polly-js
Transient exception handling for JavaScript made easy.

Polly-js is a library to help developers recover from transient errors using policies like retry or wait and retry.
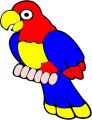
Usage
Try to load the Google home page and retry twice if it fails.
polly()
.retry(2)
.executeForPromise(function () {
return requestPromise('http://www.google.com');
})
.then(function(result) {
console.log(result)
}, function(err) {
console.error('Failed trying three times', err)
});
Try to read a file from disk and retry twice if this fails.
polly()
.retry(2)
.executeForNode(function (cb) {
fs.readFile(path.join(__dirname, './hello.txt'), cb);
}, function (err, data) {
if (err) {
console.error('Failed trying three times', err)
} else {
console.log(data)
}
});
Only retry 'no such file or directory' errors. Wait 100 ms before retrying.
polly()
.handle(function(err) {
return err.code === 'ENOENT';
})
.waitAndRetry()
.executeForNode(function (cb) {
fs.readFile(path.join(__dirname, './not-there.txt'), cb);
}, function (err, data) {
if (err) {
console.error('Failed trying twice with a 100ms delay', err)
} else {
console.log(data)
}
});
Acknowledgements
The library is based on the Polly NuGet package by Michael Wolfenden