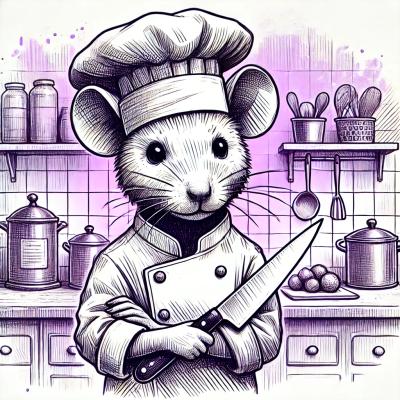
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
nanocomponent
Advanced tools
1kb
library to wrap native DOM libraries to work with DOM diffing algorithms.
// Implementer API
var Nanocomponent = require('nanocomponent')
var html = require('bel')
function MyButton () {
if (!(this instanceof MyButton)) return new MyButton()
this._color = null
Nanocomponent.call(this)
}
MyButton.prototype = Object.create(Nanocomponent.prototype)
MyButton.prototype._render = function () {
if (!this._element) {
// initial render
return html`
<button style="background-color: ${this.props.color}">
Click Me
</button>
`
} else {
// mutate this._element
this._element.style.backgroundColor = this.props.color
}
}
MyButton.prototype._update = function (props) {
return props.color !== this.props.color
}
// Consumer API
var MyButton = require('./my-button.js')
var myButton = MyButton()
document.body.appendChild(myButton.render())
No matter the language, inheritance is tricky. Each layer adds more abstractions and can make it hard to understand what's going on. That's why we don't recommend doing more than one level of inheritance. However, this means that any API built on top of Nanocomponent directly shouldn't also expose a prototypical API.
Instead we recommend people use an interface that somewhat resembles Node's
require('events').EventEmitter
API.
var MyComponent = require('./my-component')
var myComponent = MyComponent()
myComponent.on('render', function () {
console.log('rendered')
})
myComponent.on('load', function () {
console.log('loaded on DOM')
})
myComponent.on('unload', function () {
console.log('removed from DOM')
})
document.body.appendChild(myComponent.render())
This API allows consumers of the MyComponent
to hook into the event system
without needing to inherit. It also allows MyComponent
to expose more hooks
with little cost. See
yoshuawuyts/microcomponent for
an example of how to create a higher level interface.
Nanocomponent.prototype()
Inheritable Nanocomponent prototype. Should be inherited from using
Nanococomponent.call(this)
and prototype = Object.create(Nanocomponent.prototype)
.
Internal properties are:
this._placeholder
: placeholder element that's returned on subsequent
render()
calls that don't pass the ._update()
check.this._element
: rendered element that should be returned from the first
._render()
call. Used to apply ._load()
and ._unload()
listeners on.this._hasWindow
: boolean if window
exists. Can be used to create
elements that render both in the browser and in Node.this._loaded
: boolean if the element is currently loaded on the DOM.this._onload
: reference to the on-load library.this.props
: the current props
passed to render()
this.oldProps
: the previous props
this.state
: any out of band state to be storedDOMNode|placeholder = Nanocomponent.prototype.render(props)
Create an instance of the component. Calls prototype._render()
if
prototype._update()
returns true
. As long as the element is mounted on the
DOM, subsequent calls to .render()
will return a placeholder element with a
.isSameNode()
method that compares arguments with the previously rendered
node. This is useful for diffing algorithms like
nanomorph which use this method to
determine if a portion of the tree should be walked.
Nanocomponent.prototype._render(props)
Must be implemented. Render an HTML node with arguments. For
prototype._load()
and prototype._unload()
to work, make sure you return the
same node on subsequent renders. The Node that's initially returned is saved as
this._element
.
Nanocomponent.prototype._update(props)
Must be implemented. Return a boolean to determine if prototype._render()
should be called. Not called on the first render.
Nanocomponent.prototype._load()
Called when the component is mounted on the DOM.
Nanocomponent.prototype._unload()
Called when the component is removed from the DOM.
$ npm install nanocomponent
FAQs
Native DOM components that pair nicely with DOM diffing algorithms
The npm package nanocomponent receives a total of 417 weekly downloads. As such, nanocomponent popularity was classified as not popular.
We found that nanocomponent demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 30 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.