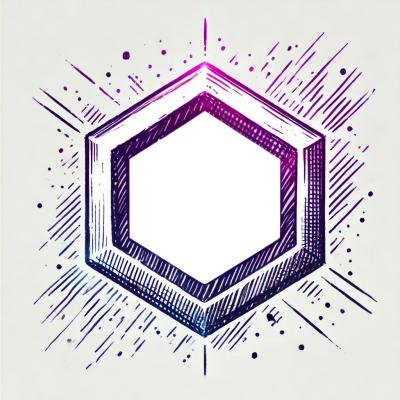
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
msc-collages
Advanced tools
People love collages. With collages we could combined several images together and make them more vivid and interesting. Developers could apply <msc-collages /> instead of annoying HTML code & CSS setting. All we need to do is just make a few setting and e
People love collages. With collages we could combined several images together and make them more vivid and interesting. Developers could apply <msc-collages /> instead of annoying HTML code & CSS setting. All we need to do is just make a few setting and everything will be all set.
<script
type="module"
src="https://your-domain/wc-msc-collages.js"
</script>
<msc-collages>
<script type="application/json">
{
"theme": 8, // 1 ~ 8
"object-fit": "cover", // cover || contain
"collages": [
{
"link": "?",
"src": "https://picsum.photos/300/300?grayscale&random=1",
"alt": "grayscale 1",
"target": "_blank"
},
{
"link": "?",
"src": "https://picsum.photos/300/300?grayscale&random=2",
"alt": "grayscale 2",
"target": "_blank"
},
{
"link": "?",
"src": "https://picsum.photos/300/300?grayscale&random=3",
"alt": "grayscale 3",
"target": "_blank"
},
{
"link": "?",
"src": "https://picsum.photos/300/300?grayscale&random=4",
"alt": "grayscale 4",
"target": "_blank"
}
]
}
</script>
</msc-collages>
Otherwise, developers could also choose remoteconfig
to fetch config for <msc-collages />.
<msc-collages
remoteconfig="https://your-domain/api-path"
...
></msc-collages>
<msc-collages /> could also use JavaScript to create DOM element. Here comes some examples.
<script type="module">
import { MscCollages } from 'https://your-domain/wc-msc-collages.js';
//use DOM api
const nodeA = document.createElement('msc-collages');
document.body.appendChild(nodeA);
nodeA.theme = 1;
nodeA.collages = [ {...} ];
// new instance with Class
const nodeB = new MscCollages();
document.body.appendChild(nodeB);
nodeB.theme = 2;
nodeB.collages = [ {...}, {...} ];
// new instance with Class & default config
const config = {
theme: 3,
collages: [
{...},
{...},
...
]
};
const nodeC = new MscCollages(config);
document.body.appendChild(nodeC);
</script>
<msc-collages /> uses CSS variables to hook uploader trigger theme & drop zone. That means developer could easy change it into the looks you like.
<style>
msc-collages {
--msc-collages-gap: 1px;
--msc-collages-overlay: #1d2228;
--msc-collages-border-radius: 8px;
}
</style>
<msc-collages /> supports some attributes to let it become more convenience & useful.
Set theme id for different usage. Developers could set 1
~ 8
theme. Default is "1
".
<msc-collages
theme="1"
...
></msc-collages>
Set image render property. This attribute only accept cover
or contain
. Default is "cover
".
<msc-collages
object-fit="cover"
...
></msc-collages>
Set collages data. This should be JSON string and each element needs contains "link
"、"src
"、"alt
"、"target
" for rendering. Max count is 4.
<msc-collages
collages='[{"link":"?","src":"https://picsum.photos/300/300?grayscale&random=1","alt":"grayscale 1","target":"_blank"}]'
...
></msc-collages>
Property Name | Type | Description |
---|---|---|
theme | String | Getter / Setter for theme id. |
object-fit | String | Getter / Setter for image render property. Only accept "cover " or "contain " |
collages | Object | Getter / Setter for collages data. |
Event Signature | Description |
---|---|
msc-collages-click | Fired when clicked. Developers could get original click event from event.detail.baseEvent to do preventDefault behavior. |
FAQs
People love collages. With collages we could combined several images together and make them more vivid and interesting. Developers could apply <msc-collages /> instead of annoying HTML code & CSS setting. All we need to do is just make a few setting and e
The npm package msc-collages receives a total of 0 weekly downloads. As such, msc-collages popularity was classified as not popular.
We found that msc-collages demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.