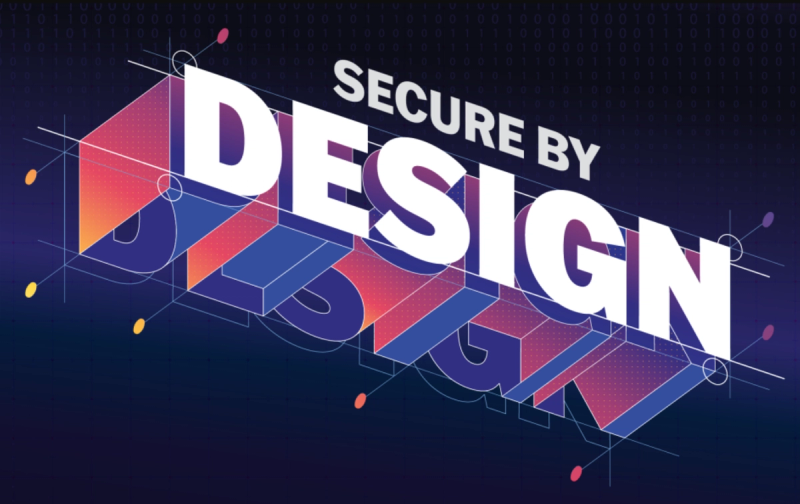
Security News
Socket Partners with CISA to Champion 'Secure by Design' Standards
Socket is joining forces with CISA and other industry leaders at the RSA Conference to sign the Secure by Design pledge, committing to uphold the highest security standards in our products.