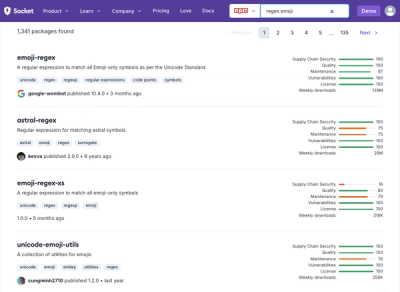
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
pawe
An automatic web progress bar. Inspired by pace
.
If you're using a framework like React, that comes with a build system.
Run this command in your terminal:
npm i pawe
Then, add this in your layout (e.g. layout.tsx
, +layout.svelte
, etc.)
import 'pawe';
If you want to use
pawe
directly in the browser.
Include this in the <head>
of your HTML:
<script
type="module"
src="https://cdn.jsdelivr.net/npm/pawe"
></script>
This is the default mode. This means pawe
will automatically start monitoring the page load progress & expose the values as HTML attributes & CSS custom properties.
A basic progress bar.
<style>
/* 1. using CSS custom properties for values */
#progress {
/* progress bar */
width: var(--pawe-bar-percent, 0%);
background: hsl(0 0% 0%);
position: fixed;
height: 2px;
top: 0;
left: 0;
transition:
width 1s ease-out,
opacity 1s;
/* progress text (i.e. '100%') */
&::after {
content: var(--pawe-bar-percent-string) '%';
}
}
/* 2. using HTML attributes for state changes */
:root[data-pawe='idle'] #progress {
opacity: 0;
}
</style>
<div id="progress"></div>
Here are all the exposed values in HTML/CSS that you can use:
Attribute | Element | Type | Description |
---|---|---|---|
data-pawe | :root (<html> ) | idle | loading | The current state of pawe . |
Property | Range | Description |
---|---|---|
--pawe-bar | 0 to 1 | The augmented progress of the page load that approximates the loading in between down time of collected data points. Users generally prefer this as it provides feedback even if their connections are spotty, causing data to come down in bursts. |
--pawe-bar-percent | 0% to 100% | '', in percent. |
--pawe-bar-percent-int | 0 to 100 | '', floored, without unit. |
--pawe-bar-percent-string | '0' to '100' | '', in string type, for use with the content property. |
--pawe-progress | 0 to 1 | The objective progress of the page load solely based on the collected data points. |
--pawe-progress-percent | 0% to 100% | '', in percent. |
--pawe-progress-percent-int | 0 to 100 | '', floored, without unit. |
--pawe-progress-percent-string | '0' to '100' | '', in string type, for use with the content property. |
You can also use the JavaScript API to get the progress values programmatically.
For the full list of available exports, see the index.ts
file.
Better docs are coming soon™!
import { bar } from 'pawe';
const unsubscribe = bar.subscribe(($bar) => {
// this is equivalent to `--pawe-bar`
console.log(`bar: ${$bar}`);
});
You can also use pawe
in manual mode by importing from pawe/api
. This means you are in charge of starting the monitoring & scaffolding any other load signals you want to track.
// note the different import!
// * `pawe` - automatic mode
// monitoring is started automatically
// * `pawe/api` - manual mode
// only the api is exposed & no side effects are ran
import { monitorDOM, createLoad, progress } from 'pawe/api';
// log the progress values as we add loads
const unsubscribe = progress.subscribe(($progress) => {
console.log(`progress: ${$progress}`);
});
// add monitoring of the DOM (skipping the rest)
monitorDOM();
// add your own load signal to the calculated progress
const load = createLoad();
console.log('starting load...');
console.log('loaded: 0%');
// simulate loading
load.set(0.5);
console.log('loaded: 50%');
// mark as finished after 1 second
setTimeout(() => {
load.finish();
// or
// `load.set(1)`
}, 1000);
// the load acts as a promise that will resolve when it hits `1`
await load;
console.log('loaded: 100%');
// note the different import!
// * `pawe` - automatic mode
// monitoring is started automatically
// * `pawe/api` - manual mode
// only the api is exposed & no side effects are ran
import { createPool, createLoad, createProgress, createBar } from 'pawe/api';
// where all the loads are stored
const pool = createPool();
// create a load signal
const load = createLoad(pool);
// ^^^^
// note that `pool` is passed as the first argument!
// this is so that the load signal is added to our pool
// instead of the global one
// create a progress signal
const progress = createProgress(pool);
// create a progress bar (for UI)
const bar = createBar(progress);
// use the progress values
const unsubscribeProgress = progress.subscribe(($progress) => {
console.log(`progress: ${$progress}`);
});
const unsubscribeBar = bar.subscribe(($bar) => {
console.log(`bar: ${$bar}`);
});
If you want to bypass AJAX requests from being tracked by pawe
, you can pass in the non-standard pawe
property. It will be removed from the final config/instance before being sent.
fetch
const resp = await fetch('https://example.com', {
pawe: 'bypass', // add this to bypass tracking
});
XMLHttpRequest
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com');
xhr.pawe = 'bypass'; // add this to bypass tracking
xhr.send();
Include the following in your tsconfig.json
to get type hints for the above mentioned pawe
property.
{
"compilerOptions": {
"types": ["pawe/client"]
}
}
DOM
- Track load events from DOM elements that are statically & dynamically added to the page
document.readyState
until complete
<img>
load (both eager & lazy)<video>
load (when it starts loading until it's ready to play)<audio>
load (when it starts loading until it's ready to play)<iframe>
load<object>
load<embed>
loadfetch
- Track data stream from fetch
requestsXMLHttpRequest
- Track progress
events from XMLHttpRequest
sMIT
FAQs
An automatic web progress bar.
The npm package pawe receives a total of 180 weekly downloads. As such, pawe popularity was classified as not popular.
We found that pawe demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.