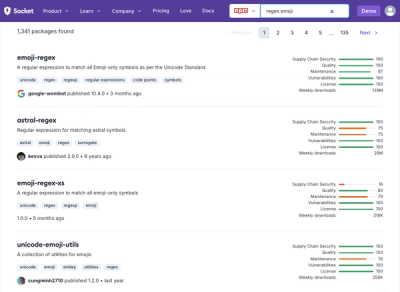
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Octonom brings you TypeScript-based models and collections for any database with proper separation of concerns:
MongoCollection
, CouchCollection
)Let's first define an interface for a person. The interface is required for making the constructor aware of which properties your model accepts.
interface IPerson {
id: string;
name: string;
age: number;
}
Then we can define the actual model:
import { Model } from 'octonom';
export class PersonModel extends Model<IPerson> {
@Model.PropertySchema({type: 'string', default: () => '42'})
public id: string;
@Model.PropertySchema({type: 'string'})
public name: string;
@Model.PropertySchema({type: 'number', integer: true, min: 0})
public age: number;
public makeOlder() {
this.age++;
}
}
Let's create an instance:
// create an instance with initial data
const person = new PersonModel({name: 'Marx', age: 200});
// call a model instance method
person.makeOlder();
console.log(person); // {id: '42', name: 'Marx', age: 201}
// set a model instance property
person.name = 'Rosa';
console.log(person); // {id: '42', name: 'Rosa', age: 201}
Having a local instance of a model is really nice but you probably want to persist it to some database. Collections provide a bridge between raw data objects in a database and the class-based models.
Let's create a collection for people and connect it with a database:
import { MongoCollection } from 'octonom';
// create people collection
const people = new MongoCollection<IPerson, PersonModel>
('people', PersonModel, {modelIdField: 'id'});
// connect with to database
const db = await MongoClient.connect('mongodb://localhost:27017/mydb');
// init collection in database
await people.init(db);
Inserting and retrieving models is straight-forward:
// insert a person
const karl = new PersonModel({id: 'C4p1T4l', name: 'Marx', age: 200});
await people.insertOne(karl);
// retrieve a person
const foundPerson = await people.findById('C4p1T4l');
// foundPerson is again a model instance so we can call its methods
foundPerson.makeOlder();
console.log(foundPerson); // {id: 'C4p1T4l', name: 'Marx', age: 201}
// update a person
await people.update(foundPerson);
FAQs
Object Document Mapper for any database
The npm package octonom receives a total of 0 weekly downloads. As such, octonom popularity was classified as not popular.
We found that octonom demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.