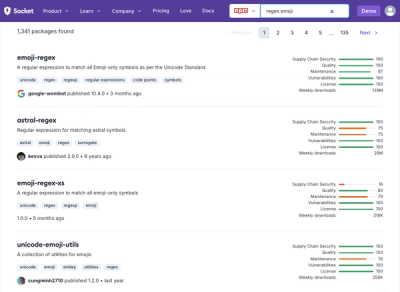
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
nestjs-pino-stackdriver
Advanced tools
Pino logger for Nestjs adapted to be used with Stackdriver
Nestjs Pino Stackdriver exports a LoggerService that includes a logger context, as the default logger implementation in Nest. It can be configured to log parts from the Request and to log parts of the default context-storage of the application. You can also set custom (static) labels.
Futhermore:
You can continue using your LoggerModule as you were using it before 1.x:
import { Module } from '@nestjs/common';
import { CqrsModule } from '@nestjs/cqrs';
import {
LoggerModule,
} from 'nestjs-pino-stackdriver';
import { ExampleController } from './example.controller';
import { ExampleHandler } from './command/handler/example.handler';
@Module({
imports: [
LoggerModule, // or LoggerModule.forRoot() or LoggerModule.forRoot({})
CqrsModule,
],
controllers: [ExampleController],
providers: [ExampleHandler],
})
export class ExampleModule {}
You just need to stop using CorrelationTracerMiddleware, just call GcloudTraceService.start() instead:
// 0.x
import { NestFactory } from '@nestjs/core';
import { MyModule } from './my/my.module';
import { CorrelationTracerMiddleware } from 'nestjs-pino-stackdriver';
async function bootstrap() {
const app = await NestFactory.create(MyModule);
app.use(CorrelationTracerMiddleware({ app }));
await app.init();
}
bootstrap();
// 1.x
import { NestFactory } from '@nestjs/core';
import { MyModule } from './my/my.module';
import { GcloudTraceService } from 'nestjs-pino-stackdriver';
async function bootstrap() {
const app = await NestFactory.create(MyModule);
await app.init();
}
GcloudTraceService.start();
bootstrap();
Now you can inject the logger in your providers or controllers and use it:
import { Controller, Post, Body } from '@nestjs/common';
import { CommandBus } from '@nestjs/cqrs';
import { Logger } from 'nestjs-pino-stackdriver';
import { ExampleCommand } from './command/impl/example.command';
@Controller()
export class ExampleController {
constructor(
private readonly commandBus: CommandBus,
private readonly logger: Logger,
) {
// if you do not call setContext, "ExampleController" will be used as context
// logger.setContext('my custom context');
}
@Post('/example')
async example(
@Body()
command: ExampleCommand,
) {
this.logger.verbose('Simple verbose message');
this.logger.debug({
msg: 'Object-like debug message',
sample: 'another field',
});
this.logger.warn('Warning passing custom context', 'custom-context');
this.logger.error(
'Error',
`An error trace`,
);
this.logger.log(
'An interpolation message: %o correlation-id %s',
undefined,
{ try: 1 },
'xxx',
);
return this.commandBus.execute(command);
}
}
You can use the logger to log your application logs
import { NestFactory } from '@nestjs/core';
import { createStackdriverLoggerTool, GcloudTraceService } from 'nestjs-pino-stackdriver';
import { MyModule } from './my.module';
async function bootstrap() {
const app = await NestFactory.create(MyModule);
// Pass the app to createStackdriverLoggerTool
// and it will instantiate your logger from the DI
app.useLogger(createStackdriverLoggerTool(app));
return await app.listen(3000);
}
GcloudTraceService.start();
bootstrap();
You can use also use the logger to log your application + initialization logs
import { NestFactory } from '@nestjs/core';
import { createStackdriverLoggerTool, GcloudTraceService } from 'nestjs-pino-stackdriver';
import { MyModule } from './my.module';
import { myLoggerConfig } from './my-logger.config';
async function bootstrap() {
// pass your logger config to createStackdriverLoggerTool
// and it will create a logger with your config
const app = await NestFactory.create(MyModule, {logger: createStackdriverLoggerTool(myLoggerConfig)});
return await app.listen(3000);
}
GcloudTraceService.start();
bootstrap();
You can configure your logger to include environment, request and context labels on each log:
import { Module } from '@nestjs/common';
import { CqrsModule } from '@nestjs/cqrs';
import {
LoggerModule,
} from 'nestjs-pino-stackdriver';
import { ExampleController } from './example.controller';
import { ExampleHandler } from './command/handler/example.handler';
@Module({
imports: [
// ...or LoggerModule.forRoot
LoggerModule.register({
labels: {
env: [
{ label: 'project', value: 'example' },
{ label: 'node-env', path: 'NODE_ENV' },
],
request: [
{
label: 'content-type',
pick: { headers: ['content-type'] },
},
{
label: 'entity-id',
pick: [
{ query: ['fallback-id'], body: ['entity-id', 'id'] },
{ query: ['override-id'] },
],
},
{
label: 'deep-in-body',
pick: [{ body: ['deep.id'] }],
path: 'deep-in-body.id',
},
],
},
}),
CqrsModule,
],
controllers: [ExampleController],
providers: [ExampleHandler],
})
export class ExampleModule {}
Please refer to nestjs-pino-stackdriver for further information about the configuration of the logger.
If you want to use the logger without using the CorrelationIdModule or the GcloudTraceModule, use the PinoContextModule instead:
// In your module:
import { Module } from '@nestjs/common';
import {
PinoContextModule,
PredefinedConfig,
CorrelationIdModule,
GcloudTraceModule,
} from 'nestjs-pino-stackdriver';
@Module({
imports: [
CorrelationIdModule.register(), // do not include this if you do not want to include Correlation Id module
GcloudTraceModule, // do not include this if you do not want to use Gcloud Trace
PinoContextModule.register({
base: PredefinedConfig.STACKDRIVER,
labels: {
env: [
{ label: 'project', value: 'example' },
],
request: [
{
label: 'content-type',
pick: { headers: ['content-type'] },
},
],
},
}),
]
(...)
// In your application:
import { NestFactory } from '@nestjs/core';
import { GcloudTraceService, PinoContextLogger } from 'nestjs-pino-stackdriver';
import { OnboardingModule } from './onboarding/my.module';
async function bootstrap() {
const app = await NestFactory.create(MyModule);
await app.listen(3000);
}
// Do not include this if you do not want to use GcloudTrace
GcloudTraceService.start();
bootstrap();
You need to inject the PinoContextLogger instead of the default Logger:
import { Controller, Post, Body } from '@nestjs/common';
import { CommandBus } from '@nestjs/cqrs';
import { PinoContextLogger } from 'nestjs-pino-stackdriver';
import { ExampleCommand } from './command/impl/example.command';
@Controller()
export class ExampleController {
constructor(
private readonly commandBus: CommandBus,
private readonly logger: PinoContextLogger,
) {
// if you do not call setContext, "ExampleController" will be used as context
// logger.setContext('my custom context');
}
@Post('/example')
async example(
@Body()
command: ExampleCommand,
) {
this.logger.log('Simple message');
return this.commandBus.execute(command);
}
}
This project include several decorators to get the correlation-id into your providers and controllers:
import { CorrelationIdMetadata } from 'nestjs-correlation-id';
@CorrelationIdMetadata()
export class ExampleEvent {
constructor(public readonly data: any, public readonly metadata: any) {}
}
import { CorrelationId } from 'nestjs-pino-stackdriver';
export class InternalServerErrorException {
@CorrelationId()
private readonly correlationId;
}
import { AddCorrelationId } from 'nestjs-pino-stackdriver';
@AddCorrelationId('property.correlation_id')
export class ExampleClass {
private readonly property = {}; // property.correlation_id will be added / overwritten
}
Use the BuildDto decorator to facilitate the creation of your CQRS commands or your DTOs:
import { Controller, Post } from '@nestjs/common';
import { CommandBus } from '@nestjs/cqrs';
import { BuildDto } from 'nestjs-pino-stackdriver';
import { ExampleCommand } from './example/src/command/impl/example.command';
@Controller()
export class ExampleController {
constructor(
private readonly commandBus: CommandBus,
) {}
@Post('/example')
async example(
@BuildDto({
query: false,
body: Object.getOwnPropertyNames(new ExampleCommand()),
headers: ['x-correlation-id'],
})
command: ExampleCommand,
) {
return this.commandBus.execute(command);
}
Create an issue.
There is a full working example in the directory "example" of each project inside this project:
FAQs
NestJS Pino logger with Stackdriver support
The npm package nestjs-pino-stackdriver receives a total of 309 weekly downloads. As such, nestjs-pino-stackdriver popularity was classified as not popular.
We found that nestjs-pino-stackdriver demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.