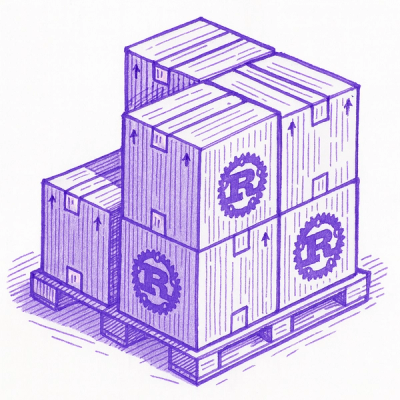
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
ngx-uploader
Advanced tools
Angular 2+ File Uploader
Do not open issues for general support questions as we want to keep GitHub issues for bug reports and feature requests. You've got much better chances of getting your question answered on Stack Overflow where the questions should be tagged with tag ngx-uploader
.
To save your and our time, we will systematically close all issues that are requests for general support and redirect people to Stack Overflow.
ngx-uploader
module as dependency to your project.npm install ngx-uploader --save
NgxUploaderModule
into your main AppModule or in module where you will use it.// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { NgxUploaderModule } from 'ngx-uploader';
@NgModule({
imports: [BrowserModule, NgxUploaderModule],
declarations: [AppComponent]
})
export class AppModule {}
or include NgxUploaderModule
into your SharedModule. This could be usefull if your project has nested Modules.
// shared.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { NgxUploaderModule } from 'ngx-uploader';
...
@NgModule({
imports: [
CommonModule,
NgxUploaderModule,
...
],
exports: [
CommonModule,
NgxUploaderModule,
...
],
...
})
export class SharedModule {
}
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { SharedModule } from './shared.module';
@NgModule({
imports: [BrowserModule, SharedModule],
declarations: [AppComponent]
})
export class AppModule {}
export interface UploaderOptions {
concurrency: number; // number of files uploaded at the same time
allowedContentTypes?: string[]; // content types allowed (default *)
maxUploads?: number; // max number of files the user can upload
maxFileSize?: number; // max size of the file in bytes the user can upload
}
export interface UploadProgress {
status: UploadStatus; // current status of upload for specific file (Queue | Uploading | Done | Canceled)
data?: {
percentage: number; // percentage of upload already completed
speed: number; // current upload speed per second in bytes
speedHuman: string; // current upload speed per second in human readable form,
eta: number; // estimated time remaining in seconds
etaHuman: string; // estimated time remaining in human readable format
};
}
export interface UploadFile {
id: string; // unique id of uploaded file instance
fileIndex: number; // fileIndex in internal ngx-uploader array of files
lastModifiedDate: Date; // last modify date of the file (Date object)
name: string; // original name of the file
size: number; // size of the file in bytes
type: string; // mime type of the file
form: FormData; // FormData object (you can append extra data per file, to this object)
progress: UploadProgress;
response?: any; // response when upload is done (parsed JSON or string)
responseStatus?: number; // response status code when upload is done
responseHeaders?: { [key: string]: string }; // response headers when upload is done
}
// output events emitted by ngx-uploader
export interface UploadOutput {
type:
| 'addedToQueue'
| 'allAddedToQueue'
| 'uploading'
| 'done'
| 'removed'
| 'start'
| 'cancelled'
| 'dragOver'
| 'dragOut'
| 'drop';
file?: UploadFile;
nativeFile?: File; // native javascript File object, can be used to process uploaded files in other libraries
}
// input events that user can emit to ngx-uploader
export interface UploadInput {
type: 'uploadAll' | 'uploadFile' | 'cancel' | 'cancelAll' | 'remove' | 'removeAll';
url?: string; // URL to upload file to
method?: string; // method (POST | PUT)
id?: string; // unique id of uploaded file
fieldName?: string; // field name (default 'file')
fileIndex?: number; // fileIndex in internal ngx-uploader array of files
file?: UploadFile; // uploading file
data?: { [key: string]: string | Blob }; // custom data sent with the file
headers?: { [key: string]: string }; // custom headers
includeWebKitFormBoundary?: boolean; // If false, only the file is send trough xhr.send (WebKitFormBoundary is omit)
concurrency?: number; // concurrency of how many files can be uploaded in parallel (default is 0 which means unlimited)
withCredentials?: boolean; // apply withCredentials option
}
With version 4.2.1 we've introduced restrictions for Content-Types. To not break the behaviour of previous releases, there are no restrictions by default at all.
Look at app-home.component.ts for an example of Content-Type restriction.
If you want to toast a message for the rejected file, add this to your onUploadOutput method.
onUploadOutput(output: UploadOutput): void {
....
} else if (output.type === 'rejected' && typeof output.file !== 'undefined') {
// console.log(output.file.name + ' rejected');
}
...
If you have to upload files with Token Authorization, you can set the header in startUpload as follows.
startUpload(): void {
let token = this.myToken; // <---- get token
const event: UploadInput = {
type: 'uploadAll',
url: 'http://ngx-uploader.com/upload',
method: 'POST',
headers: { 'Authorization': 'JWT ' + token }, // <---- set headers
data: { foo: 'bar' },
includeWebKitFormBoundary: true // <---- set WebKitFormBoundary
};
this.uploadInput.emit(event);
}
You can always run working example by cloning this repository, building project with yarn build:prod
and running server with node ./dist-app/api/index.js
.
import { Component, EventEmitter } from '@angular/core';
import { UploadOutput, UploadInput, UploadFile, humanizeBytes, UploaderOptions } from 'ngx-uploader';
@Component({
selector: 'app-home',
templateUrl: 'app-home.component.html'
})
export class AppHomeComponent {
options: UploaderOptions;
formData: FormData;
files: UploadFile[];
uploadInput: EventEmitter<UploadInput>;
humanizeBytes: Function;
dragOver: boolean;
constructor() {
this.options = { concurrency: 1, maxUploads: 3, maxFileSize: 1000000 };
this.files = []; // local uploading files array
this.uploadInput = new EventEmitter<UploadInput>(); // input events, we use this to emit data to ngx-uploader
this.humanizeBytes = humanizeBytes;
}
onUploadOutput(output: UploadOutput): void {
switch (output.type) {
case 'allAddedToQueue':
// uncomment this if you want to auto upload files when added
// const event: UploadInput = {
// type: 'uploadAll',
// url: '/upload',
// method: 'POST',
// data: { foo: 'bar' }
// };
// this.uploadInput.emit(event);
break;
case 'addedToQueue':
if (typeof output.file !== 'undefined') {
this.files.push(output.file);
}
break;
case 'uploading':
if (typeof output.file !== 'undefined') {
// update current data in files array for uploading file
const index = this.files.findIndex(file => typeof output.file !== 'undefined' && file.id === output.file.id);
this.files[index] = output.file;
}
break;
case 'removed':
// remove file from array when removed
this.files = this.files.filter((file: UploadFile) => file !== output.file);
break;
case 'dragOver':
this.dragOver = true;
break;
case 'dragOut':
case 'drop':
this.dragOver = false;
break;
case 'done':
// The file is downloaded
break;
}
}
startUpload(): void {
const event: UploadInput = {
type: 'uploadAll',
url: 'http://ngx-uploader.com/upload',
method: 'POST',
data: { foo: 'bar' }
};
this.uploadInput.emit(event);
}
cancelUpload(id: string): void {
this.uploadInput.emit({ type: 'cancel', id: id });
}
removeFile(id: string): void {
this.uploadInput.emit({ type: 'remove', id: id });
}
removeAllFiles(): void {
this.uploadInput.emit({ type: 'removeAll' });
}
}
For whole template code please check here.
<div
class="drop-container"
ngFileDrop
[options]="options"
(uploadOutput)="onUploadOutput($event)"
[uploadInput]="uploadInput"
[ngClass]="{ 'is-drop-over': dragOver }"
>
<h1>Drag & Drop</h1>
</div>
<label class="upload-button">
<input
type="file"
ngFileSelect
[options]="options"
(uploadOutput)="onUploadOutput($event)"
[uploadInput]="uploadInput"
multiple
/>
or choose file(s)
</label>
<button type="button" class="start-upload-btn" (click)="startUpload()">Start Upload</button>
MIT
FAQs
Angular 2+ File Uploader
We found that ngx-uploader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.