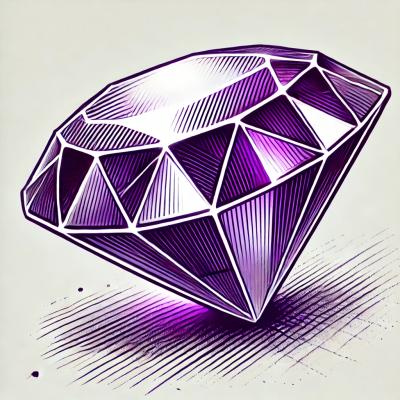
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
The node-dir package is a utility library for Node.js that provides methods to read directories recursively, read files within directories, and perform other directory and file system related operations. It is useful for applications that need to handle file system operations extensively.
Reading all files in a directory recursively
This feature allows you to list all files within a directory and its subdirectories. The function `dir.files` takes a directory path and a callback function that receives an array of file paths.
const dir = require('node-dir');
dir.files('/path/to/dir', function(err, files) {
if (err) throw err;
console.log(files);
});
Reading all subdirectories within a directory
This function lists all subdirectories within a specified directory. It is useful for applications that need to analyze or manipulate directory structures.
const dir = require('node-dir');
dir.subdirs('/path/to/dir', function(err, subDirs) {
if (err) throw err;
console.log(subDirs);
});
Reading content of files in a directory
This method reads the content of each file in a directory sequentially. It provides the file content to a callback function and proceeds to the next file when the `next` function is called.
const dir = require('node-dir');
dir.readFiles('/path/to/dir', function(err, content, next) {
if (err) throw err;
console.log(content);
next();
});
fs-extra is a package that builds on the native 'fs' module, providing additional methods and simplifying file system operations. It includes methods similar to node-dir but also offers file copying, moving, and more comprehensive error handling. It is generally more robust than node-dir.
glob is a package that allows pattern matching for filenames. It can be used to find files in a directory that match a given pattern, which is somewhat similar to listing files with node-dir but with more flexibility in file selection based on patterns.
recursive-readdir is a minimalistic package specifically focused on recursively reading directories. It is similar to the recursive file listing feature of node-dir but is lighter and has fewer features, focusing only on reading directories.
A small node.js module to provide some convenience methods for asynchronous, non-blocking, recursive directory operations like being able to get an array of all files, subdirectories, or both (with the option to either combine or separate the results), and for sequentially reading and processing the contents all files in a directory recursively, optionally firing a callback when finished.
For the sake of brevity, assume that the following line of code precedes all of the examples.
var dir = require('node-dir');
Sequentially read the content of each file in a directory, passing the contents to a callback, optionally calling a finished callback when complete. The options and finishedCallback arguments are not required.
Valid options are:
dir.readFiles(__dirname,
function(err, content, next) {
if (err) throw err;
console.log('content:', content);
next();
},
function(err, files){
if (err) throw err;
console.log('finished reading files:',files);
});
// match only filenames with a .txt extension and that don't start with a `.´
dir.readFiles(__dirname, {
match: /.txt$/,
exclude: /^\./
}, function(err, content, next) {
if (err) throw err;
console.log('content:', content);
next();
},
function(err, files){
if (err) throw err;
console.log('finished reading files:',files);
});
// the callback for each file can optionally have a filename argument as its 3rd parameter
// and the finishedCallback argument is optional, e.g.
dir.readFiles(__dirname, function(err, content, filename, next) {
console.log('processing content of file', filename);
next();
});
Asynchronously iterate the files of a directory and its subdirectories and pass an array of file paths to a callback.
dir.files(__dirname, function(err, files) {
if (err) throw err;
console.log(files);
});
Asynchronously iterate the subdirectories of a directory and its subdirectories and pass an array of directory paths to a callback.
dir.subdirs(__dirname, function(err, subdirs) {
if (err) throw err;
console.log(subdirs);
});
Asynchronously iterate the subdirectories of a directory and its subdirectories and pass an array of both file and directory paths to a callback.
Separated into two distinct arrays (paths.files and paths.dirs)
dir.paths(__dirname, function(err, paths) {
if (err) throw err;
console.log('files:\n',paths.files);
console.log('subdirs:\n', paths.dirs);
});
Combined in a single array (convenience method for concatenation of the above)
dir.paths(__dirname, true, function(err, paths) {
if (err) throw err;
console.log('paths:\n',paths);
});
MIT licensed (See LICENSE.txt)
FAQs
asynchronous file and directory operations for Node.js
The npm package node-dir receives a total of 2,502,620 weekly downloads. As such, node-dir popularity was classified as popular.
We found that node-dir demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.