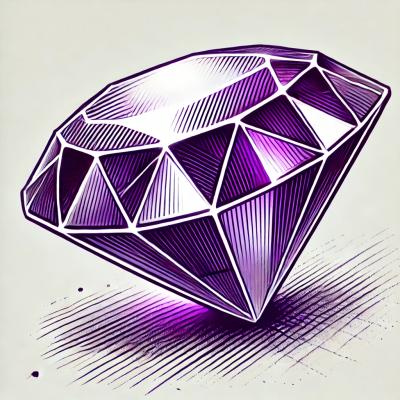
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
The 'progress' npm package is used to create a flexible text progress bar in the console. It is useful for tracking the progress of an operation in command-line applications.
Basic Progress Bar
This code sample demonstrates how to create a simple progress bar that updates every 100 milliseconds until it's complete.
const ProgressBar = require('progress');
let bar = new ProgressBar(':bar', { total: 10 });
let timer = setInterval(() => {
bar.tick();
if (bar.complete) {
console.log('\nComplete!');
clearInterval(timer);
}
}, 100);
Custom Tokens
This code sample shows how to use custom tokens within the progress bar format to display additional information.
const ProgressBar = require('progress');
let bar = new ProgressBar(':current/:total (:percent) :bar :custom', { total: 20, width: 30 });
bar.tick({ custom: 'Token' });
Increment with Step
This code sample illustrates how to increment the progress bar by a step greater than one.
const ProgressBar = require('progress');
let bar = new ProgressBar(':bar', { total: 10 });
bar.tick(2);
cli-progress is a full-featured progress bar for Node.js command line applications. It offers more customization options than 'progress', such as multiple bars, custom bar characters, and payload handling.
nanobar is a very lightweight progress bar without jQuery. While it is not directly comparable to 'progress' since it's for browser use, it serves a similar purpose in providing visual feedback for progress in a minimalistic way.
node-progress-bars is another alternative to 'progress' that allows for multiple simultaneous progress bars in the terminal. It provides a different API and additional features like color support.
Flexible ascii progress bar.
$ npm install progress
First we create a ProgressBar
, giving it a format string
as well as the total
, telling the progress bar when it will
be considered complete. After that all we need to do is tick()
appropriately.
var ProgressBar = require('progress');
var bar = new ProgressBar(':bar', { total: 10 });
var timer = setInterval(function () {
bar.tick();
if (bar.complete) {
console.log('\ncomplete\n');
clearInterval(timer);
}
}, 100);
These are keys in the options object you can pass to the progress bar along with
total
as seen in the example above.
curr
current completed indextotal
total number of ticks to completewidth
the displayed width of the progress bar defaulting to totalstream
the output stream defaulting to stderrhead
head character defaulting to complete charactercomplete
completion character defaulting to "="incomplete
incomplete character defaulting to "-"renderThrottle
minimum time between updates in milliseconds defaulting to 16clear
option to clear the bar on completion defaulting to falsecallback
optional function to call when the progress bar completesThese are tokens you can use in the format of your progress bar.
:bar
the progress bar itself:current
current tick number:total
total ticks:elapsed
time elapsed in seconds:percent
completion percentage:eta
estimated completion time in seconds:rate
rate of ticks per secondYou can define custom tokens by adding a {'name': value}
object parameter to your method (tick()
, update()
, etc.) calls.
var bar = new ProgressBar(':current: :token1 :token2', { total: 3 })
bar.tick({
'token1': "Hello",
'token2': "World!\n"
})
bar.tick(2, {
'token1': "Goodbye",
'token2': "World!"
})
The above example would result in the output below.
1: Hello World!
3: Goodbye World!
In our download example each tick has a variable influence, so we pass the chunk length which adjusts the progress bar appropriately relative to the total length.
var ProgressBar = require('progress');
var https = require('https');
var req = https.request({
host: 'download.github.com',
port: 443,
path: '/visionmedia-node-jscoverage-0d4608a.zip'
});
req.on('response', function(res){
var len = parseInt(res.headers['content-length'], 10);
console.log();
var bar = new ProgressBar(' downloading [:bar] :rate/bps :percent :etas', {
complete: '=',
incomplete: ' ',
width: 20,
total: len
});
res.on('data', function (chunk) {
bar.tick(chunk.length);
});
res.on('end', function () {
console.log('\n');
});
});
req.end();
The above example result in a progress bar like the one below.
downloading [===== ] 39/bps 29% 3.7s
To display a message during progress bar execution, use interrupt()
var ProgressBar = require('progress');
var bar = new ProgressBar(':bar :current/:total', { total: 10 });
var timer = setInterval(function () {
bar.tick();
if (bar.complete) {
clearInterval(timer);
} else if (bar.curr === 5) {
bar.interrupt('this message appears above the progress bar\ncurrent progress is ' + bar.curr + '/' + bar.total);
}
}, 1000);
You can see more examples in the examples
folder.
MIT
FAQs
Flexible ascii progress bar
The npm package progress receives a total of 7,556,655 weekly downloads. As such, progress popularity was classified as popular.
We found that progress demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.