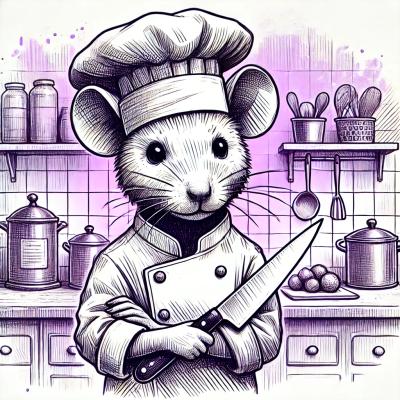
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
occamsrazor-match
Advanced tools
This is an helper library for writing validators.
A validator is a function. When it runs against an object, it returns true or false.
var isFive = function(o) {
return o === 5;
}
isFive(5); // true
isFive(4); // false
Writing complex validators may be a bit verbose. This library helps you to write short yet expressive validators.
This library contains a main helper called "match" and some extra ones.
var match = require('occamsrazor-match');
var has = require('occamsrazor-match/extra/has');
var isInstanceOf = require('occamsrazor-match/extra/isInstanceOf');
var isPrototypeOf = require('occamsrazor-match/extra/isPrototypeOf');
var not = require('occamsrazor-match/extra/not');
var or = require('occamsrazor-match/extra/or');
var and = require('occamsrazor-match/extra/and');
This is the main helper, and can be used to create a lot of different validators.
These ones matches the value in it.
var isFive = match(5);
var isNull = match(null);
var isTrue = match(true);
var isFalse = match(true);
var isHello = match('hello');
For example:
isHello('hello'); // true
isFive(5); // true
Using undefined you create a validator that matches any value:
var isAnything = match(undefined);
isAnything(5);
isAnything('hello');
isAnything({ greeting: 'hello' });
Using a regular expression, the validator will run that, on the value.
var doesMatch = match(/[0-9]+/);
doesMatch('123'); // true
Passing a function, the function itself will be returned:
var isLessThan5 = match(function (n) {
return n < 5;
});
isLessThan5(2); // true
isLessThan5(8); // false
It looks like there is no real reason to do it, this will make more sense in a bit.
"match" can take an array or an object and perform a nested validation:
var isPoint = match({ x : undefined, y: undefined });
isPoint({ x: 1, y: 2 }); // true
isPoint({ x: 1, y: 2, z: 3 }); // true
isPoint({ x: 1, z: 3 }); // false
Object matching ensures the object has all the properties specified. It is not in the scope of the library find out what an object isn't, but rather what it is. Passing values as undefined indicates that I don't really care about their value.
var isPointOnXaxis = match({ x : 0, y: undefined });
isPointOnXaxis({ x: 1, y: 2 }); // false
isPointOnXaxis({ x: 0, y: 2 }); // true
Values will be interpreted as specified before so I can implement recursive validation:
function isNumber(n) { return typeof n === 'number'; }
var isEmployee = match({
name : /[A-Za-z]+/,
job: {
position: undefined,
salary: isNumber
}
});
Arrays are supported as well:
var startsWith123 = match([1, 2, 3]);
startsWith123([1, 2, 3, 4]); // true
startsWith123([2, 3, 4]); // false
This is a shortcut for a very common match:
var isPoint = has(['x', 'y']);
is equivalent to:
var isPoint = match({ x : undefined, y: undefined });
It checks if an object has been built with a specific factory function:
var isPoint = isInstanceOf(Point);
It checks if an object is the prototype of another:
var isPoint = isPrototypeOf(Point.prototype);
Negate the result of a validator. I can take either a function or the argument used for the match function.
var isNotAPoint = not(isPrototypeOf(Point.prototype));
var isNotFive = not(5);
A validator that returns true only if all validators passed as argument return true. Every validator passed is transformed into a function using "match".
function isOdd(n) { return n % 2 !== 0; }
function isInteger(n) { return n % 1 === 0; }
function isSquares(n) { return isInteger(Math.sqrt(n)); }
var oddAndSquared = and([isOdd, isSquared]);
oddAndSquared(4); // false
oddAndSquared(9); // true
A validator that returns true if at least one validator passed as argument returns true. Every validator passed is transformed into a function using "match".
var is5or9 = or(5, 9);
is5or9(5); // true
is5or9(9); // true
is5or9(3); // false
A validator is a simple function that given a value returns true or false. You can build your own and use it together with the other functions:
function isEven(n) {return o % 2 === 0;};
match(isEven);
or([isEven, match(null)]);
match({
numberOfShoes: isEven
});
Note: I have used a named function (not an anonymous function expression or an arrow function). It is important as logging and debugging rely entirely on the function name.
Being able to use functions, you can mix-up and reuse validators:
var isPoint = match({ x : undefined, y: undefined });
var isSquare = match([isPoint, isPoint, isPoint, isPoint]);
var isTriangle = match([isPoint, isPoint, isPoint]);
var containsSquareAndTriangle = {
triangle: isTriangle,
square: isSquare,
extra: or([isSquare, isTriangle])
};
Helpers provide always a descriptive name to the generated functions:
match(true).name === 'isTrue'
match(2).name === 'isNumber:2'
match([1,'test']).name === 'array:[isNumber:1,isString:test]'
has('test1', 'test2').name === 'object:{test1:isAnything,test2:isAnything}'
This can be very helpful for debugging.
This feature can be useful to have some insight about the validation. Like where did it fail, for example. When validating a value you can pass a function, as a second argument. This function is called whenever a validation step succeed or fail:
var validator = match({
user: {
name: /[a-zA-Z]+/,
jobtitle: or(['engineer', 'analyst'])
},
deleted: not(true)
});
validator({
user: {
name: 'Maurizio',
jobtitle: 'engineer'
},
deleted: false
}, function (val) {
// val is an object containing:
{
path: path, // if this validation step is nested in an object or array
name: name, // name of the function
result: result, // true or false
value: o // the value on which the validator ran
}
});
Returning:
{ path: '', name: 'isObject', result: true, value: { user: { name: 'Maurizio', jobtitle: 'engineer' }, deleted: false } },
{ path: 'user', name: 'hasAttribute', result: true, value: { user: { name: 'Maurizio', jobtitle: 'engineer' }, deleted: false } },
{ path: 'user', name: 'isObject', result: true, value: { name: 'Maurizio', jobtitle: 'engineer' } },
{ path: 'user.name', name: 'hasAttribute', result: true, value: { name: 'Maurizio', jobtitle: 'engineer' } },
{ path: 'user.name', name: 'isRegExp:/[a-zA-Z]+/', result: true, value: 'Maurizio' },
{ path: 'user.jobtitle', name: 'hasAttribute', result: true, value: { name: 'Maurizio', jobtitle: 'engineer' } },
{ path: 'user.jobtitle', name: 'isString:engineer', result: true, value: 'engineer' },
{ path: 'deleted', name: 'hasAttribute', result: true, value: { user: { name: 'Maurizio', jobtitle: 'engineer' }, deleted: false } },
{ path: 'deleted', name: 'not(isTrue)', result: true, value: false }]);
FAQs
helper library for writing validators
The npm package occamsrazor-match receives a total of 5 weekly downloads. As such, occamsrazor-match popularity was classified as not popular.
We found that occamsrazor-match demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.