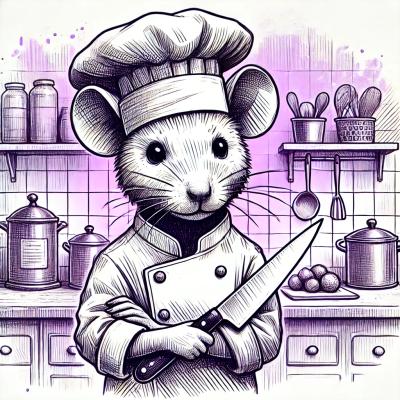
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
OneJS is a command-line utility for converting CommonJS packages to single, stand-alone JavaScript files that can be run on web browsers.
$ one > browser.js
$ npm install -g one
The quickest way of giving OneJS a try is to run "onejs build package.json" command in a project folder. It'll walk through all the directories, find JavaScript files in the "lib" folder (if exists, and by considering .npmignore), and produce an output that can be run by any web browser and NodeJS, as well.
Bundle files produced by OneJS consist of a CommonJS implementation and the throughout package tree wrapped by OneJS' templates. And the produced output will be unobtrusive. Which means, your project will not conflict with any other JavaScript on the page that is embedded.
Once you produce the output, as you expect, it needs to be included by an HTML page, like the below example;
$ one build hello-world/package.json output.js
$ cat > index.html
<html>
<script src="output.js"></script>
<script>helloWorld();</script>
</html>
You may notice a function named helloWorld
was called. That's what starts running your program by requiring the main
module of the project.
Besides of that helloWorld
refers to the main module, it also exports some utilities that can be useful. See following for an example;
> helloWorld.require('./module');
[object ./module.js]
> helloWorld.require('dependency');
[object node_modules/dependency]
In addition to command-line API, there are two more ways to configure build options. You can have the configuration in a package.json manfest;
{
"name": "hello-world",
"version": "1.0.0",
"directories": {
"lib": "lib"
},
"web": {
"save": "bundle.js",
"alias": {
"crypto": "crypto-browserify"
},
"tie": {
"jquery": "window.jQuery"
}
}
}
Or, you can write your own build script using OneJS' chaining API:
// build.js
var one = require('../../../lib');
one('./package.json')
.alias('crypto', 'crypto-browserify')
.tie('pi', 'Math.PI')
.tie('json', 'JSON')
.exclude('underscore')
.filter(/^build\.js$/)
.filter(/^bundle\.js$/)
.save('bundle.js');
Specified dependencies (including their subdependencies) can be splitted to different files via package.json
manifest.
{
"name": "hello-world",
"version": "1.0.0",
"dependencies": {
"foo": "*",
"bar": "*"
},
"web": {
"save": {
"hello-world": "hello-world.js",
"bar": {
"to": "bar.js",
"url: "/js/bar.js"
}
}
}
}
OneJS will be outputting an async require implementation to let you load splitted packages;
// hello-world/index.js
var foo = require('foo');
require.async('bar', function(bar)){ // loads "/js/bar.js"
console.log('dependencies are loaded!');
console.log(bar);
// => [object bar]
});
OneJS doesn't have a watching utility since a built-in one becomes useless when you have other build steps.
Recommended way is to create a Makefile, and use the good tools such as visionmedia/watch to , isaacs/node-supervisor build your project when there is a change. Following Makefile example watches files under /lib directory.
SRC = $(wildcard lib/*/*.js)
build: $(SRC)
@one build package.json bundle.js
Below command will be updating bundle.js
when lib/
has a change.
$ watch make
Registers a new name for specified package. It can be configured via command-line, package.json and NodeJS APIs.
$ one build package.json output.js --alias request:superagent,crypto:crypto-browserify
package.json
{
"name": "hello-world",
"web": {
"alias": {
"crypto": "crypto-browserify"
}
}
}
NodeJS
one('./package.json')
.alias('crypto', 'crypto-browserify')
.save('foo.js');
OneJS doesn't stop you from accessing globals. However, you may want to use require
for accessing some global variables,
such as jQuery, for some purposes like keeping your source-code self documented.
OneJS may tie some package names to global variables if demanded. And it can be configured via command-line, package.json and NodeJS APIs.
$ one build package.json output.js --tie jquery:jQuery,google:goog
package.json
{
"name": "hello-world",
"web": {
"tie": {
"jquery": "jQuery",
"google": "goog"
}
}
}
NodeJS
one('./package.json')
.tie('jquery', 'jQuery')
.tie('google', 'goog')
.save('foo.js');
Excludes specified packages from your bundle.
$ one build package.json output.js --excude underscore
package.json
{
"name": "hello-world",
"web": {
"exclude": ["underscore"]
}
}
NodeJS
one('./package.json')
.exclude('underscore')
.save('foo.js');
OneJS reads .npmignore to ignore anything not wanted to have in the bundle. In addition to .npmignore, you may also define your own Regex filters to ignore files. This config is only provided for only NodeJS scripts.
one('./package.json')
.filter(/^build\.js$/)
.filter(/^bundle\.js$/)
.save('bundle.js');
You may add some dependencies not defined in your package.json;
one('./package.json')
.dependency('request', '2.x')
.dependency('async', '2.x')
.save(function(error, bundle){
bundle
// => the source code
})
one('./package.json')
.devDependencies()
.save('bundle.js')
OneJS defines the global variable that wraps the bundle by default. For example, a project named "hello-world" lies under "helloWorld" object on the browsers.
To customize it, define "name" field on your package.json;
{
"name": "hello-world",
"web": {
"name": "HelloWorld"
}
}
OneJS lets you pick any of the following ways to configure your build.
{
"name": "hello-world",
"version": "1.0.0",
"directories": {
"lib": "lib"
},
"web": {
"name":"HelloWorld",
"save": "bundle.js",
"alias": {
"crypto": "crypto-browserify"
},
"tie": {
"jquery": "window.jQuery"
}
}
}
usage: onejs [action] [manifest] [options]
Transforms NodeJS packages into single, stand-alone JavaScript files that can be run at other platforms. See the documentation at http://github.com/azer/onejs for more information.
actions:
build <manifest> <target> Generate a stand-alone JavaScript file from specified package. Write output to <target> if given any.
server <manifest> <port> <host> Publish generated JavaScript file on web. Uses 127.0.0.1:1338 by default.
options:
--debug Enable SourceURLs.
--alias <alias>:<package name> Register an alias name for given package. e.g: request:superagent,crypto:crypto-browserify
--tie <package name>:<global object> Create package links to specified global variables. e.g; --tie dom:window.document,jquery:jQuery
--exclude <package name> Do not contain specified dependencies. e.g: --exclude underscore,request
--plain Builds the package within a minimalistic template for the packages with single module and no dependencies.
--quiet Make console output less verbose.
--verbose Tell what's going on by being verbose.
--version Show version and exit.
--help Show help.
// build.js
var one = require('../../../lib');
one('./package.json')
.alias('crypto', 'crypto-browserify')
.tie('pi', 'Math.PI')
.tie('json', 'JSON')
.include('optional-module.js')
.dependency('optional-dependency', '2.x')
.exclude('underscore')
.filter(/^build\.js$/)
.filter(/^bundle\.js$/)
.devDependencies()
.name('helloKitty')
.save('bundle.js'); // or .save(function(error, bundle){ })
// Following examples are out of date. Will be updated soon.
node_modules/
properly.onejs build package.json --verbose
projectName.map
object if it contains the missing dependencyRun npm test
for running all test modules. And run make test module=?
for specific test modules;
> make test module=build
FAQs
One is a new React Framework that makes Vite serve both native and web.
The npm package one receives a total of 1,299 weekly downloads. As such, one popularity was classified as popular.
We found that one demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.