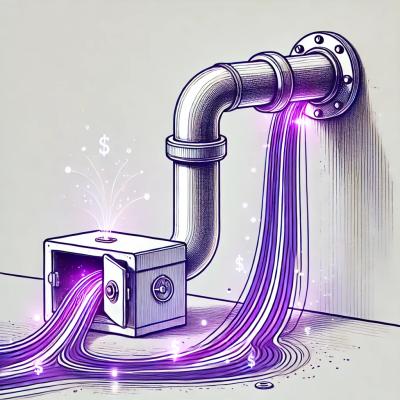
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
The on-headers npm package is used to execute custom logic when headers are about to be written to the response object in a Node.js HTTP server. This can be useful for logging, setting default headers, or performing other actions based on the headers before they are sent to the client.
Execute custom logic before headers are sent
This code sample demonstrates how to use the on-headers package to execute a function right before the headers are sent in an HTTP response. The function `onHeadersListener` is called just before the headers are written to the response.
const onHeaders = require('on-headers');
function onHeadersListener(res) {
console.log('Headers will be sent soon.');
// Perform any custom logic here
}
http.createServer(function (req, res) {
onHeaders(res, onHeadersListener);
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World!');
});
The on-finished package is similar to on-headers in that it allows you to execute code based on HTTP request/response lifecycle events. However, on-finished is used to detect when the HTTP response has finished or when the request has been closed, rather than when headers are about to be sent.
While not directly similar to on-headers, prepend-http is another package that deals with HTTP headers. It is used to prepend `http://` to a URL if it's missing. This package does not provide hooks into the response object's lifecycle.
Helmet is a package that provides a set of middleware functions to set HTTP response headers for security purposes. It differs from on-headers as it focuses on security headers and provides a higher-level API for configuring them, rather than providing a hook for any kind of custom logic before headers are sent.
Execute a listener when a response is about to write headers.
This is a Node.js module available through the
npm registry. Installation is done using the
npm install
command:
$ npm install on-headers
var onHeaders = require('on-headers')
This will add the listener listener
to fire when headers are emitted for res
.
The listener is passed the response
object as it's context (this
). Headers are
considered to be emitted only once, right before they are sent to the client.
When this is called multiple times on the same res
, the listener
s are fired
in the reverse order they were added.
var http = require('http')
var onHeaders = require('on-headers')
http
.createServer(onRequest)
.listen(3000)
function addPoweredBy () {
// set if not set by end of request
if (!this.getHeader('X-Powered-By')) {
this.setHeader('X-Powered-By', 'Node.js')
}
}
function onRequest (req, res) {
onHeaders(res, addPoweredBy)
res.setHeader('Content-Type', 'text/plain')
res.end('hello!')
}
$ npm test
FAQs
Execute a listener when a response is about to write headers
The npm package on-headers receives a total of 18,181,974 weekly downloads. As such, on-headers popularity was classified as popular.
We found that on-headers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.