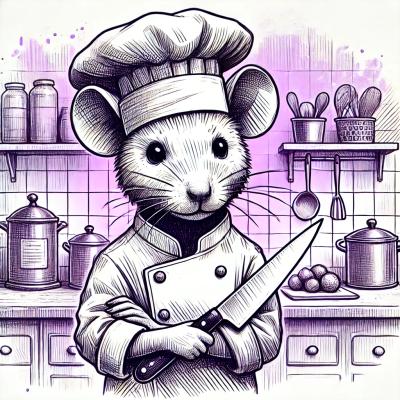
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
p-each-series
Advanced tools
The p-each-series package is a utility that allows you to iterate over a list of items and apply an asynchronous function to each item in series, meaning one after the other. It returns a Promise that resolves when all the iterations are completed. This is particularly useful when you need to perform async operations in a specific order.
Iterating over an array in series
This code sample demonstrates how to use p-each-series to iterate over an array of items and log each item to the console one by one. After all items have been logged, it prints 'Done:' followed by the original array.
const pEachSeries = require('p-each-series');
const logItem = async item => {
console.log(item);
return item;
};
(async () => {
const result = await pEachSeries(['a', 'b', 'c'], logItem);
console.log('Done:', result);
})();
Handling asynchronous operations in series
This code sample shows how p-each-series can be used to perform asynchronous operations on each item in an array, with each operation being delayed by 1 second. It logs a message after each item is processed and finally logs that all items have been processed.
const pEachSeries = require('p-each-series');
const asyncOperation = item => new Promise(resolve => {
setTimeout(() => {
console.log(`Processed ${item}`);
resolve(item);
}, 1000);
});
(async () => {
const result = await pEachSeries(['item1', 'item2', 'item3'], asyncOperation);
console.log('All items have been processed:', result);
})();
The 'async' package provides a wide range of functions for working with asynchronous code. Its 'eachSeries' function is similar to p-each-series, but 'async' offers many other patterns for control flow as well, making it more versatile but also larger in size.
Bluebird is a fully-featured promise library that includes utilities for serial and parallel flow control. Its 'mapSeries' function can be used similarly to p-each-series for serial iteration, but Bluebird also provides a plethora of other features for promise manipulation and optimization.
p-series is another package that runs promise-returning & async functions in series. It is similar to p-each-series but is designed to run an array of functions rather than mapping over an array of values.
Iterate over promises serially
Useful as a side-effect iterator. Prefer p-map
if you don't need side-effects, as it's concurrent.
$ npm install p-each-series
import pEachSeries from 'p-each-series';
const keywords = [
getTopKeyword(), //=> Promise
'rainbow',
'pony'
];
const iterator = async element => saveToDiskPromise(element);
console.log(await pEachSeries(keywords, iterator));
//=> ['unicorn', 'rainbow', 'pony']
Returns a Promise
that is fulfilled when all promises in input
and ones returned from iterator
are fulfilled, or rejects if any of the promises reject. The fulfillment value is the original input
.
Type: Iterable<Promise | unknown>
Iterated over serially in the iterator
function.
Type: Function
Return value is ignored unless it's Promise
, then it's awaited before continuing with the next iteration.
Stop iterating through items by returning pEachSeries.stop
from the iterator function.
import pEachSeries from 'p-each-series';
// Logs `a` and `b`.
const result = await pEachSeries(['a', 'b', 'c'], value => {
console.log(value);
if (value === 'b') {
return pEachSeries.stop;
}
});
console.log(result);
//=> ['a', 'b', 'c']
FAQs
Iterate over promises serially
The npm package p-each-series receives a total of 3,572,801 weekly downloads. As such, p-each-series popularity was classified as popular.
We found that p-each-series demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.