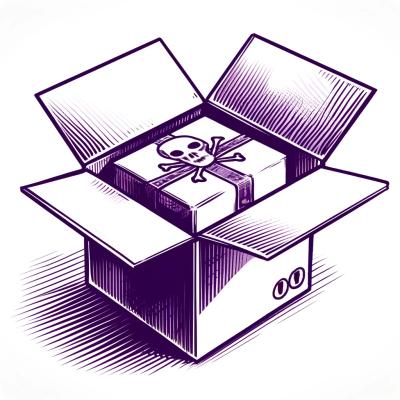
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
ngx-indexed-db
Advanced tools
ngx-indexed-db
is a service (CSR & SSR) that wraps IndexedDB database in an Angular service combined with the power of observables.
$ npm install ngx-indexed-db
OR
$ yarn add ngx-indexed-db
Import the NgxIndexedDBModule
and initiate it:
import { NgxIndexedDBModule } from 'ngx-indexed-db';
const dbConfig: DBConfig = {
name: 'MyDb',
version: 1,
objectStoresMeta: [{
store: 'people',
storeConfig: { keyPath: 'id', autoIncrement: true },
storeSchema: [
{ name: 'name', keypath: 'name', options: { unique: false } },
{ name: 'email', keypath: 'email', options: { unique: false } }
]
}]
};
@NgModule({
...
imports: [
...
NgxIndexedDBModule.forRoot(dbConfig)
],
...
})
Use provideIndexedDb
and set it up:
import { provideIndexedDb, DBConfig } from 'ngx-indexed-db';
const dbConfig: DBConfig = {
name: 'MyDb',
version: 1,
objectStoresMeta: [{
store: 'people',
storeConfig: { keyPath: 'id', autoIncrement: true },
storeSchema: [
{ name: 'name', keypath: 'name', options: { unique: false } },
{ name: 'email', keypath: 'email', options: { unique: false } }
]
}]
};
const appConfig: ApplicationConfig = {
providers: [...,provideIndexedDb(dbConfig),...]
}
OR
@NgModule({
...
providers:[
...
provideIndexedDb(dbConfig)
],
...
})
Starting from version 19.2.0, ngx-indexed-db
fully supports Server-Side Rendering (SSR). This enhancement prevents issues related to the absence of window.indexedDB
in server environments.
Additionally, you can provide a custom implementation of IndexedDB using an injection token. This allows greater flexibility, especially when mocking IndexedDB for testing or in non-browser environments (like SSR).
const SERVER_INDEXED_DB = new InjectionToken<IDBFactory>('Server Indexed Db');
import { NgxIndexedDBModule, DBConfig } from 'ngx-indexed-db';
// Ahead of time compiles requires an exported function for factories
export function migrationFactory() {
// The animal table was added with version 2 but none of the existing tables or data needed
// to be modified so a migrator for that version is not included.
return {
1: (db, transaction) => {
const store = transaction.objectStore('people');
store.createIndex('country', 'country', { unique: false });
},
3: (db, transaction) => {
const store = transaction.objectStore('people');
store.createIndex('age', 'age', { unique: false });
}
};
}
const dbConfig: DBConfig = {
name: 'MyDb',
version: 3,
objectStoresMeta: [{
store: 'people',
storeConfig: { keyPath: 'id', autoIncrement: true },
storeSchema: [
{ name: 'name', keypath: 'name', options: { unique: false } },
{ name: 'email', keypath: 'email', options: { unique: false } }
]
}, {
// animals added in version 2
store: 'animals',
storeConfig: { keyPath: 'id', autoIncrement: true },
storeSchema: [
{ name: 'name', keypath: 'name', options: { unique: true } },
]
}],
// provide the migration factory to the DBConfig
migrationFactory
};
@NgModule({
...
imports: [
...
NgxIndexedDBModule.forRoot(dbConfig)
],
...
})
Import and inject the service:
import { NgxIndexedDBService } from 'ngx-indexed-db';
...
export class AppComponent {
constructor(private dbService: NgxIndexedDBService){
}
}
We cover several common methods used to work with the IndexedDB
Adds new entry in the store and returns item added
It publishes in the observable the key value of the entry
this.dbService
.add('people', {
name: `Bruce Wayne`,
email: `bruce@wayne.com`,
})
.subscribe((key) => {
console.log('key: ', key);
});
In the previous example I'm using undefined as the key because the key is configured in the objectStore as auto-generated.
Adds new entries in the store and returns its key
this.dbService
.bulkAdd('people', [
{
name: `charles number ${Math.random() * 10}`,
email: `email number ${Math.random() * 10}`,
},
{
name: `charles number ${Math.random() * 10}`,
email: `email number ${Math.random() * 10}`,
},
])
.subscribe((result) => {
console.log('result: ', result);
});
Delete multiple items in the store
this.dbService.bulkDelete('people', [5, 6]).subscribe((result) => {
console.log('result: ', result);
});
Retrieve multiple entries in the store
this.dbService.bulkGet('people', [1, 3, 5]).subscribe((result) => {
console.log('results: ', result);
});
Adds or updates a record in store with the given value and key. Return all items present in the store
@Return The return value is an Observable with the primary key of the object that was last in given array
@error If the call to bulkPut fails the transaction will be aborted and previously inserted entities will be deleted
this.dbService.bulkPut('people', people).subscribe((result) => {
console.log('result: ', result);
});
Adds or updates a record in store with the given value and key. Return item updated
this.dbService
.update('people', {
id: 1,
email: 'luke@skywalker.com',
name: 'Luke Skywalker',
})
.subscribe((storeData) => {
console.log('storeData: ', storeData);
});
Returns entry by key.
this.dbService.getByKey('people', 1).subscribe((people) => {
console.log(people);
});
Return all elements from one store
this.dbService.getAll('people').subscribe((peoples) => {
console.log(peoples);
});
Returns entry by index.
this.dbService.getByIndex('people', 'name', 'Dave').subscribe((people) => {
console.log(people);
});
Allows to crate a new object store ad-hoc
const storeSchema: ObjectStoreMeta = {
store: 'people',
storeConfig: { keyPath: 'id', autoIncrement: true },
storeSchema: [
{ name: 'name', keypath: 'name', options: { unique: false } },
{ name: 'email', keypath: 'email', options: { unique: false } },
],
};
this.dbService.createObjectStore(storeSchema);
Returns the number of rows in a store.
this.dbService.count('people').subscribe((peopleCount) => {
console.log(peopleCount);
});
Delete the store by name, return true or false.
this.dbService.deleteObjectStore(this.storneNameToDelete);
Returns all items from the store after delete.
this.dbService.delete('people', 3).subscribe((allPeople) => {
console.log('all people:', allPeople);
});
Returns true if the delete completes successfully.
this.dbService.deleteByKey('people', 3).subscribe((status) => {
console.log('Deleted?:', status);
});
Returns the open cursor event
next
this.dbService.openCursor('people', IDBKeyRange.bound("A", "F")).subscribe((evt) => {
const cursor = (evt.target as IDBOpenDBRequest).result as unknown as IDBCursorWithValue;
if(cursor) {
console.log(cursor.value);
cursor.continue();
} else {
console.log('Entries all displayed.');
}
});
Open a cursor by index filter.
next
readonly
this.dbService.openCursorByIndex('people', 'name', IDBKeyRange.only('john')).subscribe((evt) => {
const cursor = (evt.target as IDBOpenDBRequest).result as unknown as IDBCursorWithValue;
if(cursor) {
console.log(cursor.value);
cursor.continue();
} else {
console.log('Entries all displayed.');
}
});
Returns all items by an index.
this.dbService.getAllByIndex('people', 'name', IDBKeyRange.only('john')).subscribe((allPeopleByIndex) => {
console.log('All: ', allPeopleByIndex);
});
Returns the current database version.
this.dbService.getDatabaseVersion().pipe(
tap(response => console.log('Versione database => ', response)),
catchError(err => {
console.error('Error recover version => ', err);
return throwError(err);
})
).subscribe();
Returns true if successfully delete all entries from the store.
this.dbService.clear('people').subscribe((successDeleted) => {
console.log('success? ', successDeleted);
});
Returns true if successfully delete the DB.
this.dbService.deleteDatabase().subscribe((deleted) => {
console.log('Database deleted successfully: ', deleted);
});
Returns all object store names.
this.dbService.getAllObjectStoreNames().subscribe((storeNames) => {
console.log('storeNames: ', storeNames);
});
Released under the terms of the MIT License.
FAQs
Angular wrapper to IndexedDB database.
The npm package ngx-indexed-db receives a total of 12,082 weekly downloads. As such, ngx-indexed-db popularity was classified as popular.
We found that ngx-indexed-db demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.