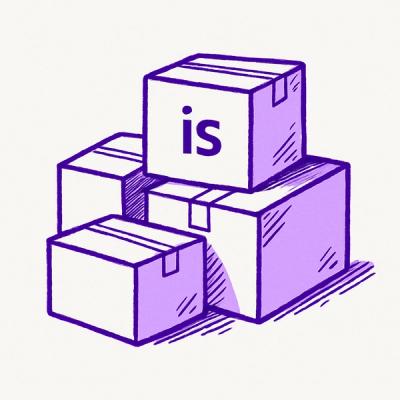
Security News
npm βisβ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
node-emoji
Advanced tools
The node-emoji package provides a simple way to add Unicode emoji support to your Node.js applications. It allows you to easily include emoji characters in your strings and manipulate them as needed.
Get emoji by name
Retrieve an emoji character using its name (alias).
const emoji = require('node-emoji');
console.log(emoji.get('coffee')); // outputs the coffee emoji
Find emoji in a string
Search for and return information about an emoji within a string.
const emoji = require('node-emoji');
console.log(emoji.find('I :heart: node-emoji!')); // finds and returns the heart emoji object
Replace emoji names with characters in a string
Convert emoji aliases in a string to their corresponding emoji characters.
const emoji = require('node-emoji');
console.log(emoji.emojify('I :heart: node-emoji!')); // replaces the :heart: alias with the actual heart emoji character
Replace characters with emoji names in a string
Convert emoji characters in a string to their corresponding emoji aliases.
const emoji = require('node-emoji');
console.log(emoji.unemojify('I β€οΈ node-emoji!')); // replaces the heart emoji character with its alias :heart:
Random emoji
Get a random emoji object, which includes the emoji character and its alias.
const emoji = require('node-emoji');
console.log(emoji.random()); // returns a random emoji object
Emojione is a package that provides emoji conversion and supports a wide range of emoji characters. It is similar to node-emoji but also includes additional features such as emoji shortcodes, ASCII conversions, and sprite generation.
Emoji-js is a package that allows for conversion between Unicode characters and emoji images. It differs from node-emoji in that it can convert emoji characters into images, which can be useful for web applications that need to support a wide range of devices and platforms.
Twemoji is a Twitter open-source project that provides emoji support across different platforms. It offers emoji parsing and image conversion, similar to emoji-js, and focuses on consistent emoji rendering across all platforms.
Friendly emoji lookups and parsing utilities for Node.js. π
node-emoji
provides a fun, straightforward interface on top of the following excellent libraries:
emojilib
: provides a list of emojis and keyword search on top of itskin-tone
: parses out base emojis from skin tonesnpm install node-emoji
This is the new 2.0 release of node-emoji, supporting ESM, new emoji and a new API.
If you want to use the old version, please check out the legacy branch.
import * as emoji from 'node-emoji'
emoji.emojify('I :heart: :coffee:!') // 'I β€οΈ βοΈ!'
emoji.find('heart') // { emoji: 'β€', name: 'heart' }
emoji.find('β€οΈ') // { emoji: 'β€', name: 'heart' }
emoji.get('unicorn') // π¦
emoji.get(':unicorn:') // π¦
emoji.has(':pizza:') // true
emoji.has('π') // true
emoji.has('unknown') // false
emoji.random() // { name: 'house', emoji: 'π ' }
emoji.replace('I β€οΈ coffee!', 'love', { preserveSpaces: true }) // 'I love coffee!'
emoji.search(':uni:') // [ { emoji: 'π¦', name: 'unicorn' }, ... ]
emoji.strip('I β€οΈ coffee!') // 'I coffee!'
emoji.unemojify('π for π') // ':pizza: for :dancer:'
emoji.which('π¦') // 'unicorn'
Parse all markdown-encoded emojis in a string.
Parameters:
input
(string
): The input string containing the markdown-encoding emojis.options
(optional):
fallback
(string
; default: ""
): The string to fallback to if an emoji was not found.format
(() => (emoji: string, part: string, string: string) => string
; default: value => value
): Add a middleware layer to modify each matched emoji after parsing.import * as emoji from 'node-emoji'
console.log(emoji.emojify('The :unicorn: is a fictitious animal.'))
// 'The π¦ is a fictitious animal.'
Get the name and character of an emoji.
Parameters:
emoji
(string
): The emoji to get the data of.import * as emoji from 'node-emoji'
console.log(emoji.find('π¦'))
// { name: 'unicorn', emoji: 'π¦' }
Get an emoji from an emoji name.
Parameters:
name
(string
): The name of the emoji to get.import * as emoji from 'node-emoji'
console.log(emoji.get('unicorn'))
// 'π¦'
Check if this library supports a specific emoji.
Parameters:
emoji
(string
): The emoji to check.import * as emoji from 'node-emoji'
console.log(emoji.has('π¦'))
// true
Get a random emoji.
import * as emoji from 'node-emoji'
console.log(emoji.random())
// { name: 'unicorn', emoji: 'π¦' }
Replace the emojis in a string.
Parameters:
input
(string
): The input string.replacement
(string | (emoji: string, index: number, string: string) => string
): The character to replace the emoji with.
Can be either a string or a callback that returns a string.import * as emoji from 'node-emoji'
console.log(emoji.replace('The π¦ is a fictitious animal.', 'unicorn'))
// 'The unicorn is a fictitious animal.'
Search for emojis containing the provided name in their name.
Parameters:
keyword
(string
): The keyword to search for.import * as emoji from 'node-emoji'
console.log(emoji.search('honey'))
// [ { name: 'honeybee', emoji: 'π' }, { name: 'honey_pot', emoji: 'π―' } ]
Remove all of the emojis from a string.
Parameters:
input
(string
): The input string to strip the emojis from.
options
(optional):
preserveSpaces
(boolean
): Whether to keep the extra space after a stripped emoji.import * as emoji from 'node-emoji'
console.log(emoji.strip('π¦ The unicorn is a fictitious animal.'))
// 'The unicorn is a fictitious animal.'
console.log(
emoji.strip('π¦ The unicorn is a fictitious animal.', {
preserveSpaces: true,
}),
)
// ' The unicorn is a fictitious animal.'
Convert all emojis in a string to their markdown-encoded counterparts.
Parameters:
input
(string
): The input string containing the emojis.import * as emoji from 'node-emoji'
console.log(emoji.unemojify('The π¦ is a fictitious animal.'))
// 'The :unicorn: is a fictitious animal.'
Get an emoji name from an emoji.
Parameters:
emoji
(string
): The emoji to get the name of.options
(optional):
markdown
(boolean
; default: false
): Whether to return a ":emoji:"
string instead of "emoji"
import * as emoji from 'node-emoji'
console.log(emoji.which('π¦'))
// 'unicorn'
...to Anand Chowdhary (@AnandChowdhary) and his company Pabio for sponsoring this project via GitHub Sponsors!
FAQs
Friendly emoji lookups and parsing utilities for Node.js. π
The npm package node-emoji receives a total of 7,068,352 weekly downloads. As such, node-emoji popularity was classified as popular.
We found that node-emoji demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.