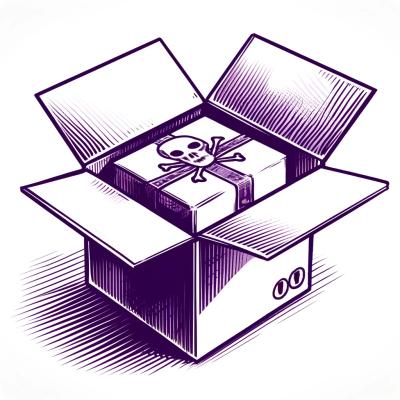
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
next-transpile-modules
Advanced tools
The next-transpile-modules package is a Next.js plugin that allows you to transpile code from node_modules. This is particularly useful when you are using third-party packages that are not pre-transpiled to ES5/ES6, or when you want to customize the build process for specific modules.
Transpile specific modules
This feature allows you to specify which modules from node_modules should be transpiled. This is useful for ensuring compatibility with older browsers or customizing the build process for specific modules.
const withTM = require('next-transpile-modules')(['some-module', 'and-another']);
module.exports = withTM({
// Your Next.js config
});
Custom Babel configuration
You can customize the Babel configuration for the transpiled modules. This allows you to add specific Babel plugins or presets that are required for your project.
const withTM = require('next-transpile-modules')(['some-module']);
module.exports = withTM({
babel: {
presets: ['next/babel'],
plugins: ['styled-components']
}
});
Support for monorepos
The package supports monorepos by allowing you to transpile packages from a monorepo setup. This is useful for large projects that are split into multiple packages.
const withTM = require('next-transpile-modules')(['@my-scope/my-package']);
module.exports = withTM({
// Your Next.js config
});
babel-plugin-module-resolver is a Babel plugin that allows you to customize the resolution of modules. It can be used to alias module paths, making it easier to manage imports in large projects. Unlike next-transpile-modules, it does not specifically target Next.js or handle transpilation of node_modules.
next-compose-plugins is a utility for composing multiple Next.js plugins into a single configuration. While it does not specifically handle transpilation of node_modules, it can be used in conjunction with next-transpile-modules to manage complex Next.js configurations.
babel-plugin-transform-require-ignore is a Babel plugin that allows you to ignore specific modules during the build process. This can be useful for excluding non-JavaScript files or modules that should not be transpiled. It is more limited in scope compared to next-transpile-modules, which focuses on transpiling specific modules.
Note All
next-transpile-modules
features have natively landed to Next.js 13.1, you can check the migration guide here.This package is now officially deprecated and on life-support.
PRs with fixes are welcome and I will help review them, but that's it. I highly recommend contributing to Next.js in case your setup is not working there.
node_modules
Transpile modules from node_modules
using the Next.js Babel configuration.
Makes it easy to have local libraries and keep a slick, manageable dev experience.
.js
, .jsx
, .ts
, .tsx
, .mjs
, .css
, .scss
and .sass
This plugin aims to solve the following challenges:
styleguide
package)lodash-es
)What this plugin does not aim to solve:
Next.js version | Plugin version |
---|---|
Next.js 13 | 10.x |
Next.js 12 | 9.x |
Next.js 11 | 8.x |
Next.js 9.5+ / 10 | 4.x, 5.x, 6.x, 7.x |
Next.js 9.2 | 3.x |
Next.js 8 / 9 | 2.x |
Next.js 6 / 7 | 1.x |
Latest Next.js version tested: 13.4.12.
npm install --save next-transpile-modules
or
yarn add next-transpile-modules
transpileModules
String[]: modules to be transpiledoptions
Object (optional)
resolveSymlinks
Boolean: Enable symlinks resolution to their real path by Webpack (default to true
)debug
Boolean: Display some informative logs in the console (can get noisy!) (default to false
)__unstable_matcher
(path) => boolean: Custom matcher that will override the default one. Don't use it.resolveSymlinks
Node.js resolution is based on the fact that symlinks are resolved. Not resolving them will alter the behavior, but there are some cases where the alternative behavior makes things a lot easier.
If:
npm/yarn link
to link packages into node_modules.npm
with file:
dependencies that live outside of your project directory
npm
will create symlinks in this case. Yarn will copy instead.you should set resolveSymlinks: false
, which results which will make things work as expected.
For other scenarios like:
pnpm
yarn/npm
workspacesyarn 2
portalsyou should keep resolveSymlinks: true
(default).
// next.config.js
const withTM = require('next-transpile-modules')(['somemodule', 'and-another']); // pass the modules you would like to see transpiled
module.exports = withTM({});
Notes:
withTM
as your last plugin (the outermost one).main
field.7.1.0
)You can include scoped packages or nested ones:
const withTM = require('next-transpile-modules')(['@shared/ui', '@shared/utils']);
// ...
const withTM = require('next-transpile-modules')(['styleguide/node_modules/lodash-es']);
// ...
If you need to install all packages of a certain scope (it is highly discouraged), you can do the following:
const packageJSON = require('./package.json');
const transpiledPackages = Object.keys(packageJSON.dependencies).filter((it) => it.includes('@shared/'));
const withTM = require('next-transpile-modules')(transpiledPackages);
next-compose-plugins
:const withPlugins = require('next-compose-plugins');
const withTM = require('next-transpile-modules')(['some-module', 'and-another']);
module.exports = withPlugins([withTM], {
// ...
});
Since next-transpile-modules@3
and next@>9.2
, this plugin can also transpile CSS included in your transpiled packages. SCSS/SASS is also supported since next-transpile-modules@3.1.0
.
In your transpiled package:
// shared-ui/components/Button.js
import styles from './Button.module.css';
function Button(props) {
return (
<button type='button' className={styles.error}>
{props.children}
</button>
);
}
export default Button;
/* shared-ui/components/Button.module.css */
.error {
color: white;
background-color: red;
}
In your app:
// next.config.js
const withTM = require('next-transpile-modules')(['shared-ui']);
// ...
// pages/home.jsx
import React from 'react';
import Button from 'shared-ui/components/Button';
const HomePage = () => {
return (
<main>
{/* will output <button class="Button_error__xxxxx"> */}
<Button>Styled button</Button>
</main>
);
};
export default HomePage;
It also supports global CSS import packages located in node_modules
:
// pages/_app.js
import 'shared-ui/styles/global.css'; // will be imported globally
export default function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
cd next-transpile-modules
yarn
If you want to update the Next.js tested version:
scripts/next-update.sh
with the latest Next.js versionnpm
, yarn
and pnpm
are installedyarn setup
bash scripts/next-update.sh
Push your changes and Github Actions will test everything as it should be.
@weco/next-plugin-transpile-modules
?@weco
's seems dead.mjs
It is important to understand that this plugin is a big hack of the Next.js Webpack configuration. When the Next.js team pushes an update to their build configuration, the changes next-transpile-modules
bring may be outdated, and the plugin needs to be updated (which is a breaking change for this plugin, as the updated plugin is usually not retro-compatible with the previous versions of Next.js).
Now, this build problem can happen when you install your dependencies with npm install
/yarn install
(in your CI pipeline for example). Those commands may re-resolve your next
dependency of your package.json
to a newer one, and this newer one may have critical Webpack changes, hence breaking your build.
The way to fix it is easy, and it is what you should always do: install your dependencies with npm ci
("clean install") or yarn --frozen-lockfile
. This will force npm
or yarn
to use the version of Next.js declared in your lock file, instead of downloading the latest one compatible with the version accepted by your package.json
.
So basically: use your lock files right, and understand what problems they are solving ;)
more:
Please make sure to read the changelog.
.babelrc
If you get a transpilation error when using a custom Babel configuration, make sure you are using a babel.config.js
and not a .babelrc
.
The former is a project-wide Babel configuration, when the latter works for relative paths only (and may not work for Yarn for example, as it installs dependencies in a parent directory).
If you add a local library (let's say with yarn add ../some-shared-module
), Yarn will copy those files by default, instead of symlinking them. So your changes to the initial folder won't be copied to your Next.js node_modules
directory.
You can go back to npm
, or use Yarn workspaces. See an example in the official Next.js repo.
config.optimization.minimize = false;
to you next.config.js
's Webpack configurationLerna's purpose is to publish different packages from a monorepo, it does not help for and does not intend to help local development with local modules (<- this, IN CAPS).
This is not coming from me, but from Lerna's maintainer.
So you are probably using it wrong, and I advice you to use npm
or Yarn workspaces instead.
Again, most probably a bad idea. You may need to tell your Webpack configuration how to properly resolve your scoped packages, as they won't be installed in your Next.js directory, but the root of your Lerna setup.
const withTM = require('next-transpile-modules')(['@your-project/shared', '@your-project/styleguide']);
module.exports = withTM({
webpack: (config, options) => {
config.resolve.alias = {
...config.resolve.alias,
// Will make webpack look for these modules in parent directories
'@your-project/shared': require.resolve('@your-project/shared'),
'@your-project/styleguide': require.resolve('@your-project/styleguide'),
// ...
};
return config;
},
});
Invalid hook call
error in react
It can happen that when using next-transpile-modules
with a local package and npm
, you end up with duplicated dependencies in your final Next.js build. It is important to understand why it happens.
Let's take the following setup: one Next.js app ("Consumer"), and one Styleguide library.
You will probably have react
as a peerDependencies
and as a devDependecy
of the Styleguide. If you use npm i
, it will create a symlink to your Styleguide package in your "Consumer" node_modules
.
The thing is in this shared package, you also have a node_modules
. So when your shared modules requires, let's say react
, Webpack will resolve it to the version in your Styleguide's node_modules
, and not your Consumer's node_modules
. Hence the duplicated react
in your final bundles.
You can tell Webpack how to resolve the react
of your Styleguide to use the version in your Next.js app like that:
const withTM = require('next-transpile-modules')(['styleguide']);
module.exports = withTM({
webpack: (config, options) => {
+ if (options.isServer) {
+ config.externals = ['react', ...config.externals];
+ }
+
+ config.resolve.alias['react'] = path.resolve(__dirname, '.', 'node_modules', 'react');
return config
},
});
Please note, the above will only work if react
is properly declared as peerDependencies
or devDependencies
in your referenced package.
It is not a great solution, but it works. Any help to find a more future-proof solution is welcome.
All the honor goes to James Gorrie who created the first version of this plugin.
FAQs
Next.js plugin to transpile code from node_modules
The npm package next-transpile-modules receives a total of 251,472 weekly downloads. As such, next-transpile-modules popularity was classified as popular.
We found that next-transpile-modules demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.