Opensea Scraper
Scraping NFT floor prices from opensea, because the Opensea API returns inaccurate floor prices. With this utility you get the actual floor price, that is the lowest offer currently availible.
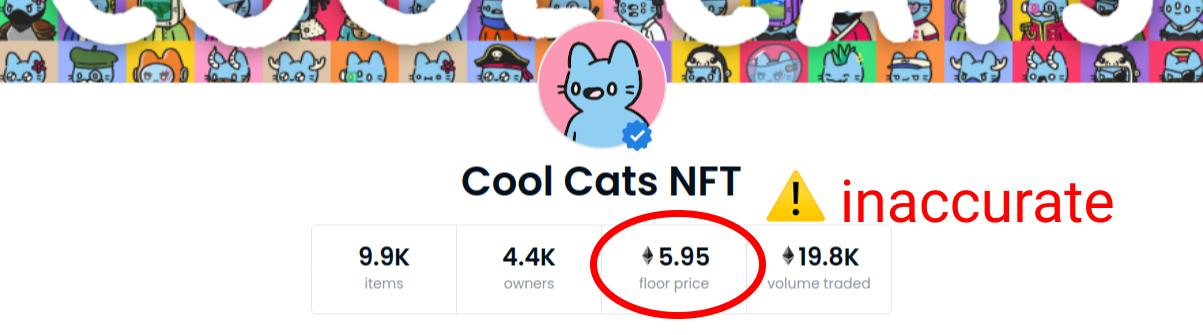
Install
npm install opensea-scraper
Usage
const OpenseaScraper = require("opensea-scraper");
const slug = "cool-cats-nft";
const floorPrice = await OpenseaScraper.floorPrice(slug);
const basicInfo = await OpenseaScraper.basicInfo(slug);
**slug**
is the human readable identifier that opensea uses to identify a collection. It can be extracted from the URL: https://opensea.io/collection/{slug}

Demo
npm run demo
Script to fetch Floor Price from API
⚠ Important Note: floor prices fetched with this method are not accurate (not in real time).
const axios = require("axios");
async function getFloorPrice(slug) {
try {
const url = `https://api.opensea.io/collection/${slug}`;
const response = await axios.get(url);
return response.data.collection.stats.floor_price;
} catch(err) {
console.log(err);
return undefined;
}
}
const result = await getFloorPrice("lostpoets");
const result = await getFloorPrice("treeverse");
const result = await getFloorPrice("cool-cats-nft");
Contribute
Open PR or issue if you would like to have more features added.