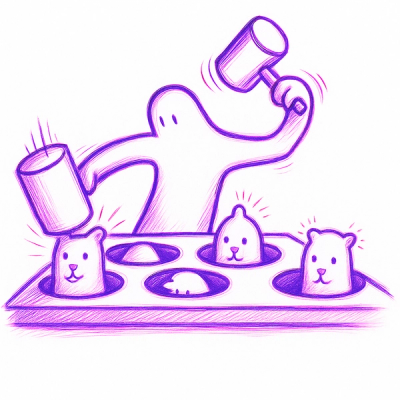
Research
/Security News
Contagious Interview Campaign Escalates With 67 Malicious npm Packages and New Malware Loader
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
Node bindings to the libgit2 project.
Stable: 0.2.0
Maintained by Tim Branyen @tbranyen, Michael Robinson @codeofinterest, John Haley @johnhaley81, Max Korp @maxkorp, and Nick Kallen @nk with help from awesome contributors!
NodeGit will work on most systems out-of-the-box without any native dependencies.
npm install nodegit
If you encounter problems while installing, you should try the Building from source instructions below.
Minimum dependencies:
If you wish to help contribute to nodegit it is useful to build locally.
# Fetch this project.
git clone git://github.com/nodegit/nodegit.git
# Enter the repository.
cd nodegit
# Installs the template engine, run the code generation script, and build.
npm install
If you encounter errors, you most likely have not configured the dependencies correctly.
Using APT in Ubuntu:
sudo apt-get install build-essential
Using Pacman in Arch Linux:
sudo pacman -S base-devel
You may have to add a build flag to the installation process to successfully install. Try first without, if the build fails, try again with the flag.
Allegedly the order in which you install Visual Studio could trigger this error.
npm install nodegit --msvs_version=2013
# Or whatever version you've installed.
var clone = require("./").Clone.clone;
// Clone a given repository into a specific folder.
clone("https://github.com/nodegit/nodegit", "tmp", null)
// Look up this known commit.
.then(function(repo) {
// Use a known commit sha from this repository.
return repo.getCommit("59b20b8d5c6ff8d09518454d4dd8b7b30f095ab5");
})
// Look up a specific file within that commit.
.then(function(commit) {
return commit.getEntry("README.md");
})
// Get the blob contents from the file.
.then(function(entry) {
// Patch the blob to contain a reference to the entry.
return entry.getBlob().then(function(blob) {
blob.entry = entry;
return blob;
});
})
// Display information about the blob.
.then(function(blob) {
// Show the name, sha, and filesize in byes.
console.log(blob.entry.name() + blob.entry.sha() + blob.size() + "b");
// Show a spacer.
console.log(Array(72).join("=") + "\n\n");
// Show the entire file.
console.log(String(blob));
})
.catch(function(err) { console.log(err); });
var open = require("nodegit").Repository.open;
// Open the repository directory.
open("tmp")
// Open the master branch.
.then(function(repo) {
return repo.getMasterCommit();
})
// Display information about commits on master.
.then(function(firstCommitOnMaster) {
// Create a new history event emitter.
var history = firstCommitOnMaster.history();
// Create a counter to only show up to 9 entries.
var count = 0;
// Listen for commit events from the history.
history.on("commit", function(commit) {
// Disregard commits past 9.
if (++count >= 9) {
return;
}
// Show the commit sha.
console.log("commit " + commit.sha());
// Store the author object.
var author = commit.author();
// Display author information.
console.log("Author:\t" + author.name() + " <", author.email() + ">");
// Show the commit date.
console.log("Date:\t" + commit.date());
// Give some space and show the message.
console.log("\n " + commit.message());
});
// Start emitting events.
history.start();
});
You will need to build locally before running the tests. See above.
npm test
The bump from 0.1.4 to 0.2.0 was a big one. Many things changed, see here: https://github.com/nodegit/nodegit/compare/refs/tags/0.1.4...0.2.0
This update is wholly and entirely a breaking one, and older versions won't be maintained. For the purpose of migration, perhaps the biggest point to make is that async methods can now use promises, rather than just taking callbacks. Additionally, lots of method and property names have changed.
<a name="v0-2-0" href="#v0-2-0">v0.2.0</a> (2014-11-25)
Closed issues:
Find some way to automatically generate a list of missing tests. #272
libgit2 creation methods have name collisions with internal V8 functions #271
Enums are still being manually assigned in javascript #268
We're using too many promise libraries #264
unable to resolve symbolic references #262
nodegit installation falls back when Python install dir contains spaces #261
Probe features #245
require('path').Repo.open(...) returns {} #241
RevWalk malloc error #239
OS X tests in Travis-CI #237
Fix RevWalk tests #236
Simple clone fails. #231
Create templates for remaining src and include files #230
Error: SSL is not supported by this copy of libgit2. #228
error while install nodegit latest version 0.1.4 #225
error while install nodegit latest version 0.1.4 #224
Did getReferences dissapear? #223
Again for #147 #218
Update documentation on nodegit.org #217
Stable = bump to 1.0 #215
Example on nodegit.com homepage is invalid #211
tree.diffWorkDir deprecated? #209
Abort on getRemotes #201
Generic Logging/Tracing mechanism #199
Repo#openIndex missing #197
Documentation on http://www.nodegit.org/ out of date #196
Remove extern "C" with 0.21 bump #193
CloneOptions documentation lacking #192
Webpage examples are not up to date #190
Automatically generate structs from types array #187
Error: connect ETIMEDOUT during install #179
TODO #177
Notes #176
Integration improvements. #171
Merged pull requests:
[WIP] Push example #288 (johnhaley81)
Add details-for-tree-entry #285 (johnhaley81)
update add-and-commit example to include new paths #283 (maxkorp)
Added osx for testing on Travis #281 (johnhaley81)
Added " around python path to help fix issues with spaces in path #280 (johnhaley81)
Tests for branch class #279 (johnhaley81)
[WIP] Update examples #276 (johnhaley81)
Added script to generate missing tests #274 (johnhaley81)
MSBUILD doesn't allow an array of size 0 #270 (johnhaley81)
voidcheck string pointers and reenable attr test #266 (maxkorp)
require --documentation flag to include text in idefs #265 (maxkorp)
Added ability for callbacks to poll promises for fulfillment value #260 (johnhaley81)
Generate nodegit from libgit2 docs and refactor descriptor #259 (johnhaley81)
Use Start-Process to start pageant.exe #254 (FeodorFitsner)
Added more git_cred methods #251 (johnhaley81)
Add revwalk.hide and revwalk.simplifyFirstParent #235 (tbranyen)
Add revwalk.hide and revwalk.simplifyFirstParent #234 (orderedlist)
Moved wrapper/copy out of include/src #233 (johnhaley81)
Removed ejs dependency #232 (johnhaley81)
WIP: Refactor source generation templates from EJS to Combyne #227 (tbranyen)
Test fixes #226 (johnhaley81)
Added new methods in checkout and repository #207 (tbranyen)
moved libgit2 gyp to separate dir #184 (deepak1556)
FAQs
Node.js libgit2 asynchronous native bindings
The npm package nodegit receives a total of 62,391 weekly downloads. As such, nodegit popularity was classified as popular.
We found that nodegit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.