'l'}
// events would look something like:
// emit a a
// emit b b
// emit c c
// emit d { e: 'f' }
// emit g h
// emit i j
// emit k l
Install dev dependencies:
$ npm i -d && npm test
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Jon Schlinkert
Copyright © 2015 Jon Schlinkert Released under the MIT license.
This file was generated by verb-cli on October 06, 2015.
FAQs
Map `visit` over an array of objects.
The npm package map-visit receives a total of 4,929,684 weekly downloads. As such, map-visit popularity was classified as popular.
We found that map-visit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.