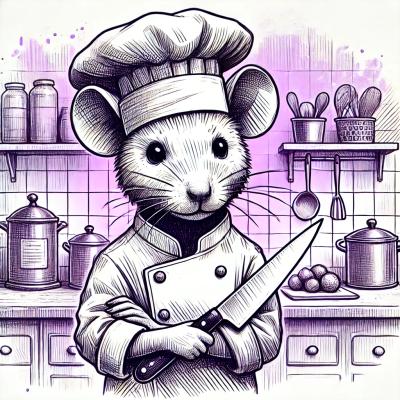
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
myinterview
Advanced tools
Build and integrate myInterview with ease via our node.js npm package and connect to the myInterview API.
The documentation for the myInterview API can be found here.
This project requires NodeJS (version 14 or later) and NPM. Node and NPM are really easy to install. To make sure you have them available on your machine, try running the following command.
$ npm -v && node -v
6.14.15
v14.18.1
npm i myinterview
:warning: Do not use this Node.js library in a front-end application. Doing so can expose your myInterview credentials to end-users as part of the bundled HTML/JavaScript sent to their browser.
const { MyInterviewApi } = require('myinterview');
MyInterviewApi.setConfig({
secret: 'yoursecret',
companyId: 'yourcompanyid',
accessKey: 'youraccesskey',
});
To use the SDK you have to set the configuration with the keys provided by myinterview prior use of the classes.
Field | Type | Description |
---|---|---|
statusCode | number | Status code of the response |
errorCode | number | Internal error code |
statusReason | string | Info message of the response |
callId | string | Internal call ID |
data | any | This field contain the data required based on the request |
time | Date / number | Timestamp |
This is the response object you will receive from the SDK the content of data will change based on your request.
Field | Type | Description |
---|---|---|
*errorMessage | string | Error message |
errorDetails | string | Error message details |
*statusCode | number | Status code of the response |
*errorCode | number | Internal error code |
*statusReason | string | Info message of the response |
*callId | string | Internal call ID |
*time | Date / number | Timestamp |
*required field |
const { Candidates } = require('myinterview');
const res = await Candidates.getCandidate('candidate_id');
const candidate = res.data;
const body = {
job_id: "job_id",
query: {
ids: ['candidate_id1', 'candidate_id2'],
email: 'email@email.com',
status: 'pending',
username: 'username',
skip: 0,
limit: 20,
}
};
const res = await Candidates.getJobCandidates(body);
const candidates = res.data;
Field | Type | Description |
---|---|---|
*job_id | string | ID of the job |
query.ids | string array | ids of candidates you need |
query.email | string | email of candidate you need |
query.status | string | status of the candidate enum (pending, clicked, completed, cancelled) |
query.username | string | username of candidate you need |
query.skip | number | Pagination |
query.limit | number | Pagination |
*required field |
const body = {
job_id: "job_id",
communication: true,
reminders: true,
deadlineDate: "2021-10-20T08:42:59.537+00:00",
timezoneForInvite: "Australia/Sydney",
candidates: [{
username: "username",
email: "email@email.com",
jobTitle: "Job title",
}]
};
await Candidates.createBulkCandidates(body);
Field | Type | Description |
---|---|---|
*job_id | string | ID of the job to connect the candidate |
communication | boolean | Flag to send mail to invited candidates (default = false) |
reminders | boolean | Flag to activate reminders mails |
deadlineDate | ISO Date string | Deadline date for the candidate/s |
timezoneForInvite | string | Timezone for the deadline |
*candidates | Array of candidate object | Array of candidate objects |
*required field |
This call will create one or more candidates and connect them to your Job.
const body = {
id: "candidate_id", // You have to provide the id of the candidate
username: "username",
email: "email@email.com",
jobTitle: "Job title",
};
await Candidates.updateCandidate(body);
Field | Type | Description |
---|---|---|
*id | string | ID of the candidate |
status | string | Status of the candidate |
username | string | Username of the candidate |
deadline | ISO Date string | Deadline date for the candidate/s |
timezoneForInvite | string | Timezone for the deadline |
*required field |
const { Companies } = require('myinterview')
const res = await Companies.getCompanyInfo();
const companyInfo = res.data;
const res = await Companies.getCompanyTemplates();
const templates = res.data;
const body = [{
id: "template_id",
name: "template_name",
questions: [{
numOfRetakes: 1,
partDuration: 30,
question: "How are you today?"
}],
introVideo: 'youtube.com/watch'
},
{
id: "template_id",
name: "template_name",
questions: [{
numOfRetakes: 1,
partDuration: 30,
question: "How are you today?"
}],
introVideo: 'youtube.com/watch'
}]
await Companies.createCompanyTemplateBulk(body);
Field | Type | Description |
---|---|---|
*id | string | ID of the company template |
hideQuestions | boolean | questions will not be displayed upfront |
*name | string | Name of the template |
introVideo | string | Url of the introduction video on the application page |
*questions | Array of job questions | Array of job questions objects |
*required field |
const body = {
id: "template_id",
name: "template_name",
questions: [{
numOfRetakes: 1,
partDuration: 30,
question: "How are you today?",
description: "some description"
}],
introVideo: 'youtube.com/watch'
};
await Companies.updateCompanyTemplate(body);
Field | Type | Description |
---|---|---|
*id | string | ID of the company template |
hideQuestions | boolean | questions will not be displayed upfront |
name | string | Name of the template |
introVideo | string | Url of the introduction video on the application page |
*questions | Array of job questions | Array of job questions objects |
*required field |
const { Jobs } = require('myinterview')
const res = await Jobs.getJobs();
const jobs = res.data;
const ids = ['id1', "id2"]
const res = await Jobs.getJobsWithIds(ids);
const jobs = res.data;
const id = "id"
const res = await Jobs.getJob(id);
const job = res.data;
const body = {
title: "job_title",
language: 'en',
overlay: '#000000',
config: {
hideQuestions: true,
practice: true
},
jobDescription: 'jobDescription',
logo: "logo.url.com",
questions: [{
numOfRetakes: 1,
partDuration: 30,
question: "How are you today?"
}],
}
await Jobs.createJob(body);
Field | Type | Description |
---|---|---|
*title | string | Title of the job |
language | string | Language of the job |
overlay | string | Overlay of the of the application page (Value in Color Hexa #000000) |
termsUrl | string | Url of the Terms of Service |
privacyUrl | string | Url of the Privacy |
backgroundImage | string | Background Image of the of the application page |
transcriptLanguage | string | Language of the transcript video |
*config | Config Object | Configuration object |
deadlineDate | ISO Date string | Deadline date for the job |
experience | string | experience of the job |
jobDescription | string | Job description |
logo | string | Logo |
*questions | Array of job questions | Array of job questions objects |
skills | Array of string | skills |
introVideo | string | introduction video for application page |
*required field |
const body = {
template_id: 'template_id', // If provided the questions will be taken from template
title: "job_title",
language: 'en',
overlay: '#000000',
config: {
practice: true
},
jobDescription: 'jobDescription',
logo: "logo.url.com",
questions: [{
numOfRetakes: 1,
partDuration: 30,
question: "How are you today?"
}],
candidates: {
communication: true,
reminders: true,
deadlineDate: "2021-10-20T08:42:59.537+00:00",
timezoneForInvite: "Australia/Sydney",
candidates: [{
username: "username",
email: "email@email.com",
}]
}
}
await Jobs.createJobWithCandidates(body);
Field | Type | Description |
---|---|---|
template_id | string | If the template ID is given you will create your job with the template questions |
*title | string | Title of the job |
questions | Array of job questions | Array of job questions objects is required if you don't provide template_id |
language | string | Language of the job |
overlay | string | Overlay of the of the application page (Value in Color Hexa #000000) |
termsUrl | string | Url of the Terms of Service |
privacyUrl | string | Url of the Privacy |
backgroundImage | string | Background Image of the of the application page |
transcriptLanguage | string | Language of the transcript video |
config | Config Object | Configuration object |
deadlineDate | ISO Date string | Deadline date for the job |
experience | string | experience of the job |
jobDescription | string | Job description |
logo | string | Logo |
skills | Array of string | skills |
introVideo | string | introduction video for application page |
*required field |
const body = {
job_id: 'job_id',
title: "job_title",
language: 'en',
overlay: '#000000',
config: {
hideQuestions: true,
practice: true
},
jobDescription: 'jobDescription',
logo: "logo.url.com",
questions: [{
numOfRetakes: 1,
partDuration: 30,
question: "How are you today?"
}],
}
await Jobs.updateJob(body);
Field | Type | Description |
---|---|---|
*job_id | string | ID of the job |
title | string | Title of the job |
language | string | Language of the job |
overlay | string | Overlay of the of the application page (Value in Color Hexa #000000) |
termsUrl | string | Url of the Terms of Service |
privacyUrl | string | Url of the Privacy |
backgroundImage | string | Background Image of the of the application page |
transcriptLanguage | string | Language of the transcript video |
config | Config Object | Configuration object |
deadlineDate | ISO Date string | Deadline date for the job |
experience | string | experience of the job |
jobDescription | string | Job description |
logo | string | Logo |
questions | Array of job questions | Array of job questions objects |
skills | Array of string | skills |
introVideo | string | introduction video for application page |
*required field |
const { Videos } = require('myinterview')
const body = {
video_id: 'video_id',
ai: true,
transcript: true
}
const res = await Videos.getVideo(body);
const video = res.data;
Field | Type | Description |
---|---|---|
*video_id | string | ID of the Video |
ai | boolean | add ai object to the video object |
transcript | boolean | add transcript object to the video object |
*required field |
const body = {
ai: true,
transcript: true,
username: 'fullname',
email: "email@example.com",
skip: 0,
limit: 20
}
const res = await Videos.getVideos(body);
const videos = res.data;
Field | Type | Description |
---|---|---|
ai | boolean | add ai object to the video object |
transcript | boolean | add transcript object to the video object |
username | string | get with specific username |
string | get with specific email | |
skip | number | for pagination |
limit | number | for pagination |
*required field |
const body = {
job_id: 'job_id',
query: { // Optional
email: "email@example.com",
username: "fullname",
candidate_id: "candidate_id",
skip: 20,
limit: 20
}
}
const res = await Videos.getJobVideos(body);
const videos = res.data;
Field | Type | Description |
---|---|---|
*job_id | string | ID of the job |
query | Query Object | Query object |
*required field |
const id = "video_id"
const res = await Videos.getShareLink(id);
const shareLink = res.data.shareLink;
const { Shares } = require('myinterview');
const body = {
candidate_videos: [
{
id: 'video_id',
},
],
job_id: 'job_id',
anonymised: true,
share_personality: true,
recipients: [
{
recipient_email: 'mail@myinterview.com',
recipient_name: 'Harry Potter',
},
],
};
const res = await Shares.getShortlistUrl(body);
const shortlistUrl = res.data.shortlistUrl;
Field | Type | Description |
---|---|---|
*candidate_videos | Array of Object | Ids of the videos you want to share |
*job_id | string | ID of the job |
anonymised | boolean | Flag to anonymised the candidates name |
share_personality | boolean | Flag to add AI Insights |
recipients | Array of Object | Array of recipients (recruiters) if you add some they will receive a mail with the link to comment the videos |
*required field |
const { Intelligence } = require('myinterview');
const body = {
job_id: 'job_id',
intelligence: [
{
name: 'Creative',
order: 1,
},
{
name: 'Persuasive',
order: 2,
},
{
name: 'Assertive',
order: 3,
},
{
name: 'Optimism',
order: 4,
},
{
name: 'Outgoing',
order: 5,
},
],
};
const res = await Intelligence.updateJobIntelligence(body);
Field | Type | Description |
---|---|---|
*job_id | string | job_id |
*intelligence.order | number | order from 1 to 5 |
*intelligence.name | string | trait name need to be one of these values: Creative,Strategic,Disciplined,Driven,Friendly,Outgoing,Assertive,Persuasive,Stress Tolerant,Optimism |
*required field |
const { Webhooks } = require('myinterview');
const body = {
url: 'https://webhook.test',
}
const res = await Webhooks.testWebhook(body);
Field | Type | Description |
---|---|---|
*url | string | webhook url |
*required field |
const body = {
url: 'https://webhook.io',
}
const res = await Webhooks.createWebhook(body);
Field | Type | Description |
---|---|---|
*url | string | webhook url |
*required field |
You can catch the error the same way you catch error normally.
try {
const res = await Videos.getVideoPersonality("");
} catch (error) {
// The error will be of type IApiErrorObj the definition is above.
console.log(error)
}
Videos.getVideoPersonality("").then((res) => {
// Type IApiResponseObj
}).catch((error) => {
// The error will be of type IApiErrorObj the definition is above.
console.log(error)
})
This call will populate the headers you need to connect to the global API service it will return an object that you can use in you request. In order to authenticate to the API you need to populate the headers (x-myinterview-timestamp, x-myinterview-key, x-myinterview-signed) the populateHeaders method will return an object that you need to include in the headers config.
import { MyInterviewApi } from 'myinterview';
MyInterviewApi.populateHeaders({
secret: 'yoursecret',
companyId: 'yourcompanyid',
accessKey: 'youraccesskey',
});
It will return:
{
'x-myinterview-timestamp': 'headTimestamp',
'x-myinterview-key': 'headKey',
'x-myinterview-signed': 'headSigned'
}
FAQs
Build and integrate myInterview with ease via our node.js npm package and connect to the myInterview API.
The npm package myinterview receives a total of 92 weekly downloads. As such, myinterview popularity was classified as not popular.
We found that myinterview demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.