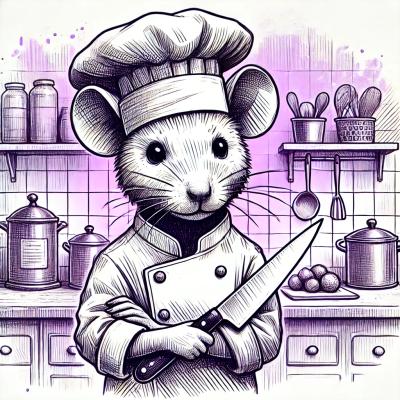
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
A simple Promise-based JavaScript library with a built-in dialog manager for building Telegram bots
Ninebot is a simple Promise-based JavaScript library with a built-in dialog manager. This library allows you to build your own Telegram bot faster and easier.
To build complicated ramified dialog you should to design all dialog branches and nodes.
For example, we need to send user different messages depending on his languages. Let's write a simple algorithm on pseudocode
language = %language%
if language = 'Human'
send 'Hello'
ask %name%
send 'Hello %name%'
// Some instructions here
if language = 'Martian'
send 'Sorry, we do not want to communicate with aliens'
This is a simple dialog which we can realize by N nested if ... else ...
, but what about declaratively description
of every dialog node? Let's transform previous algorithm
// Start node (entry point)
name: 'start'
message: 'Select language'
content:
'Human'
'Martian'
conditions:
'Human' => 'proceed'
'Martian' => 'exit'
// Proceed node
name: 'proceed'
message: 'What is your name?'
conditions:
%name is text% => 'next'
// Exit node
name: 'exit'
message: 'Sorry, we do not want to communicate with aliens'
In result, we have an array of nodes, and can easily switch between them depending on the current conditions.
Note: This guide implies that you have already created a bot and received a token.
Create a blank project and install a package via npm
or yarn
:
# npm
npm install --save ninebot
# yarn
yarn install ninebot
Then create a index.js
file and connect dependencies
const Ninebot = require('ninebot');
Then create a class which extends a Ninebot
class
class MyBot extends Ninebot {
// Great!
}
In constructor()
you can initialize your global class entities. Don't forget super()
!
class MyBot extends Ninebot {
constructor() {
super();
// Initialize your global entities here
}
}
In state
of your class you can store your data and update them to control dialog nodes data.
Dialog nodes are declaratively defined in update
function in JSON-like format:
{
name: 'node_name',
conditions: [
type: 'text', // Optional parameter [ text | photo ] to tell the bot what type of content to expect. Default value is
'text'
regExp: /.+/, // Regular expression to test input string (incoming message)
next: 'next_node_name', // Next dialog node name if regExp test is passed
callback: callback_fn // Optional. Callback function which called at the moment of transition to 'next_node_name'
],
reply: {
text: 'Here\'s a text which bot sends at the moment of transition to this node'
options: {
// Some addition options, like keyboard markup, you can find it in full docs
}
}
}
Now we can realize previous algorithm
const token = '{Your Telegram bot token}';
class MyBot extends Ninebot {
constructor(_token = token) {
super(_token);
// Initialize your global entities here
}
update() {
return ([
{
name: 'before',
conditions: [
{
type: 'text',
regExp: /\/start/,
next: 'start'
}
],
reply: { text: 'Enter /start' }
},
{
name: 'start',
conditions: [
{
type: 'text',
regExp: /Human/,
next: 'proceed'
},
{
type: 'text',
regExp: /Martian/,
next: 'exit'
}
],
reply: {
text: 'Select language',
options: {
reply_markup: {
keyboard: [
['Human', 'Martian']
],
resize_keyboard: true, // Set small buttons
one_time_keyboard: true // Hide keyboard after selecting language
}
}
}
},
{
name: 'proceed',
conditions: [
{
type: 'text',
regExp: /./,
next: 'next' // Some next node
}
],
reply: { text: 'What is your name?' }
},
{
name: 'next',
conditions: [{ regExp: /./, next: 'before' }],
reply: { text: 'Print any string' }
},
{
name: 'exit',
conditions: [
{
type: 'text',
regExp: /./,
next: 'start' // Loop the dialog
}
],
reply: { text: 'Sorry, we do not want to communicate with aliens' }
}
]);
}
}
Now that we have a simple bot logic, we can go to start a simple server to serve this bot.
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
const ADDRESS = '127.0.0.1'; //localhost
const PORT = 33459;
const BOT_PATH = '/bot'; // Pathname where Telegram will send messages to bot
const server = app.listen(process.env.PORT, ADDRESS, () => {
const host = server.address().address;
const port = server.address().port;
console.log('Web server started at http://%s:%s', host, port);
});
app.post(BOT_PATH, (req, res) => {
// Here the received data will be sent to the bot for processing
});
To identify the user, just use req.body.chat_id
- this is a unique chat ID of every Telegram user. To get data from
message to bot, we can use req.body.nessage
.
// Initializing code
app.post(BOT_PATH, (req, res) => {
res.sendStatus(200); // Send 200 OK to Telegram to confirm receipt of the message
const chat_id = req.body.message.chat.id;
const message = req.body.message;
});
Now initizlize our bot MyBot
const myBot = new MyBot(token);
A couple more lines and we can receive messages
app.post(BOT_PATH, (req, res) => {
res.sendStatus(200); // Send 200 OK to Telegram to confirm receipt of the message
const chat_id = req.body.message.chat.id;
const message = req.body.message;
// Don't forget to write chat_id to state
if (!myBot.state.chat_id) {
myBot.setState({ chat_id: chat_id });
}
myBot.runDialog(message);
});
If you want to start your bot locally, you should add some manipulations.
onReady()
function set the tunnelconst localtunnel = require('localtunnel');
class MyBot extends Ninebot {
onReady() {
this.dialogManager.setNode('before');
console.log('Setting local tunnel');
localtunnel(PORT, {}, (error, tunnel) => {
if (error) {
throw error;
}
console.log(`Url: ${tunnel.url}`);
this.bot.setWebhook(`${tunnel.url}${BOT_PATH}`).then(() => {
console.log('Webhook was set - bot is ready');
}).catch((error) => {
console.error(error);
});
});
}
}
Note:
onReady()
function is similar tocomponentDidMount()
in React.js
Great! Your first ninebot app is ready!
FAQs
A simple Promise-based JavaScript library with a built-in dialog manager for building Telegram bots
The npm package ninebot receives a total of 0 weekly downloads. As such, ninebot popularity was classified as not popular.
We found that ninebot demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.