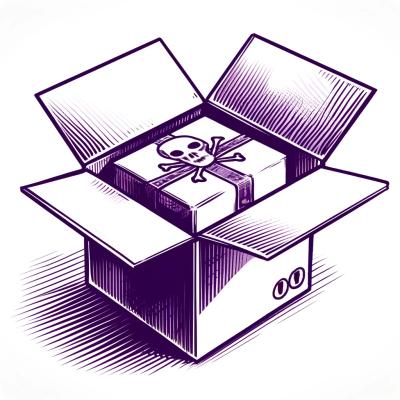
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
ngx-device-detector
Advanced tools
The ngx-device-detector package is an Angular library that provides tools to detect the device, OS, and browser information of the client. It helps in customizing the user experience based on the device type, operating system, and browser being used.
Detect Device Type
This feature allows you to detect the type of device (mobile, tablet, or desktop) the user is using. The code sample demonstrates how to use the DeviceDetectorService to determine the device type and display it in a component.
import { Component, OnInit } from '@angular/core';
import { DeviceDetectorService } from 'ngx-device-detector';
@Component({
selector: 'app-device-info',
template: '<p>Device Type: {{ deviceType }}</p>'
})
export class DeviceInfoComponent implements OnInit {
deviceType: string;
constructor(private deviceService: DeviceDetectorService) {}
ngOnInit() {
this.deviceType = this.deviceService.isMobile() ? 'Mobile' : this.deviceService.isTablet() ? 'Tablet' : 'Desktop';
}
}
Detect Operating System
This feature allows you to detect the operating system of the user's device. The code sample shows how to use the DeviceDetectorService to get the OS information and display it in a component.
import { Component, OnInit } from '@angular/core';
import { DeviceDetectorService } from 'ngx-device-detector';
@Component({
selector: 'app-os-info',
template: '<p>Operating System: {{ os }}</p>'
})
export class OsInfoComponent implements OnInit {
os: string;
constructor(private deviceService: DeviceDetectorService) {}
ngOnInit() {
this.os = this.deviceService.os;
}
}
Detect Browser
This feature allows you to detect the browser being used by the client. The code sample demonstrates how to use the DeviceDetectorService to get the browser information and display it in a component.
import { Component, OnInit } from '@angular/core';
import { DeviceDetectorService } from 'ngx-device-detector';
@Component({
selector: 'app-browser-info',
template: '<p>Browser: {{ browser }}</p>'
})
export class BrowserInfoComponent implements OnInit {
browser: string;
constructor(private deviceService: DeviceDetectorService) {}
ngOnInit() {
this.browser = this.deviceService.browser;
}
}
The device-detector-js package is a JavaScript library that provides similar functionalities to ngx-device-detector. It can detect the device type, operating system, and browser information. Unlike ngx-device-detector, which is specifically designed for Angular, device-detector-js can be used in any JavaScript environment.
The mobile-detect package is a lightweight JavaScript library that focuses on detecting mobile devices. It provides information about the device type, operating system, and user agent. While it offers similar functionalities to ngx-device-detector, it is more specialized for mobile detection and can be used in various JavaScript environments.
The ua-parser-js package is a JavaScript library that parses user-agent strings to detect browser, engine, OS, CPU, and device type/model. It offers a broader range of detection capabilities compared to ngx-device-detector and can be used in any JavaScript environment.
An Angular 6+ powered AOT compatible device detector that helps to identify browser, os and other useful information regarding the device using the app. The processing is based on user-agent. This library was built at KoderLabs, which is one of the best places I've worked at ❤️
New package :
If you use Angular 5, you must use v1.5.2 or earlier
Latest version available for each version of Angular
ngx-device-detector | Angular |
---|---|
1.3.3 | 7.x |
1.3.5 | 8.x |
1.4.1 | 9.x |
1.4.5 | 10.x |
2.0.5 | 11.x |
2.1.0 | 12.x |
3.x.x | 13.x |
4.x.x | 14.x |
5.x.x | 15.x |
6.x.x | 16.x |
7.x.x | 17.x |
8.x.x | 18.x |
9.x.x | 19.x |
To install this library, run:
$ npm install ngx-device-detector --save
In your component where you want to use the Device Service
import { Component } from '@angular/core';
...
import { DeviceDetectorService } from 'ngx-device-detector';
...
@Component({
selector: 'home', // <home></home>
styleUrls: [ './home.component.scss' ],
templateUrl: './home.component.html',
...
})
export class HomeComponent {
deviceInfo = null;
...
constructor(..., private http: Http, private deviceService: DeviceDetectorService) {
this.epicFunction();
}
...
epicFunction() {
console.log('hello `Home` component');
this.deviceInfo = this.deviceService.getDeviceInfo();
const isMobile = this.deviceService.isMobile();
const isTablet = this.deviceService.isTablet();
const isDesktopDevice = this.deviceService.isDesktop();
console.log(this.deviceInfo);
console.log(isMobile); // returns if the device is a mobile device (android / iPhone / windows-phone etc)
console.log(isTablet); // returns if the device us a tablet (iPad etc)
console.log(isDesktopDevice); // returns if the app is running on a Desktop browser.
}
...
}
For SSR, you have to make sure that the User Agent is available for device detection. I.e. you'll need to provide it manually. If using ExpressJS for example:
express.tokens.ts
import { InjectionToken } from '@angular/core';
import { Request, Response } from 'express';
export const REQUEST = new InjectionToken<Request>('REQUEST');
export const RESPONSE = new InjectionToken<Response>('RESPONSE');
universal-device-detector.service.ts:
import { Inject, Injectable, Optional, PLATFORM_ID } from '@angular/core';
import { REQUEST } from 'path/to/express.tokens';
import { Request } from 'express';
import { DeviceDetectorService } from 'ngx-device-detector';
import { isPlatformServer } from '@angular/common';
@Injectable()
export class UniversalDeviceDetectorService extends DeviceDetectorService {
constructor(@Inject(PLATFORM_ID) platformId: any, @Optional() @Inject(REQUEST) request: Request) {
super(platformId);
if (isPlatformServer(platformId)) {
super.setDeviceInfo((request.headers['user-agent'] as string) || '');
}
}
}
app.server.module.ts:
{
provide: DeviceDetectorService,
useClass: UniversalDeviceDetectorService
},
Holds the following properties
To generate all *.js
, *.js.map
and *.d.ts
files:
$ npm run tsc
To lint all *.ts
files:
$ npm run lint
To run unit tests
$ npm run test
To build the library
$ npm run build
Make sure you have @angular/cli installed
$ npm install -g @angular/cli
$ cd demo
$ npm install
$ ng serve
the demo will be up at localhost:4200
Please see CHANGE_LOG.MD for the updates.
The library is inspired by and based on the work from ng-device-detector . Also used a typescript wrapper of the amazing work in ReTree for regex based needs and an Angular2 Library Creator boilerplate to get the work started fast. I.e. Generator Angular2 library.
If you like the library and want to support, you can do it by buying me a coffee at
FAQs
Unknown package
The npm package ngx-device-detector receives a total of 158,397 weekly downloads. As such, ngx-device-detector popularity was classified as popular.
We found that ngx-device-detector demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.