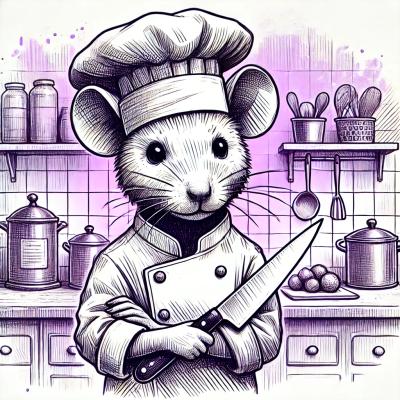
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The optics-ts package provides a powerful and type-safe way to work with immutable data structures in TypeScript. It allows you to create and compose lenses, prisms, and other optics to focus on specific parts of your data structures, making it easier to read, update, and transform data in a functional programming style.
Lenses
Lenses allow you to focus on a specific part of a data structure. In this example, we create a lens to focus on the city within the user's address and demonstrate how to get and set the city value.
const { lens } = require('optics-ts');
const user = { name: 'Alice', address: { city: 'Wonderland' } };
const addressLens = lens(user => user.address, (user, address) => ({ ...user, address }));
const cityLens = addressLens.compose(lens(address => address.city, (address, city) => ({ ...address, city })));
const city = cityLens.get(user); // 'Wonderland'
const updatedUser = cityLens.set(user, 'New Wonderland'); // { name: 'Alice', address: { city: 'New Wonderland' } }
Prisms
Prisms are used to focus on a part of a data structure that may or may not be present. In this example, we create a prism to focus on the value inside an option type and demonstrate how to get and set the value.
const { prism } = require('optics-ts');
const some = value => ({ type: 'Some', value });
const none = { type: 'None' };
const optionPrism = prism(
option => (option.type === 'Some' ? option.value : undefined),
value => some(value)
);
const option = some(42);
const value = optionPrism.getOption(option); // 42
const updatedOption = optionPrism.set(option, 100); // { type: 'Some', value: 100 }
Traversal
Traversal allows you to focus on multiple parts of a data structure. In this example, we create a traversal to focus on even numbers in an array and demonstrate how to get all even numbers and modify them.
const { traversal } = require('optics-ts');
const numbers = [1, 2, 3, 4, 5];
const evenTraversal = traversal(
numbers => numbers.filter(n => n % 2 === 0),
(numbers, evens) => numbers.map(n => (n % 2 === 0 ? evens.shift() : n))
);
const evens = evenTraversal.getAll(numbers); // [2, 4]
const updatedNumbers = evenTraversal.modify(numbers, n => n * 2); // [1, 4, 3, 8, 5]
monocle-ts is a library for functional optics in TypeScript. It provides similar functionality to optics-ts, including lenses, prisms, and traversals. monocle-ts is well-integrated with the fp-ts library, making it a good choice for projects that already use fp-ts.
Ramda is a functional programming library for JavaScript that includes support for lenses. While it is not TypeScript-specific, it provides a wide range of functional utilities, including lenses, for working with immutable data structures.
partial.lenses is a library for working with immutable data structures using lenses in JavaScript. It is highly optimized and provides a rich set of features for creating and composing lenses. It is not TypeScript-specific but can be used with TypeScript.
optics-ts
provides type-safe, ergonomic, polymorphic optics for TypeScript:
any
,
ever.➡ Documentation ⬅
optics-ts
supports lenses, prisms, traversals, removing items from containers,
and much more!
Since optics-ts v2.2.0, there are two syntaxes for defining optics: method chaining (the default) and standalone optics (experimental). See the docs for more info!
Installation:
npm install optics-ts
or
yarn add optics-ts
Here's a simple example demonstrating how lenses can be used to drill into a nested data structure:
import * as O from 'optics-ts'
type Book = {
title: string
isbn: string
author: {
name: string
}
}
// Create a lens that focuses on author.name
const optic = O.optic_<Book>().prop('author').prop('name')
// This is the input data
const input: Book = {
title: "The Hitchhiker's Guide to the Galaxy",
isbn: '978-0345391803',
author: {
name: 'Douglas Adams',
},
}
// Read through the optic
O.get(optic)(input)
// "Douglas Adams"
// Write through the optic
O.set(optic)('Arthur Dent')(input)
// {
// title: "The Hitchhiker’s Guide to the Galaxy"
// isbn: "978-0345391803",
// author: {
// name: "Arthur Dent"
// }
// }
// Update the existing value through the optic, while also changing the data type
O.modify(optic)((str) => str.length + 29)(input)
// {
// title: "The Hitchhiker’s Guide to the Galaxy"
// isbn: "978-0345391803",
// author: {
// name: 42
// }
// }
Another example that converts all words longer than 5 characters to upper case:
import * as O from 'optics-ts/standalone'
const optic = O.optic<string>().words().when(s => s.length >= 5)
const input = 'This is a string with some shorter and some longer words'
O.modify(optic)((s) => s.toUpperCase()(input)
// "This is a STRING with some SHORTER and some LONGER WORDS"
See the documentation for a tutorial and a detailed reference of all supported optics.
Run yarn
to install dependencies.
Run yarn test
.
For compiling and running the tests when files change, run these commands in separate terminals:
yarn build:test --watch
yarn jest dist-test/ --watchAll
You need Python 3 to build the docs.
python3 -m venv venv
./venv/bin/pip install mkdocs-material
Run a live reloading server for the documentation:
./venv/bin/mkdocs serve
Open http://localhost:8000/ in the browser.
$ yarn version --new-version <major|minor|patch>
$ yarn publish
$ git push origin main --tags
Open https://github.com/akheron/optics-ts/releases, edit the draft release, select the newest version tag, adjust the description as needed.
FAQs
Type-safe, ergonomic, polymorphic optics for TypeScript
We found that optics-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.