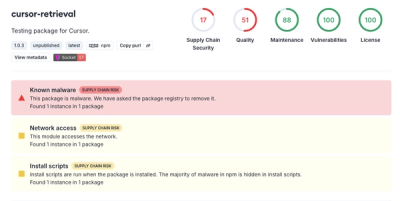
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
oauth-1.0a
Advanced tools
The oauth-1.0a npm package is a lightweight library for implementing OAuth 1.0a authentication in Node.js applications. It provides utilities for generating OAuth signatures, creating authorization headers, and handling the OAuth flow.
Generate OAuth Signature
This feature allows you to generate an OAuth signature for a given request. The code sample demonstrates how to create an OAuth instance, define the request data, and generate the signature using the consumer and token credentials.
const OAuth = require('oauth-1.0a');
const crypto = require('crypto');
const oauth = OAuth({
consumer: { key: 'your-consumer-key', secret: 'your-consumer-secret' },
signature_method: 'HMAC-SHA1',
hash_function(base_string, key) {
return crypto.createHmac('sha1', key).update(base_string).digest('base64');
}
});
const request_data = {
url: 'https://api.example.com/resource',
method: 'GET'
};
const token = {
key: 'your-access-token',
secret: 'your-token-secret'
};
const signature = oauth.authorize(request_data, token);
console.log(signature);
Create Authorization Header
This feature allows you to create an OAuth authorization header for a given request. The code sample demonstrates how to generate the authorization header using the OAuth instance and the request data.
const OAuth = require('oauth-1.0a');
const crypto = require('crypto');
const oauth = OAuth({
consumer: { key: 'your-consumer-key', secret: 'your-consumer-secret' },
signature_method: 'HMAC-SHA1',
hash_function(base_string, key) {
return crypto.createHmac('sha1', key).update(base_string).digest('base64');
}
});
const request_data = {
url: 'https://api.example.com/resource',
method: 'GET'
};
const token = {
key: 'your-access-token',
secret: 'your-token-secret'
};
const authorizationHeader = oauth.toHeader(oauth.authorize(request_data, token));
console.log(authorizationHeader);
Handle OAuth Flow
This feature demonstrates how to handle the OAuth flow to access a protected resource. The code sample shows how to generate the authorization header and make an authenticated request using the axios library.
const OAuth = require('oauth-1.0a');
const crypto = require('crypto');
const axios = require('axios');
const oauth = OAuth({
consumer: { key: 'your-consumer-key', secret: 'your-consumer-secret' },
signature_method: 'HMAC-SHA1',
hash_function(base_string, key) {
return crypto.createHmac('sha1', key).update(base_string).digest('base64');
}
});
async function getProtectedResource() {
const request_data = {
url: 'https://api.example.com/resource',
method: 'GET'
};
const token = {
key: 'your-access-token',
secret: 'your-token-secret'
};
const authorizationHeader = oauth.toHeader(oauth.authorize(request_data, token));
try {
const response = await axios.get(request_data.url, { headers: authorizationHeader });
console.log(response.data);
} catch (error) {
console.error(error);
}
}
getProtectedResource();
The 'oauth' package is a comprehensive library for OAuth 1.0 and OAuth 2.0 authentication. It provides a higher-level abstraction compared to oauth-1.0a and supports both OAuth versions, making it more versatile for different authentication needs.
The 'simple-oauth2' package is designed for OAuth 2.0 authentication and provides a simple and easy-to-use API for obtaining access tokens and making authenticated requests. It does not support OAuth 1.0a, so it is not a direct replacement for oauth-1.0a but is useful for OAuth 2.0 scenarios.
The 'passport-oauth1' package is a Passport strategy for OAuth 1.0a authentication. It integrates with the Passport.js authentication middleware, making it a good choice for applications that use Passport for managing authentication strategies.
[semaphore-url]: https://semaphoreci.com/ddo/oauth-1-0a)
OAuth 1.0a Request Authorization for Node and Browser
Send OAuth request with your favorite HTTP client (request, jQuery.ajax...)
No more headache about OAuth 1.0a's stuff or "oauth_consumer_key, oauth_nonce, oauth_signature...." parameters, just use your familiar HTTP client to send OAuth requests.
Tested on some popular OAuth 1.0a services:
var crypto = require('crypto');
...
var oauth = OAuth({
consumer: {
key: '<your consumer key>',
secret: '<your consumer secret>'
},
signature_method: 'HMAC-SHA1',
hash_function: function(base_string, key) {
return crypto.createHmac('sha1', key).update(base_string).digest('base64');
}
});
Get OAuth request data then you can use with your http client easily :)
oauth.authorize(request, token);
Or if you want to get as a header key-value data
oauth.toHeader(oauth_data);
From version 2.0.0
, crypto/hash stuff is separated.
oauth-1.0a
will use your hash_function
to sign.
var crypto = require('crypto');
function hash_function_sha1(base_string, key) {
return crypto.createHmac('sha1', key).update(base_string).digest('base64');
}
var oauth = OAuth({
consumer: {
key: '<your consumer key>',
secret: '<your consumer secret>'
},
signature_method: 'HMAC-SHA1',
hash_function: hash_function_sha1
});
crypto.createHmac('sha1', key).update(base_string).digest('base64');
crypto.createHmac('sha256', key).update(base_string).digest('base64');
using google CryptoJS
CryptoJS.HmacSHA1(base_string, key).toString(CryptoJS.enc.Base64);
CryptoJS.HmacSHA256(base_string, key).toString(CryptoJS.enc.Base64);
##Installation
###Node.js $ npm install oauth-1.0a --production
hash_function
.require('crypto')
will result in an error being thrown. function(base_string, key) return <string>
###Browser Download oauth-1.0a.js here
And also your crypto lib. For example CryptoJS
<!-- sha1 -->
<script src="http://crypto-js.googlecode.com/svn/tags/3.1.2/build/rollups/hmac-sha1.js"></script>
<!-- sha256 -->
<script src="http://crypto-js.googlecode.com/svn/tags/3.1.2/build/rollups/hmac-sha256.js"></script>
<script src="http://crypto-js.googlecode.com/svn/tags/3.1.2/build/components/enc-base64-min.js"></script>
<script src="oauth-1.0a.js"></script>
##Examples
###Work with request (Node.js)
Depencies
var request = require('request');
var OAuth = require('oauth-1.0a');
var crypto = require('crypto');
Init
var oauth = OAuth({
consumer: {
key: 'xvz1evFS4wEEPTGEFPHBog',
secret: 'kAcSOqF21Fu85e7zjz7ZN2U4ZRhfV3WpwPAoE3Z7kBw'
},
signature_method: 'HMAC-SHA1',
hash_function: function(base_string, key) {
return crypto.createHmac('sha1', key).update(base_string).digest('base64');
}
});
Your request data
var request_data = {
url: 'https://api.twitter.com/1/statuses/update.json?include_entities=true',
method: 'POST',
data: {
status: 'Hello Ladies + Gentlemen, a signed OAuth request!'
}
};
Your token (optional for some requests)
var token = {
key: '370773112-GmHxMAgYyLbNEtIKZeRNFsMKPR9EyMZeS9weJAEb',
secret: 'LswwdoUaIvS8ltyTt5jkRh4J50vUPVVHtR2YPi5kE'
};
Call a request
request({
url: request_data.url,
method: request_data.method,
form: oauth.authorize(request_data, token)
}, function(error, response, body) {
//process your data here
});
Or if you want to send OAuth data in request's header
request({
url: request_data.url,
method: request_data.method,
form: request_data.data,
headers: oauth.toHeader(oauth.authorize(request_data, token))
}, function(error, response, body) {
//process your data here
});
###Work with jQuery.ajax (Browser)
Caution: please make sure you understand what happen when use OAuth protocol at client side here
Init
var oauth = OAuth({
consumer: {
key: 'xvz1evFS4wEEPTGEFPHBog',
secret: 'kAcSOqF21Fu85e7zjz7ZN2U4ZRhfV3WpwPAoE3Z7kBw'
},
signature_method: 'HMAC-SHA1',
hash_function: function(base_string, key) {
return CryptoJS.HmacSHA1(base_string, key).toString(CryptoJS.enc.Base64);
}
});
Your request data
var request_data = {
url: 'https://api.twitter.com/1/statuses/update.json?include_entities=true',
method: 'POST',
data: {
status: 'Hello Ladies + Gentlemen, a signed OAuth request!'
}
};
Your token (optional for some requests)
var token = {
key: '370773112-GmHxMAgYyLbNEtIKZeRNFsMKPR9EyMZeS9weJAEb',
secret: 'LswwdoUaIvS8ltyTt5jkRh4J50vUPVVHtR2YPi5kE'
};
Call a request
$.ajax({
url: request_data.url,
type: request_data.method,
data: oauth.authorize(request_data, token)
}).done(function(data) {
//process your data here
});
Or if you want to send OAuth data in request's header
$.ajax({
url: request_data.url,
type: request_data.method,
data: request_data.data,
headers: oauth.toHeader(oauth.authorize(request_data, token))
}).done(function(data) {
//process your data here
});
##.authorize(/* options */)
String
String
default 'GET'
Object
any custom data you want to send with, including extra oauth option oauth_*
as oauth_callback, oauth_version...var request_data = {
url: 'https://bitbucket.org/api/1.0/oauth/request_token',
method: 'POST',
data: {
oauth_callback: 'http://www.ddo.me'
}
};
##.toHeader(/* signed data */)
convert signed data into headers
$.ajax({
url: request_data.url,
type: request_data.method,
data: request_data.data,
headers: oauth.toHeader(oauth.authorize(request_data, token))
}).done(function(data) {
//process your data here
});
##Init Options
var oauth = OAuth(/* options */);
consumer
: Object
Required
your consumer keys{
key: <your consumer key>,
secret: <your consumer secret>
}
signature_method
: String
default 'PLAINTEXT'
hash_function
: Function
if signature_method
= 'PLAINTEXT'
default return key
nonce_length
: Int
default 32
version
: String
default '1.0'
parameter_seperator
: String
for header only, default ', '
. Note that there is a space after ,
last_ampersand
: Bool
default true
. For some services if there is no Token Secret then no need &
at the end. Check oauth doc for more informationoauth_signature is set to the concatenated encoded values of the Consumer Secret and Token Secret, separated by a '&' character (ASCII code 38), even if either secret is empty
##Notes
Some OAuth requests without token use .authorize(request_data)
instead of .authorize(request_data, {})
Or just token key only .authorize(request_data, {key: 'xxxxx'})
Want easier? Take a look:
##Client Side Usage Caution
OAuth is based around allowing tools and websites to talk to each other. However, JavaScript running in web browsers is hampered by security restrictions that prevent code running on one website from accessing data stored or served on another.
Before you start hacking, make sure you understand the limitations posed by cross-domain XMLHttpRequest.
On the bright side, some platforms use JavaScript as their language, but enable the programmer to access other web sites. Examples include:
For those platforms, this library should come in handy.
FAQs
OAuth 1.0a Request Authorization for Node and Browser.
The npm package oauth-1.0a receives a total of 318,708 weekly downloads. As such, oauth-1.0a popularity was classified as popular.
We found that oauth-1.0a demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.