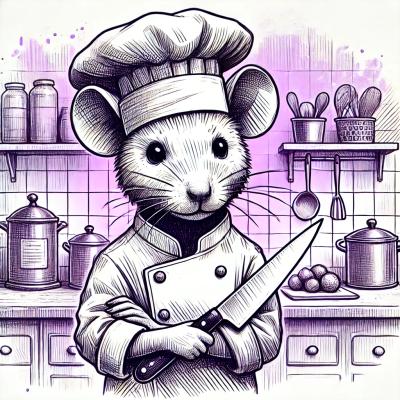
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
p-throttle
Advanced tools
The p-throttle npm package is used to throttle the execution of asynchronous functions. It allows you to limit the number of times a function can be called over a specified period, which is useful for rate-limiting API requests or controlling the flow of operations in a system.
Basic Throttling
This feature allows you to throttle the execution of a function. In this example, the function can only be called twice per second.
const pThrottle = require('p-throttle');
const throttle = pThrottle({ limit: 2, interval: 1000 });
const throttled = throttle(async (index) => {
console.log(index);
});
(async () => {
for (let i = 0; i < 10; i++) {
throttled(i);
}
})();
Throttling with Promises
This feature demonstrates throttling with asynchronous functions that return promises. The function is throttled to execute once every two seconds.
const pThrottle = require('p-throttle');
const throttle = pThrottle({ limit: 1, interval: 2000 });
const throttled = throttle(async (index) => {
return new Promise((resolve) => {
setTimeout(() => {
console.log(index);
resolve();
}, 1000);
});
});
(async () => {
for (let i = 0; i < 5; i++) {
await throttled(i);
}
})();
Bottleneck is a powerful rate limiter that can be used to control the rate of asynchronous operations. It offers more advanced features like clustering and priority queues, making it more suitable for complex scenarios compared to p-throttle.
Limiter is another rate-limiting library that provides a simple way to limit the number of operations over a given time period. It is similar to p-throttle but offers a more straightforward API for basic use cases.
Rate-limiter-flexible is a highly flexible rate-limiting library that supports various backends like Redis and MongoDB. It provides more configuration options and is suitable for distributed systems, offering more flexibility than p-throttle.
Throttle promise-returning & async functions
It also works with normal functions.
It rate-limits function calls without discarding them, making it ideal for external API interactions where avoiding call loss is crucial.
npm install p-throttle
Here, the throttled function is only called twice a second:
import pThrottle from 'p-throttle';
const now = Date.now();
const throttle = pThrottle({
limit: 2,
interval: 1000
});
const throttled = throttle(async index => {
const secDiff = ((Date.now() - now) / 1000).toFixed();
return `${index}: ${secDiff}s`;
});
for (let index = 1; index <= 6; index++) {
(async () => {
console.log(await throttled(index));
})();
}
//=> 1: 0s
//=> 2: 0s
//=> 3: 1s
//=> 4: 1s
//=> 5: 2s
//=> 6: 2s
Returns a throttle function.
Type: object
Both the limit
and interval
options must be specified.
Type: number
The maximum number of calls within an interval
.
Type: number
The timespan for limit
in milliseconds.
Type: boolean
Default: false
Use a strict, more resource intensive, throttling algorithm. The default algorithm uses a windowed approach that will work correctly in most cases, limiting the total number of calls at the specified limit per interval window. The strict algorithm throttles each call individually, ensuring the limit is not exceeded for any interval.
Type: AbortSignal
Abort pending executions. When aborted, all unresolved promises are rejected with signal.reason
.
import pThrottle from 'p-throttle';
const controller = new AbortController();
const throttle = pThrottle({
limit: 2,
interval: 1000,
signal: controller.signal
});
const throttled = throttle(() => {
console.log('Executing...');
});
await throttled();
await throttled();
controller.abort('aborted')
await throttled();
//=> Executing...
//=> Executing...
//=> Promise rejected with reason `aborted`
Type: Function
Get notified when function calls are delayed due to exceeding the limit
of allowed calls within the given interval
. The delayed call arguments are passed to the onDelay
callback.
Can be useful for monitoring the throttling efficiency.
In the following example, the third call gets delayed and triggers the onDelay
callback:
import pThrottle from 'p-throttle';
const throttle = pThrottle({
limit: 2,
interval: 1000,
onDelay: (a, b) => {
console.log(`Reached interval limit, call is delayed for ${a} ${b}`);
},
});
const throttled = throttle((a, b) => {
console.log(`Executing with ${a} ${b}...`);
});
await throttled(1, 2);
await throttled(3, 4);
await throttled(5, 6);
//=> Executing with 1 2...
//=> Executing with 3 4...
//=> Reached interval limit, call is delayed for 5 6
//=> Executing with 5 6...
Returns a throttled version of function_
.
Type: Function
A promise-returning/async function or a normal function.
Type: boolean
Default: true
Whether future function calls should be throttled and count towards throttling thresholds.
Type: number
The number of queued items waiting to be executed.
FAQs
Throttle promise-returning & async functions
The npm package p-throttle receives a total of 1,008,501 weekly downloads. As such, p-throttle popularity was classified as popular.
We found that p-throttle demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.