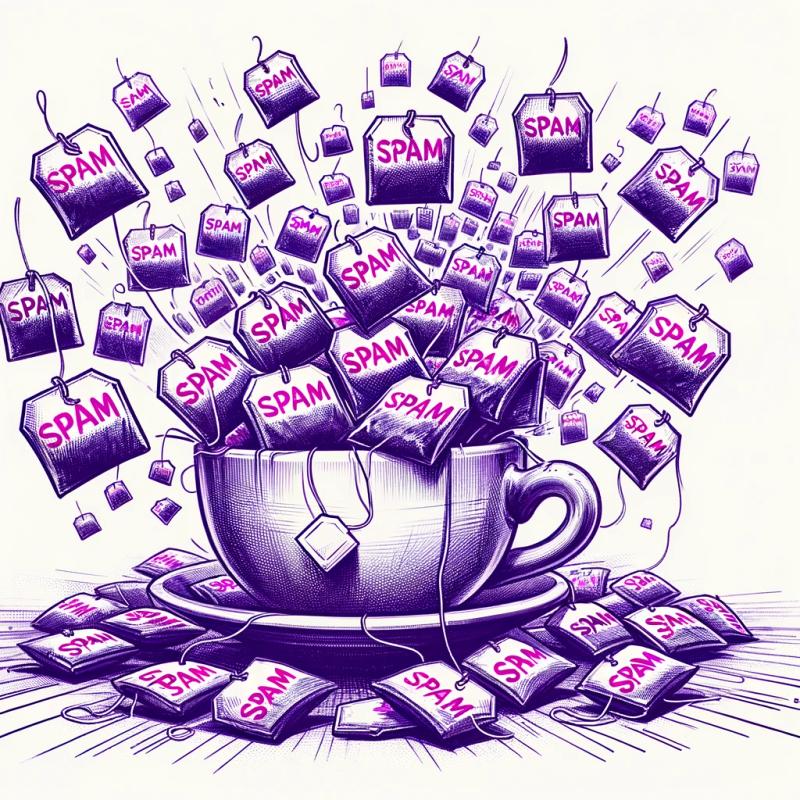
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
mersenne-twister
Advanced tools
Readme
Mersenne Twister pseudorandom number generator.
Origin source (generator interface was changed)
Algorithm - http://en.wikipedia.org/wiki/Mersenne_twister
$ npm install mersenne-twister
var MersenneTwister = require('mersenne-twister');
var generator = new MersenneTwister();
// Generates a random number on [0,1) real interval (same interval as Math.random)
generator.random();
// [0, 4294967295]
generator.random_int();
// [0,1]
generator.random_incl();
// (0,1)
generator.random_excl();
// [0,1) with 53-bit resolution
generator.random_long();
// [0, 2147483647]
generator.random_int31();
If you want to use a specific seed in order to get a repeatable random sequence, pass an integer into the constructor:
var generator = new MersenneTwister(123);
and that will always produce the same random sequence.
Also you can do it on existing generator instance:
generator.init_seed(123);
See source
FAQs
Mersenne twister pseudorandom number generator
The npm package mersenne-twister receives a total of 340,713 weekly downloads. As such, mersenne-twister popularity was classified as popular.
We found that mersenne-twister demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.