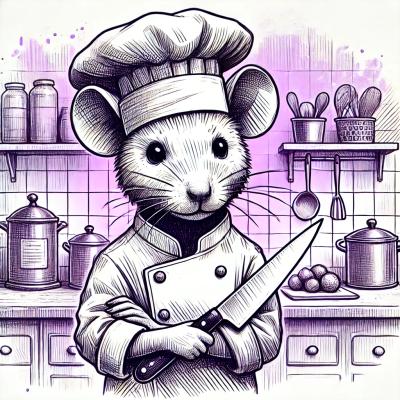
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
A JavaScript lib to efficiently estimate translation, scale, and/or rotation between two sets of 2D points. We have found it to be useful in graphics, user interfaces, multi-touch recognition, and eye tracker calibration. In general, you can apply nudged in any situation where you want to move a number of points based on a few sample points and optionally one fixed pivot point. See the image below for visual explanation.
Figure: Left: You have a set of points. Center: you known where three of them should be moved. Right: With nudged, based on the initial position of the three points and their target positions, you can estimate a transformation that nicely transforms all the rest of the points.
Mathematically speaking, nudged is a set of optimal least squares estimators for nonreflective similarity transformation matrices. Such transformations are affine transformations with translation, rotation, and/or uniform scaling, and without reflection or shearing. The estimation has time complexity of O(n), where n is the cardinality (size) of the point sets. In other words, nudged solves a 2D to 2D point set registration problem (alias Procrustes superimposition) in linear time. The algorithms and their efficiency are thoroughly described in a M.Sc. thesis Advanced algorithms for manipulating 2D objects on touch screens.
The development has been supported by Infant Cognition Laboratory at University of Tampere where it is used to correct eye tracking data.
Available also in Python.
To get a grip, play with the following demos.
The touch gesture demo takes the common pinch-zoom and rotate gestures a step further. Many multitouch apps allow you to scale and rotate with two fingers. However, usually the additional fingers are ignored. But what if one wants to use, say, both hands and all the fingers on a huge touchscreen?
For reference, the typical gesture demo implements similar demo with the popular Hammer.js touch gesture library. As you can experience, only the first two pointers are regarded for scaling and rotation.
The editor demo allows you to add domain and range points on a surface and explore how the points affect the transformation.
In this map viewer demo, nudged is used to recognize multi-touch gestures to scale, rotate, and translate a large image on HTML5 canvas.
With npm:
$ npm install nudged
Let domain
and range
be point sets before and after transformation as illustrated in the figure below:
> var domain = [[0,0], [2,0], [ 1,2]]
> var range = [[1,1], [1,3], [-1,2]]
Figure: Left: the domain. Center: the range. Right: the domain after transformation.
Compute an optimal transformation based on the points:
> var trans = nudged.estimate('TSR', domain, range)
Examine the transformation matrix:
> trans.getMatrix()
{ a: 0, c: -1, e: 1,
b: 1, d: 0, f: 1 }
> trans.getRotation()
1.5707... = π / 2
> trans.getScale()
1.0
> trans.getTranslation()
[1, 1]
Apply the transformation to other points:
> trans.transform([2,2])
[-1,3]
Inverse the transformation:
> var inv = trans.inverse()
> inv.transform([-1,3])
[2,2]
See API for more.
You can think the pivot point as a pin pushed through a paper. The pin keeps its location intact regardless of the transformation around it, as illustrated in the figure below.
Figure: Left: a black pivot point and the domain. Center: the range. Right: the pivot and the domain after transformation.
In the following example we estimate an optimal scaling and rotation around point [-1,0]
:
> var pivot = [-1,0]
> var domain = [[0,0], [2,0], [ 1,2]]
> var range = [[1,1], [1,3], [-1,2]]
> var pivotTrans = nudged.estimate('SR', domain, range, pivot)
If we now apply the transformation to the domain, we see that the result is close to the range. Also, if we apply it to the pivot, the point stays the same.
> pivotTrans.transform(domain)
[[-0.33, 0.77], [0.99, 2.33], [-1.22, 2.88]]
> pivotTrans.transform(pivot)
[-1,0]
Nudged API provides a class for nonreflective similarity transformations and 7 types of estimators, one for each combination of translation, scaling, and rotation. The ones without translation allow an optional fixed point.
Create a transformation that scales, rotates, and translates as specified.
Parameters:
The parameters are optional and default to the identity transformation.
Return a new nudged.Transform
instance.
Examples:
> var t0 = nudged.create()
> t0.transform([3, 1])
[3, 1]
> var t1 = nudged.create(2)
> t1.transform([3, 1])
[6, 2]
> var t2 = nudged.create(1, Math.PI / 2)
> t2.transform([3, 1])
[-1, 3]
> var t3 = nudged.create(1, 0, 20.2, 0)
> t3.transform([3, 1])
[23.2, 1]
Create a nudged.Transform
instance from an array created by nudged.Transform#toArray(). Together with nudged.Transform#toArray()
this method makes an easy serialization and deserialization to and from JSON possible.
> var t1 = nudged.create(1, 2, 3, 4)
> var arr = trans.toArray()
> var t2 = nudged.createFromArray(arr)
> t1.equals(t2)
true
Compute an optimal affine transformation from the domain to range points. The type of transformation is any combination of translation T
, scaling S
, and rotation R
, in this order. The transformations without translation allow an optional fixed pivot point.
Parameters:
'T'
, 'S'
, 'R'
, 'TS'
, 'TR'
, 'SR'
, 'TSR'
'S'
, 'R'
, and 'SR'
.The domain and range should have equal length. Different lengths are allowed but additional points in the longer array are ignored.
Return new nudged.Transform(...)
instance.
You can also call the estimators directly:
nudged.estimateT(domain, range)
nudged.estimateS(domain, range, pivot)
nudged.estimateR(domain, range, pivot)
nudged.estimateTS(domain, range)
nudged.estimateTR(domain, range)
nudged.estimateSR(domain, range, pivot)
nudged.estimateTSR(domain, range)
Contains the module version string identical to the version in package.json.
> nudged.version
'1.2.3'
A constructor for a nonreflective similarity transformation. You usually do not need to call it directly because both nudged.create(...)
and nudged.estimate(...)
create and return instances for you. Nevertheless, if you need to create one:
> var trans = new nudged.Transform(0.5, 0, 20, 0)
The nudged.Transform
instance is designed to be immutable.
Parameters s
, r
, tx
, and ty
define the elements of an augmented transformation matrix in the following manner:
| s -r tx |
| r s ty |
| 0 0 1 |
Note that s
and r
do not represent scaling and rotation but instead s = scalingFactor * Math.cos(rotationRads)
and r = scalingFactor * Math.sin(rotationRads)
. The parameters tx
and ty
represent horizontal and vertical translation after rotation.
A default instance of nudged.Transform
that represents the identity transformation i.e. transformation without an effect. You can use it in building new transformations:
> var trans = nudged.Transform.IDENTITY.scaleBy(0.6).rotateBy(0.3);
Elements of the internal transformation matrix. Direct use of these properties is not recommended.
> var t = nudged.create(2, Math.PI / 2, 10, 20)
> t.s
1.2246e-16
> t.r
2
> t.tx
10
> t.ty
20
Parameter tr
is an instance of nudged.Transform
.
Return true if the parameters of the two transformations are equal and false otherwise.
Get the transformation matrix in a format compatible with kld-affine.
Return an object with properties a
, b
, c
, d
, e
, and f
.
> trans.getMatrix()
{ a: 0.48, c: -0.52, e: 205.04,
b: 0.52, d: 0.48, f: 4.83 }
The properties represent the following matrix:
| a c e |
| b d f |
| 0 0 1 |
Get clockwise rotation from the positive x-axis.
Return rotation in radians.
Return scaling multiplier, e.g. 0.333
for a threefold shrink.
Return [tx, ty]
where tx
and ty
denotes movement along x-axis and y-axis accordingly.
Together with nudged.createFromArray(...)
this method makes an easy serialization and deserialization to and from JSON possible.
Return an array representation of the transformation: [s, r, tx, ty]
. Note that s
and r
do not represent scaling and rotation but elements of the matrix.
Apply the transform to a point or an array of points.
Parameter points
is an array of points [[x, y], ...]
or a single point [x, y]
.
Return an array of transformed points or single point if only a point was given. For example:
> trans.transform([1,1])
[2,2]
> trans.transform([[1,1]])
[[2,2]]
> trans.transform([[1,1], [2,3]])
[[2,2], [3,4]]
Return a new nudged.Transform
instance that is the inverse of the original transformation.
Throw an Error
instance if the transformation is singular and cannot be inversed. This occurs if the range points are all the same which forces the scale to drop to zero.
Return a new nudged.Transform
instance where the image of the original has been translated.
Parameter multiplier
is a number. Optional parameter pivot
is a point [x, y]
.
Return a new nudged.Transform
instance where the image of the original has been scaled.
The scaling is done around an optional pivot point that defaults to [0,0].
Parameter radians
is a number. Optional parameter pivot
is a point [x, y]
.
Return a new nudged.Transform
instance where the image of the original has been rotated.
The rotation is done around an optional pivot point that defaults to [0,0].
Parameter tr
is an instance of nudged.Transform
.
Return a new nudged.Transform
instance where the original transformation matrix is multiplied from the right with the transformation matrix of tr
.
The resulting transformation is equal to first transforming with tr
and then with the instance. More precisely, the image of the resulting transformation is the image of tr
transformed by the instance.
Run lint & unit tests:
$ npm run test
Build example apps:
$ npm run build:examples
Start local server to try out the examples:
$ npm start
Release:
$ git checkout -b release-7.7.7 development
$ git commit -a -m "Clean release 7.7.7"
$ git checkout master
$ git merge release-7.7.7
$ git push
$ git tag -a 7.7.7 -m "v7.7.7 Superb Name"
$ git push --tags
$ npm publish
$ git checkout development
[1.2.0] - 2016-11-22
Transform
serialization methods: createFromArray
, toArray
(#11)Transform
property documentation. (#10)nudged.create
.FAQs
Affine transformation estimator e.g. for multi-touch gestures and calibration
The npm package nudged receives a total of 109 weekly downloads. As such, nudged popularity was classified as not popular.
We found that nudged demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.